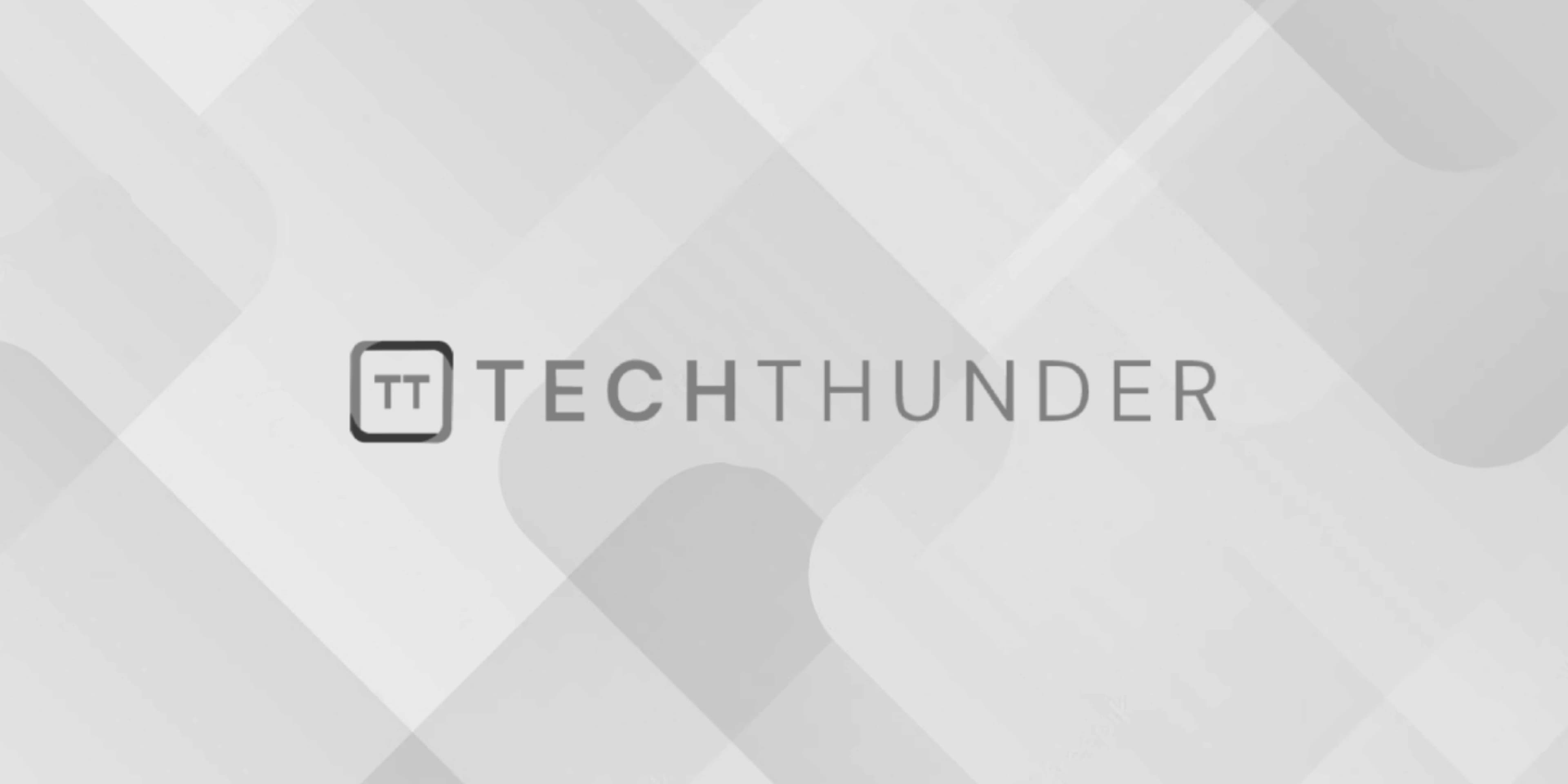
175 views
Declare C++ Function Returning Pointer to Array of Integer Pointers
The C++ can declare a function that returns a pointer to an array of integer pointers like this:
C++
int** (*functionName)();
Here’s an example of how to declare a function with this signature:
C++
#include <iostream>
// Declare a function returning a pointer to an array of integer pointers
int** (*getArrayOfPointers())();
int main() {
// Call the function to get the array of integer pointers
int** (*ptrToFunc)() = getArrayOfPointers();
int* intArray1 = new int[3]{1, 2, 3};
int* intArray2 = new int[2]{4, 5};
int** arrayOfPointers = ptrToFunc();
arrayOfPointers[0] = intArray1;
arrayOfPointers[1] = intArray2;
std::cout << "Value from array 1: " << arrayOfPointers[0][1] << std::endl; // Outputs 2
std::cout << "Value from array 2: " << arrayOfPointers[1][0] << std::endl; // Outputs 4
// Clean up memory (not shown in this example for simplicity)
return 0;
}
int** (*getArrayOfPointers())() {
// Create and return a pointer to an array of integer pointers
int** (*arrayOfPointers)() = []() -> int** {
int** arr = new int*[2];
return arr;
};
return arrayOfPointers;
}
In this example:
getArrayOfPointers
is a function that returns a pointer to an array of integer pointers. It creates and returns an array of integer pointers with a size of 2.- In the
main
function, we callgetArrayOfPointers()
to obtain a pointer to the array of integer pointers. - We allocate memory for two integer arrays (
intArray1
andintArray2
) and assign them to the elements of the array of integer pointers. - Finally, we access and print values from these arrays to demonstrate the usage.
Remember to free the allocated memory when you’re done with it to avoid memory leaks. The cleanup is not shown in this example for simplicity.