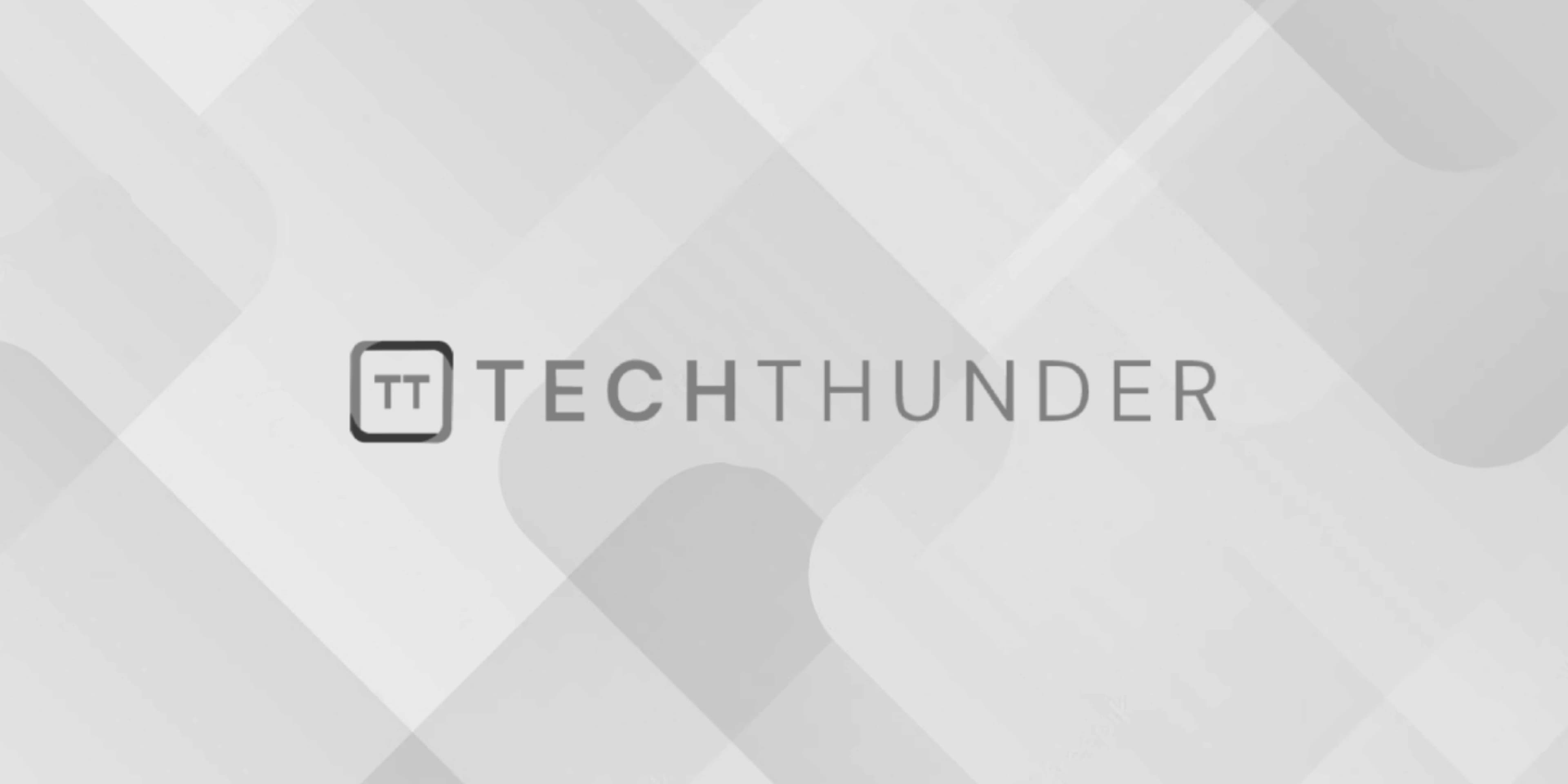
Everything You Need to Know About Conio.h Library Functions in C++
The conio.h
header is not a part of the C++ Standard Library and is typically used in old Turbo C++ and Borland C++ compilers for MS-DOS-based systems. It provides a set of functions for console input and output operations, including screen manipulation, cursor movement, and keyboard handling. However, it’s important to note that the conio.h
library is not portable and not supported on modern platforms.
Here are some commonly used functions from the conio.h
library:
- Screen Manipulation:
clrscr()
: Clears the entire console screen.gotoxy(x, y)
: Moves the cursor to the specified position (columnx
, rowy
).
- Character Input and Output:
getch()
: Reads a single character from the keyboard without echoing it on the screen.getche()
: Reads a single character from the keyboard and echoes it on the screen.
- Color and Text Attributes:
textcolor(color)
: Sets the text color to the specified value (0 to 15).textbackground(color)
: Sets the background color to the specified value (0 to 15).cprintf(format, args)
: Formatted output with color.
- Cursor Visibility:
cursorvisibility(visible)
: Sets the cursor visibility (visible
= 0 for invisible, 1 for visible).
- Delay Functions:
delay(ms)
: Pauses the program for the specified number of milliseconds.delayMicroseconds(us)
: Pauses the program for the specified number of microseconds (not present in all versions).
It’s important to emphasize that the conio.h
library is outdated and not considered a standard or portable part of modern C++ programming. Most modern programming environments, compilers, and operating systems do not support it. If you’re looking to perform console input and output operations in a modern C++ program, you should use the standard C++ streams (cin
, cout
, etc.) and other standard libraries.
Here’s an example using the standard C++ input/output functions to achieve similar behavior without relying on conio.h
:
#include <iostream>
#include <thread> // For sleep_for
#include <chrono> // For milliseconds
int main() {
std::cout << "Hello, this is a C++ program!" << std::endl;
// Wait for 2 seconds
std::this_thread::sleep_for(std::chrono::milliseconds(2000));
return 0;
}
This code achieves screen clearing and delayed output using standard C++ constructs without relying on platform-specific headers like conio.h
.