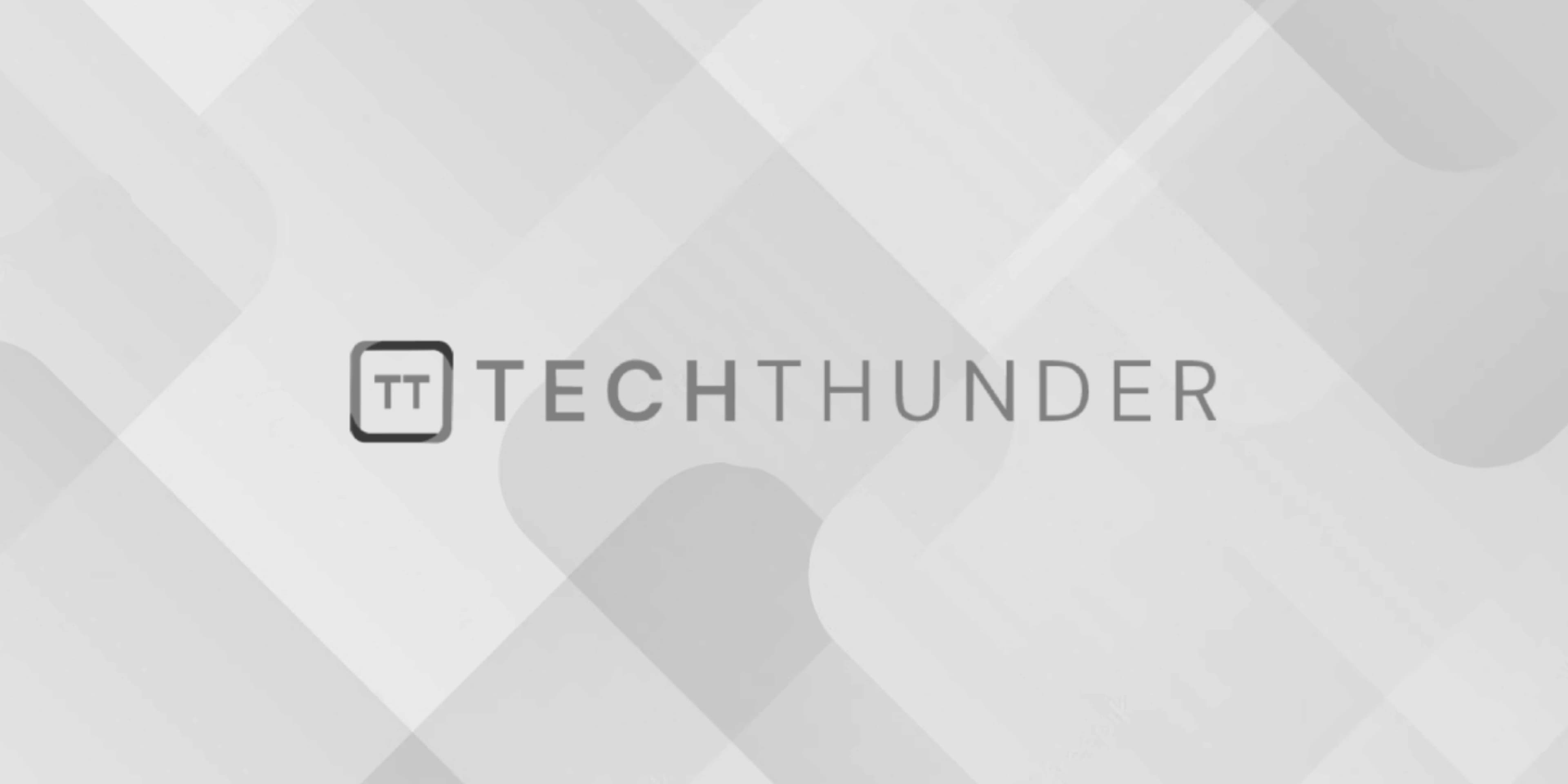
271 views
HASHSET IN C++
The C++ std::unordered_set
is a container provided by the C++ Standard Library that stores a collection of unique elements. It is based on a hash table data structure and allows for fast insertion, removal, and lookup of elements. Here’s how you can use std::unordered_set
:
TypeScript
#include <iostream>
#include <unordered_set>
int main() {
// Declare an unordered_set of integers
std::unordered_set<int> mySet;
// Insert elements into the unordered_set
mySet.insert(10);
mySet.insert(20);
mySet.insert(30);
// Check if an element exists in the unordered_set
if (mySet.find(20) != mySet.end()) {
std::cout << "Element 20 is in the set." << std::endl;
} else {
std::cout << "Element 20 is not in the set." << std::endl;
}
// Iterate through the unordered_set
for (int element : mySet) {
std::cout << element << " ";
}
std::cout << std::endl;
// Remove an element from the unordered_set
mySet.erase(20);
// Check the size of the unordered_set
std::cout << "Size of the set: " << mySet.size() << std::endl;
return 0;
}
In this example:
- We include the necessary headers,
<iostream>
and<unordered_set>
. - We declare an
std::unordered_set
calledmySet
to store integers. - We insert elements into the unordered_set using the
insert
method. - We check if an element exists in the unordered_set using the
find
method. - We iterate through the unordered_set using a range-based for loop.
- We remove an element from the unordered_set using the
erase
method. - We check the size of the unordered_set using the
size
method.
std::unordered_set
is a useful container when you need to store a collection of unique elements and perform fast insertion, removal, and lookup operations. The order of elements in an std::unordered_set
is not guaranteed to be in any particular order; it depends on the hash function and the underlying implementation.