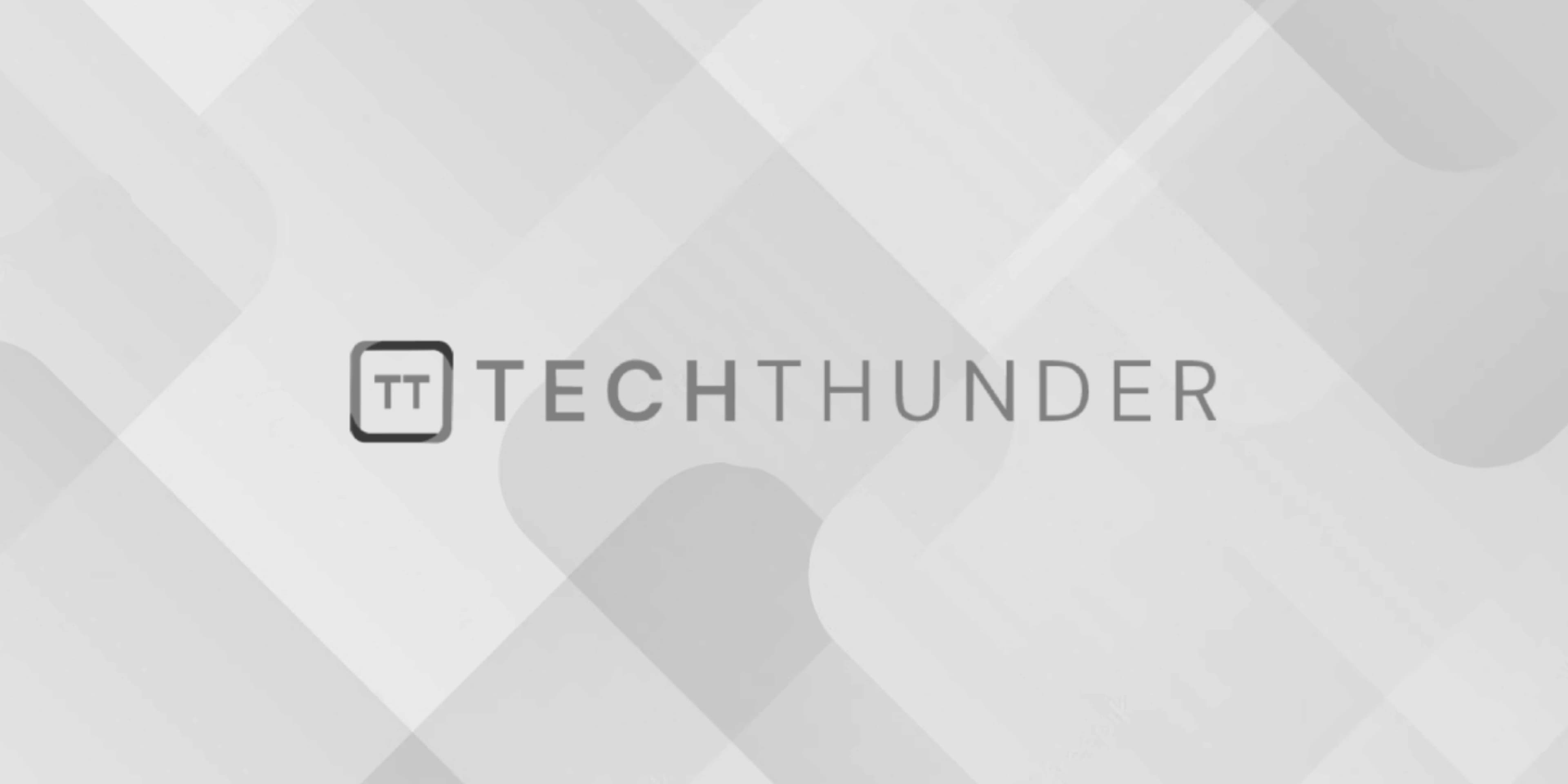
Assertions in C++
Assertions in C++ are a mechanism for incorporating runtime checks into your code to catch and report errors or unexpected conditions. They are typically used for debugging and testing purposes. Assertions are defined using the assert
macro, which is part of the <cassert>
(or <assert.h>
in C) header.
Here’s how assertions work in C++:
- Including the Header: You need to include the
<cassert>
header at the beginning of your C++ source code to use assertions:
#include <cassert>
- Writing Assertions: The
assert
macro takes an expression as its argument. If the expression evaluates tofalse
(i.e., the condition is not met), an error message is printed, and the program terminates. If the expression evaluates totrue
, the program continues to execute without any issues.
assert(expression);
For example:
int x = 10;
int y = 20;
assert(x > y); // This assertion will fail, and the program will terminate.
- Compile-Time Control: By default, assertions are active in debug builds (e.g., when compiling with debugging flags like
-g
), but they are not active in release builds (when optimizations are enabled). This means that assertions have no impact on the performance of the release version of your code. - Custom Error Messages: You can provide a custom error message as a string after the expression in the
assert
macro. This message is displayed when the assertion fails and can help you identify the cause of the error.
assert(expression && "Custom error message here");
For example:
int divisor = 0;
assert(divisor != 0 && "Division by zero is not allowed");
- Disabling Assertions: If you want to disable assertions in debug builds, you can define the
NDEBUG
macro before including<cassert>
. This will deactivate allassert
statements in your code:
#define NDEBUG
#include <cassert>
Remember to remove or comment out the #define NDEBUG
line to reactivate assertions when needed.
- Common Use Cases:
- Checking preconditions and postconditions in functions.
- Verifying that assumptions about your code are valid.
- Detecting programming errors during development and testing.
Assertions are a valuable tool for catching bugs early in the development process and ensuring that your code behaves as expected. However, they should not be used as a replacement for proper error handling and validation in production code. When writing production-ready software, consider using exceptions and error handling mechanisms to gracefully handle errors.