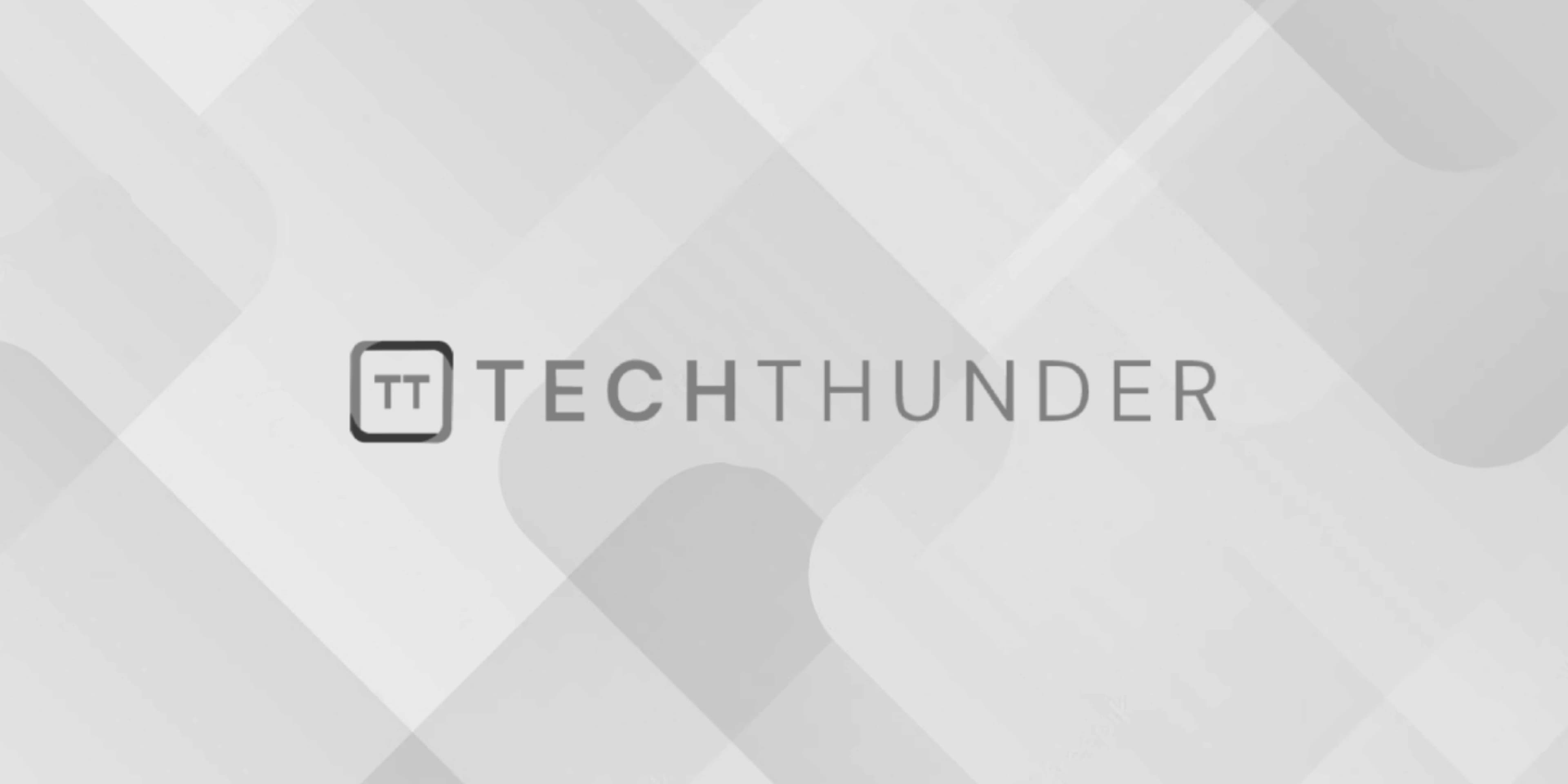
116 views
Static Keyword in C++ and JAVA
The static
keyword in C++ and Java is used to denote various features and behaviors, and its meaning can differ between the two languages. Here’s an overview of how static
is used in both languages:
In C++:
- Static Variables (Static Member Variables): In C++, when you declare a variable as
static
within a class, it becomes a static member variable. These variables are shared among all instances of the class, and they are initialized only once, typically in the class definition or in a separate source file.
C++
class MyClass {
public:
static int staticVar;
};
// Initialize the static variable outside the class definition
int MyClass::staticVar = 0;
- Static Member Functions: A
static
member function in C++ belongs to the class rather than a specific instance of the class. It can be called using the class name, without creating an object of the class.
C++
class MyClass {
public:
static void staticFunction() {
// ...
}
};
// Call the static function without an object
MyClass::staticFunction();
- Static Local Variables: In C++,
static
can also be used with local variables inside functions. It makes the variable retain its value between function calls.
C++
void myFunction() {
static int localVar = 0;
localVar++;
// localVar retains its value between calls
}
In Java:
- Static Variables (Class Variables): In Java, a
static
variable declared within a class is known as a class variable. These variables belong to the class rather than instances of the class. They are shared among all instances of the class.
C++
public class MyClass {
public static int staticVar;
}
- Static Methods: Similar to C++, Java has static methods that belong to the class rather than instances. They can be called using the class name.
C++
public class MyClass {
public static void staticMethod() {
// ...
}
}
// Call the static method using the class name
MyClass.staticMethod();
- Static Blocks: Java allows the use of static blocks (static initialization blocks) to initialize static variables or perform other initialization tasks. These blocks are executed when the class is loaded.
C++
public class MyClass {
static {
// Static block code
}
}
- Static Nested Classes: Java allows you to define nested classes as static, which means they are associated with the outer class and do not require an instance of the outer class to be created.
C++
public class OuterClass {
static class NestedClass {
// ...
}
}
In summary, while the static
keyword is used in both C++ and Java, its usage and behavior can vary between the two languages. In C++, it is commonly used for static member variables and functions, whereas in Java, it is used for class variables, methods, static blocks, and nested classes.