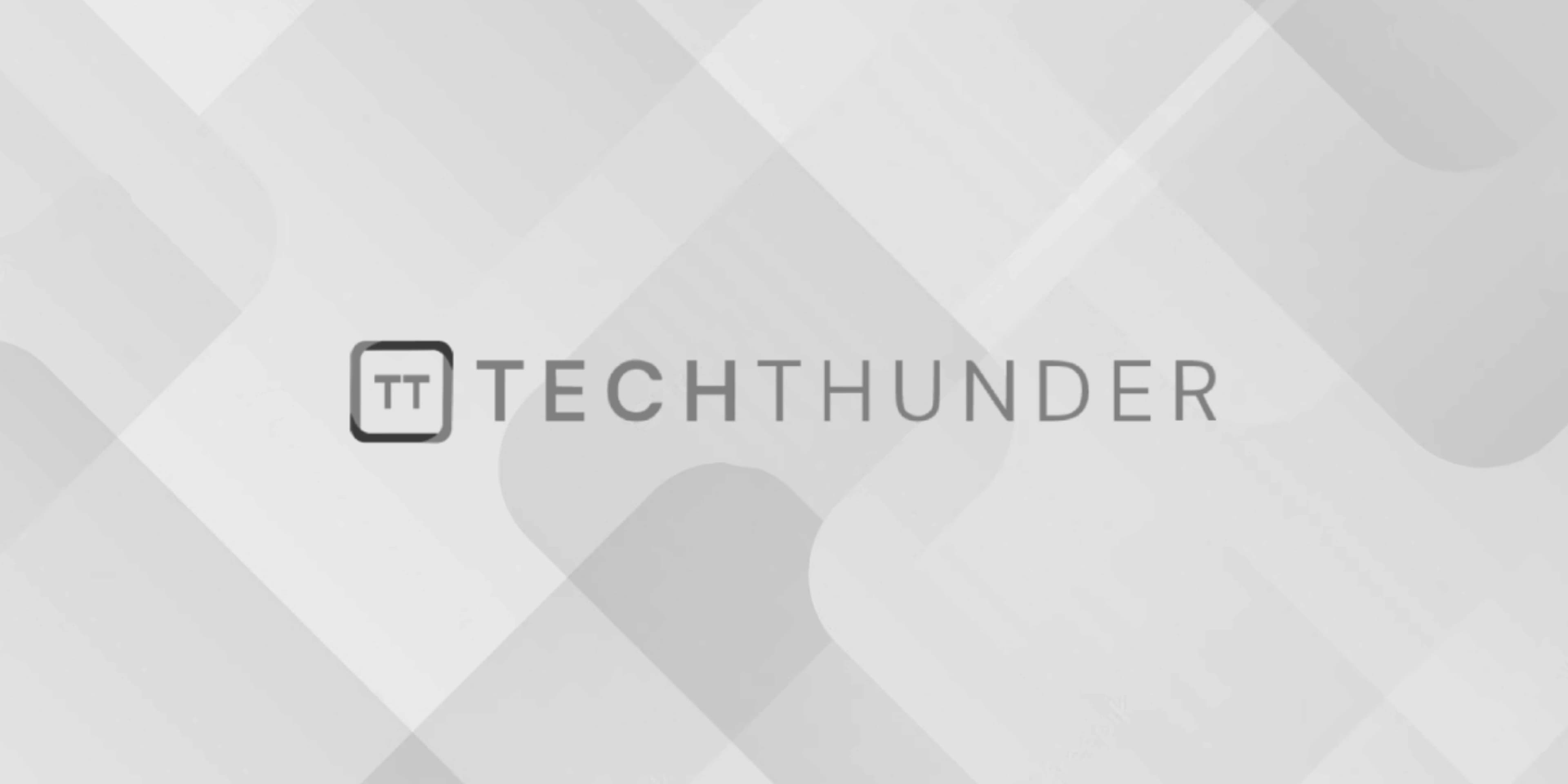
153 views
C++ ‘Using’ vs ‘Typedef’
The C++, both using
and typedef
are used for creating type aliases, which allow you to define a new name for an existing data type. However, they have different syntax and some subtle differences in usage. Here’s a comparison between using
and typedef
:
typedef
:
C++
typedef existing_type new_type_name;
- Syntax:
typedef
is followed by the existing data type (existing_type
) and the new name you want to create for it (new_type_name
). - Scope:
typedef
creates a new type name within the current scope. It’s not block-scoped like variables, so it applies globally within that scope. - Compatibility:
typedef
is compatible with C and is an older way of creating type aliases in C++. - Usage Example:
C++
typedef int MyInt; // Creating an alias MyInt for int
MyInt x = 42; // You can now use MyInt like int
using
:
C++
using new_type_name = existing_type;
- Syntax:
using
is followed by the new name you want to create for the type (new_type_name
), then the assignment (=
), and the existing data type (existing_type
). - Scope:
using
creates a new type name within the current scope, just liketypedef
. It also allows for more complex type aliases using templates. - Compatibility:
using
is a more modern way of creating type aliases in C++ and is the preferred approach. - Usage Example:
C++
using MyInt = int; // Creating an alias MyInt for int
MyInt x = 42; // You can now use MyInt like int
Key Differences:
- Syntax: The syntax for
typedef
involves placing the existing type before the new name, whileusing
places the new name before the existing type. - Readability: Many developers find the
using
syntax more readable, especially when defining complex type aliases. - Template Aliases:
using
can be used to create template aliases, allowing you to create type aliases for templates with specific type arguments. This is not possible withtypedef
.
C++
template <typename T>
using Vec = std::vector<T>;
Vec<int> v; // Equivalent to std::vector<int> v;
In modern C++ code, it’s recommended to use using
for type aliases because it offers more flexibility and is easier to read, especially when working with templates. However, if you’re maintaining or working with legacy codebases, you may encounter typedef
as well.