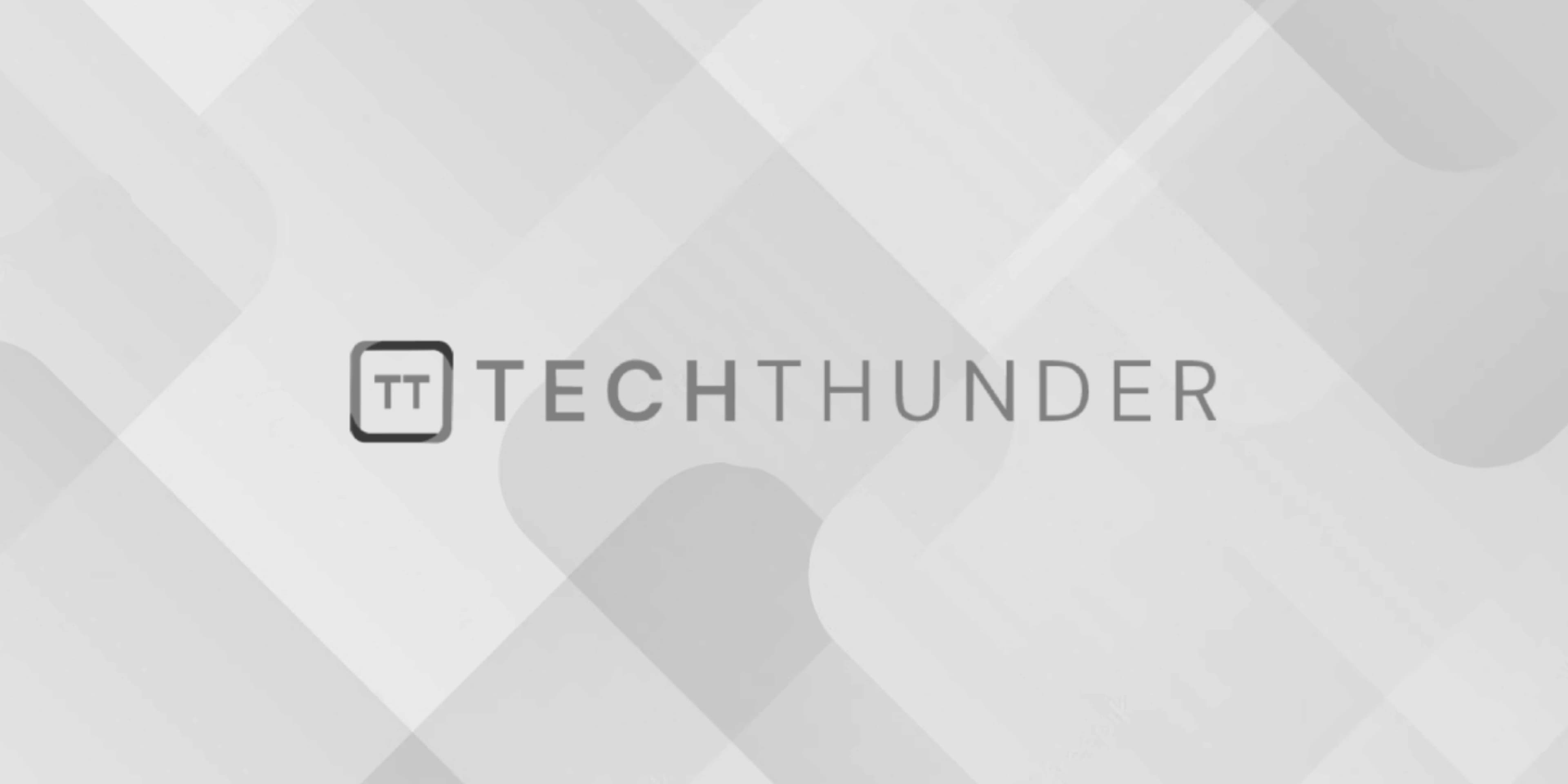
118 views
C++ using the Stack Data Structure
Using the stack data structure in C++ is common for various tasks, such as managing function calls, implementing algorithms, and parsing expressions. Here’s an example of how to use the stack data structure in C++ to perform some basic operations:
C++
#include <iostream>
#include <stack>
int main() {
std::stack<int> myStack; // Create an empty stack of integers
// Push elements onto the stack
myStack.push(10);
myStack.push(20);
myStack.push(30);
// Check if the stack is empty
if (myStack.empty()) {
std::cout << "The stack is empty." << std::endl;
} else {
std::cout << "The stack is not empty." << std::endl;
}
// Get the size of the stack
std::cout << "Size of the stack: " << myStack.size() << std::endl;
// Access and print the top element
std::cout << "Top element: " << myStack.top() << std::endl;
// Pop elements from the stack
myStack.pop();
// Access and print the new top element
std::cout << "Top element after pop: " << myStack.top() << std::endl;
return 0;
}
In this program:
- We include the
<stack>
header to work with the stack data structure. - We create a stack of integers using
std::stack<int> myStack
. - Elements are pushed onto the stack using the
push
method. - We check if the stack is empty using the
empty
method and print whether it’s empty or not. - The
size
method is used to get the size of the stack. - We access and print the top element of the stack using the
top
method. - Elements are popped from the stack using the
pop
method. - Finally, we access and print the new top element after a pop operation.
Compile and run this program to see how the stack data structure works in C++.