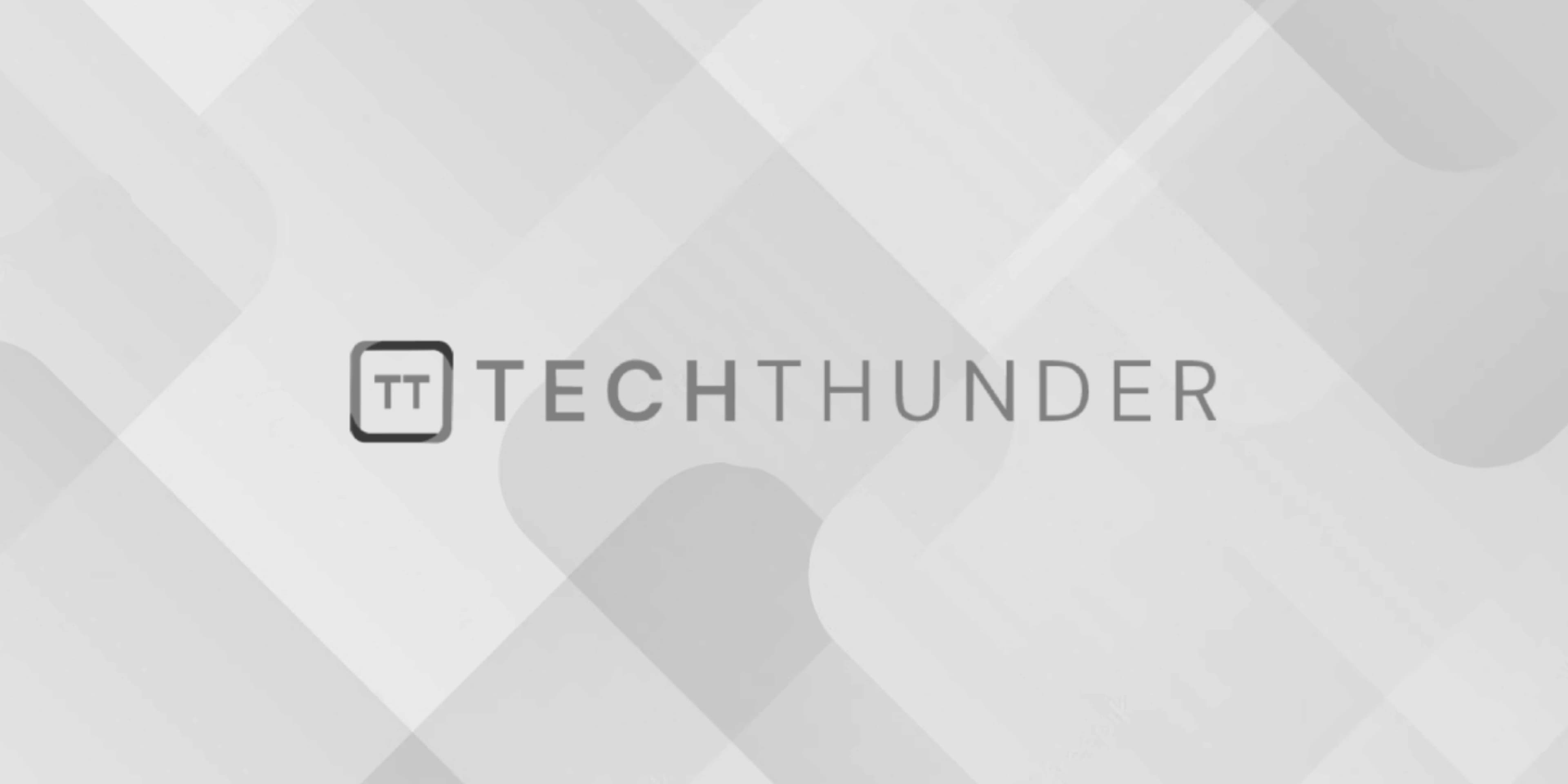
C++ Data Abstraction
Data abstraction is one of the fundamental concepts in object-oriented programming (OOP) and is also a key concept in C++. It refers to the process of hiding the complex implementation details of a data type or class and exposing only the necessary interfaces or operations to the outside world. Data abstraction allows you to represent real-world entities as objects and interact with them through well-defined interfaces, while hiding the underlying complexities.
In C++, data abstraction is achieved through classes and access control mechanisms. Here’s how data abstraction is implemented in C++:
- Classes and Objects: In C++, you define classes to represent objects. A class encapsulates both data members (variables) and member functions (methods) that operate on those data members. Data members can be made private, which means they are hidden from the outside world, and member functions can be used to access or manipulate those data members.
class BankAccount {
private:
double balance; // Data member (hidden)
public:
BankAccount(double initialBalance) : balance(initialBalance) { }
void deposit(double amount) {
// Implementation details here
}
double getBalance() const {
// Implementation details here
}
// Other member functions
};
- Access Control: C++ provides access control keywords such as
private
,protected
, andpublic
to specify the visibility and accessibility of class members.
private
: Members declared as private are only accessible within the class itself. They are hidden from external code.protected
: Members declared as protected are accessible within the class and by derived classes.public
: Members declared as public are accessible from any part of the program. By using these access control keywords, you can control what parts of your class are visible and accessible to the outside world, enforcing data abstraction.
- Encapsulation: Data abstraction is closely related to encapsulation, which is the bundling of data and functions that operate on that data into a single unit (i.e., a class). Encapsulation allows you to control how data is accessed and modified, preventing unauthorized access and ensuring data integrity. In the
BankAccount
example above, thebalance
data member is private, and the member functionsdeposit
andgetBalance
provide controlled access to it. This encapsulation ensures that thebalance
can only be modified through thedeposit
method and read through thegetBalance
method. - Separation of Interface and Implementation: Data abstraction in C++ encourages the separation of the public interface of a class (i.e., the member functions accessible to the outside world) from its implementation details (i.e., the internal data members and private member functions). This separation allows you to change the implementation of a class without affecting the code that uses it, promoting code maintainability and flexibility.
Data abstraction in C++ helps you create well-structured, modular, and maintainable code by hiding complex implementation details and providing clear and controlled interfaces to interact with objects. It also supports the principles of information hiding and reduces code dependencies, which are essential for building robust and scalable software systems.