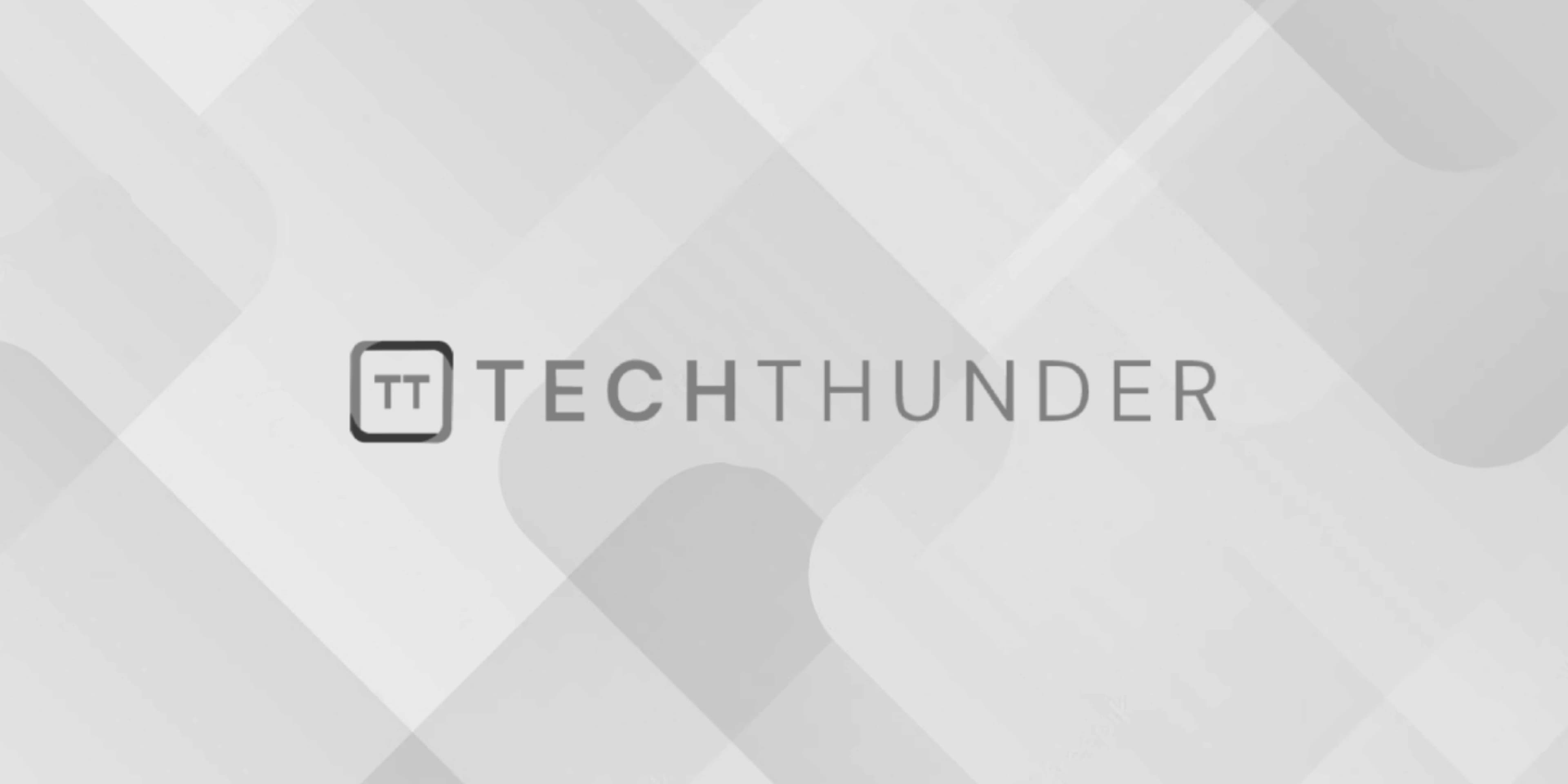
82 views
Currency Converter in C++
Creating a currency converter in C++ involves getting exchange rates, taking user input, and performing the conversion calculation. Here’s a basic console-based currency converter using fixed exchange rates. Please note that real-world currency conversion requires up-to-date exchange rate data from a reliable source.
C++
#include <iostream>
#include <map>
#include <iomanip>
using namespace std;
// Function to convert currency
double convertCurrency(double amount, double exchangeRate) {
return amount * exchangeRate;
}
int main() {
// Exchange rates (example rates, you should use actual rates)
map<string, double> exchangeRates;
exchangeRates["USD"] = 1.0;
exchangeRates["EUR"] = 0.85;
exchangeRates["GBP"] = 0.75;
cout << "Currency Converter" << endl;
// Input currency and amount
string fromCurrency, toCurrency;
double amount;
cout << "Enter the source currency (e.g., USD): ";
cin >> fromCurrency;
if (exchangeRates.find(fromCurrency) == exchangeRates.end()) {
cout << "Invalid source currency." << endl;
return 1;
}
cout << "Enter the target currency (e.g., EUR): ";
cin >> toCurrency;
if (exchangeRates.find(toCurrency) == exchangeRates.end()) {
cout << "Invalid target currency." << endl;
return 1;
}
cout << "Enter the amount to convert: ";
cin >> amount;
// Perform the currency conversion
double convertedAmount = convertCurrency(amount, exchangeRates[toCurrency] / exchangeRates[fromCurrency]);
// Display the result
cout << fixed << setprecision(2);
cout << amount << " " << fromCurrency << " = " << convertedAmount << " " << toCurrency << endl;
return 0;
}
In this example:
- We define exchange rates as a map where the keys are currency codes (e.g., USD, EUR) and the values are the exchange rates relative to the base currency (in this case, USD).
- The program takes user input for the source currency, target currency, and the amount to convert.
- The
convertCurrency
function performs the currency conversion using the exchange rates from the map. - The result is displayed with two decimal places of precision.
Please note that real-world currency conversion applications should use up-to-date exchange rate data from a reliable source, and the conversion rates should be regularly updated. This example serves as a basic illustration of the concept and should not be used for real financial transactions.