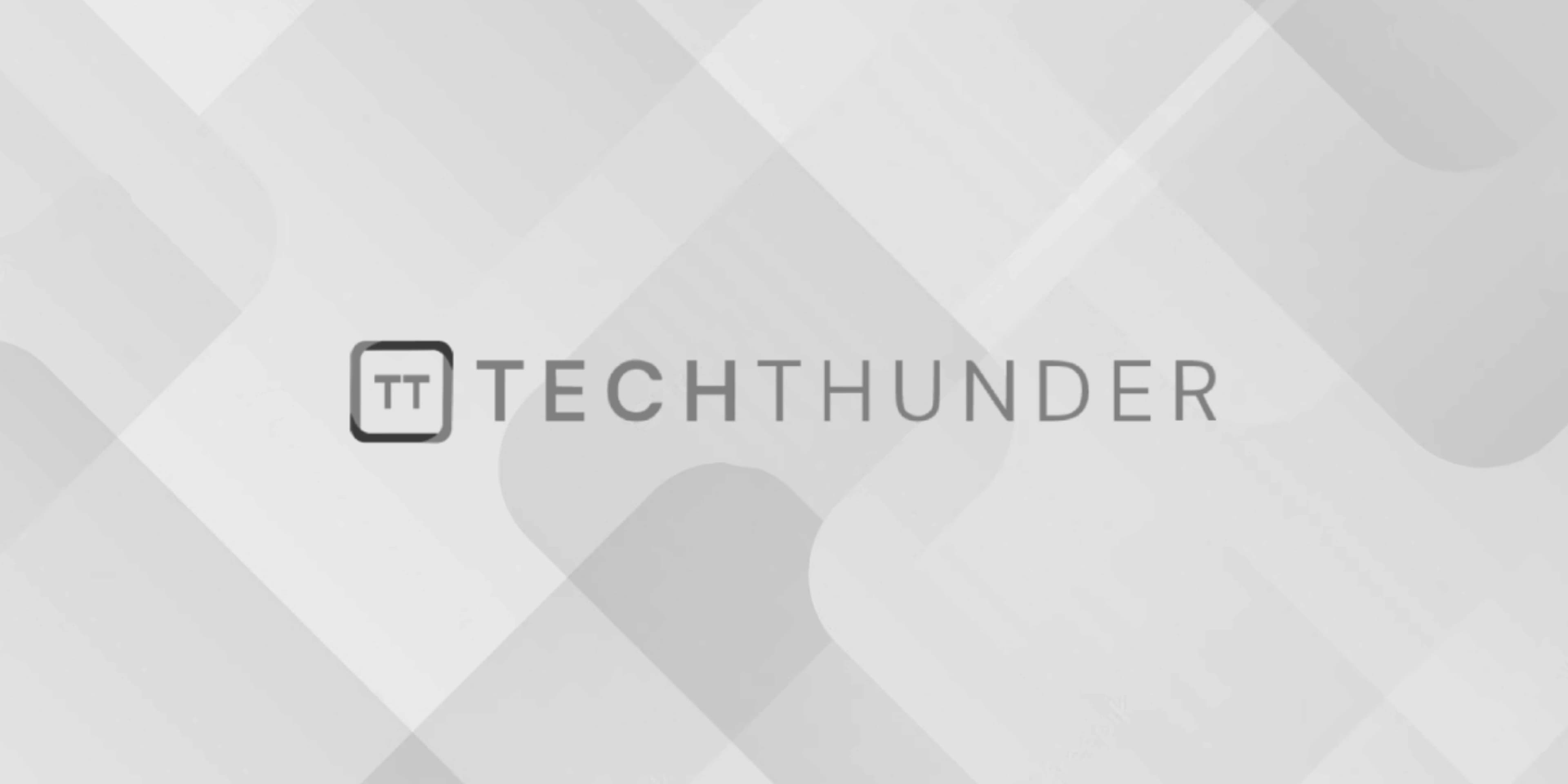
Types of polymorphism in C++
Polymorphism is one of the fundamental concepts in object-oriented programming and plays a central role in C++. It allows objects of different classes to be treated as objects of a common base class. In C++, there are two main types of polymorphism:
- Compile-Time Polymorphism (Static Binding):
- Compile-time polymorphism, also known as static polymorphism or early binding, is resolved at compile time.
- It is achieved through function overloading and operator overloading.
- Function Overloading: In function overloading, multiple functions in the same scope have the same name but different parameter lists. The correct function to call is determined at compile time based on the number and types of arguments.
- Operator Overloading: C++ allows you to redefine the behavior of operators for user-defined types. Operator overloading enables compile-time polymorphism. Example of function overloading:
int add(int a, int b) {
return a + b;
}
double add(double a, double b) {
return a + b;
}
Example of operator overloading:
class Complex {
public:
Complex operator+(const Complex& other) {
Complex result;
result.real = this->real + other.real;
result.imaginary = this->imaginary + other.imaginary;
return result;
}
private:
double real;
double imaginary;
};
- Run-Time Polymorphism (Dynamic Binding):
- Run-time polymorphism, also known as dynamic polymorphism or late binding, is resolved at runtime.
- It is achieved through virtual functions and inheritance.
- Virtual Functions: A virtual function is a function declared in a base class and overridden in derived classes. The actual function called is determined at runtime based on the object’s dynamic type.
- Inheritance: Run-time polymorphism is closely related to inheritance. It allows objects of derived classes to be treated as objects of the base class, and the appropriate function is called based on the object’s type. Example of virtual functions:
class Animal {
public:
virtual void makeSound() {
std::cout << "Animal makes a sound." << std::endl;
}
};
class Dog : public Animal {
public:
void makeSound() override {
std::cout << "Dog barks." << std::endl;
}
};
int main() {
Animal* myAnimal = new Dog;
myAnimal->makeSound(); // Calls Dog's makeSound at runtime.
delete myAnimal;
return 0;
}
Run-time polymorphism is a powerful concept as it allows for more flexibility and extensibility in code, particularly in situations where you need to work with objects of different derived classes through a common base class interface.
In summary, C++ supports both compile-time polymorphism through function overloading and operator overloading and run-time polymorphism through virtual functions and inheritance. Run-time polymorphism is a key feature of object-oriented programming that allows you to write more dynamic and flexible code.