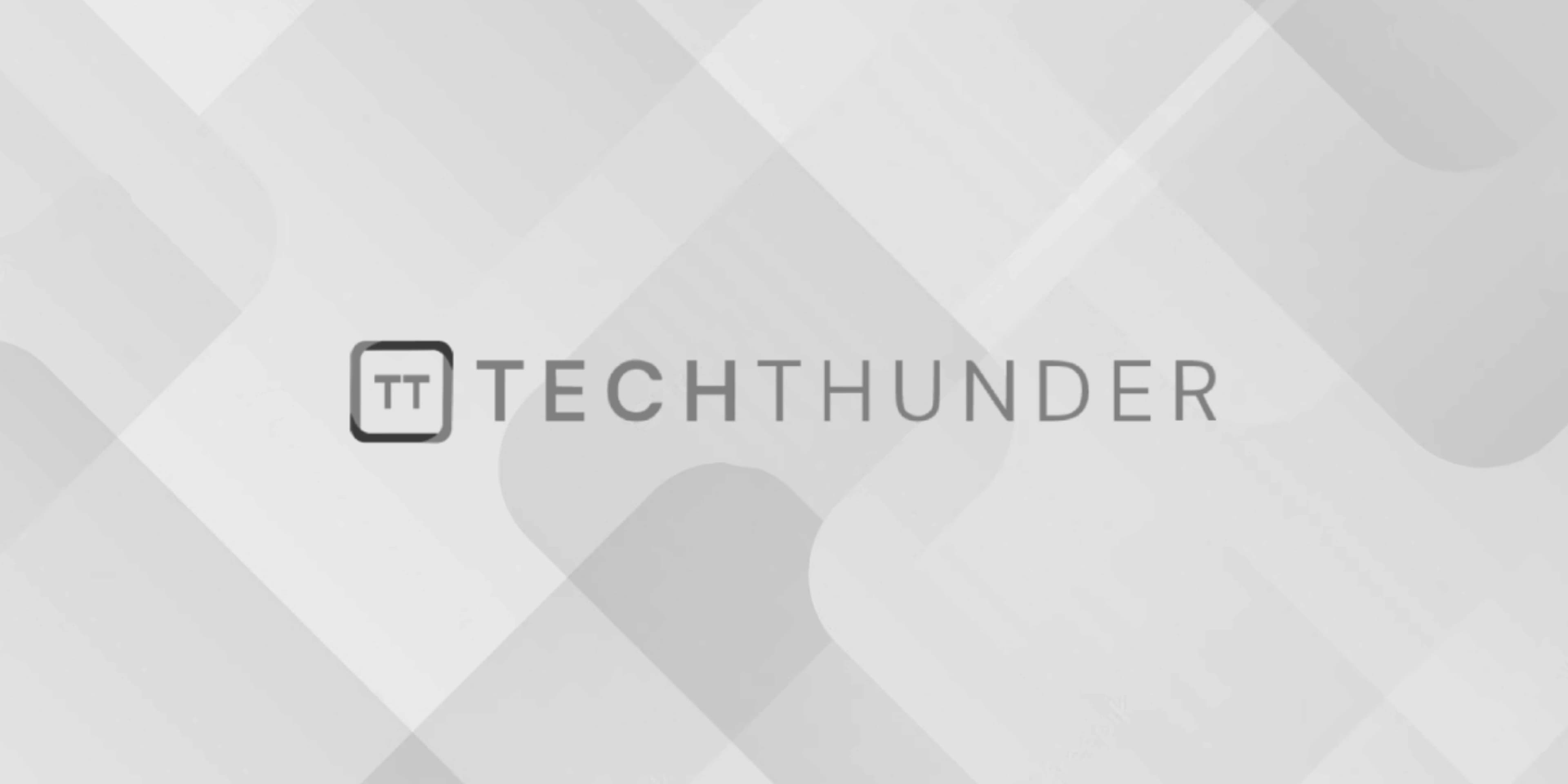
C++ Variable
The C++ variable is a named storage location in memory used to store data that can be accessed and manipulated by your program. Variables have a data type that defines the type of data they can hold, such as integers, floating-point numbers, characters, and more. Here’s how you declare and use variables in C++:
Variable Declaration:
To declare a variable, you specify its data type followed by the variable name:
data_type variable_name;
For example:
int age; // Declares an integer variable named 'age'.
double salary; // Declares a double-precision floating-point variable named 'salary'.
char grade; // Declares a character variable named 'grade'.
Variable Initialization:
You can also initialize a variable at the time of declaration by assigning it an initial value:
data_type variable_name = initial_value;
For example:
int age = 30; // Initializes 'age' to 30.
double salary = 50000; // Initializes 'salary' to 50000.0.
char grade = 'A'; // Initializes 'grade' to the character 'A'.
Variable Assignment:
After declaring and initializing a variable, you can assign new values to it during the execution of your program:
variable_name = new_value;
For example:
age = 35; // Assigns a new value (35) to 'age'.
salary = 55000.5; // Assigns a new value (55000.5) to 'salary'.
grade = 'B'; // Assigns a new value ('B') to 'grade'.
Using Variables:
You can use variables in expressions, assignments, and other operations within your program. For example:
int x = 5;
int y = 10;
int sum = x + y; // Adds the values of 'x' and 'y' and stores the result in 'sum'.
Variable Names:
Variable names must adhere to certain rules and conventions:
- Variable names are case-sensitive (e.g.,
myVar
andmyvar
are different). - Variable names must start with a letter or underscore (
_
), followed by letters, digits, or underscores. - Variable names should be meaningful and describe the purpose of the variable (e.g.,
counter
instead ofc
).
Here’s an example that demonstrates declaring, initializing, and using variables in C++:
#include <iostream>
int main() {
int age = 30;
double salary = 50000.0;
char grade = 'A';
std::cout << "Age: " << age << std::endl;
std::cout << "Salary: $" << salary << std::endl;
std::cout << "Grade: " << grade << std::endl;
return 0;
}
This program declares and initializes variables for age, salary, and grade, and then prints their values to the console.