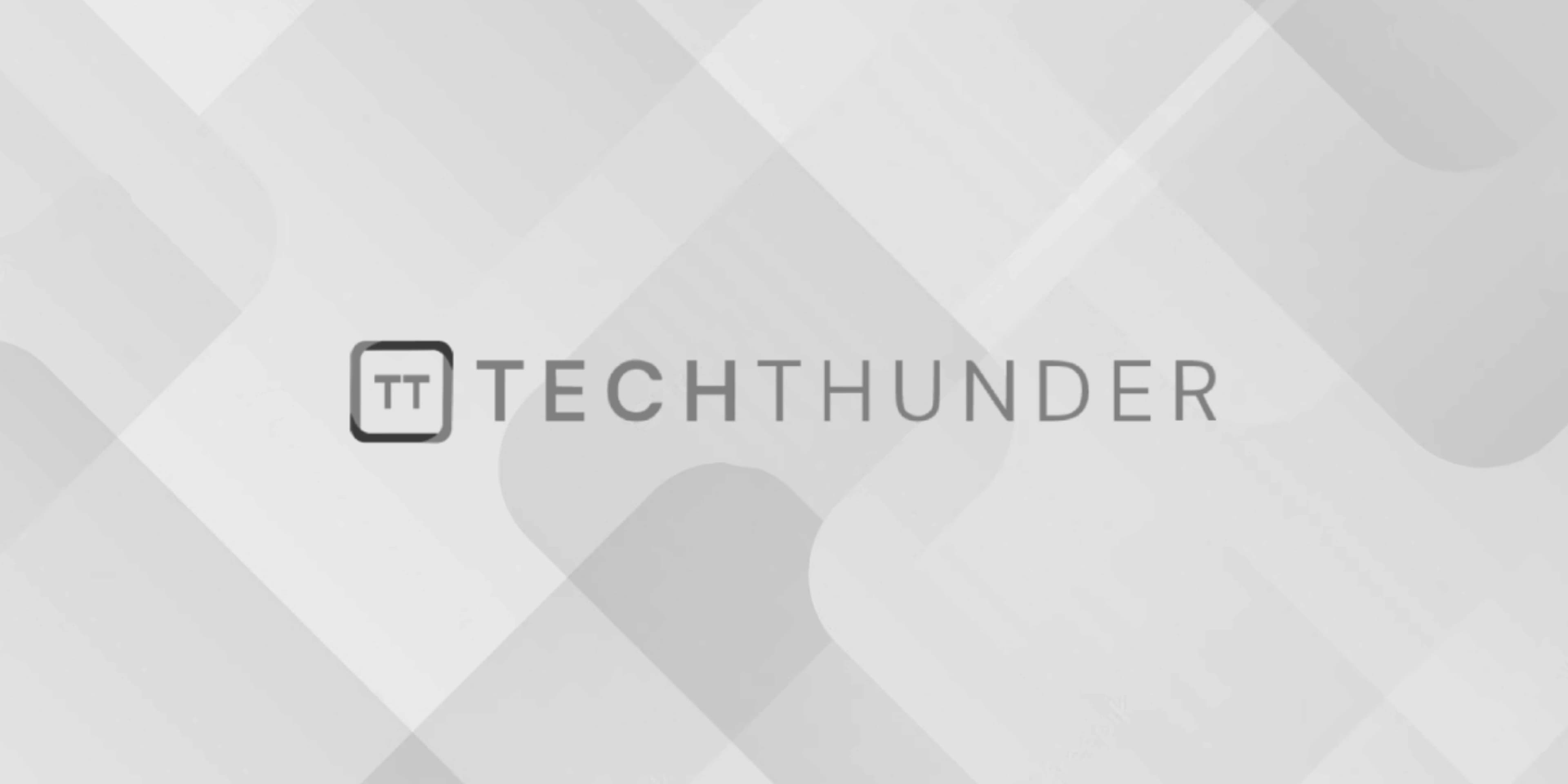
C++ Namespaces
The C++ namespaces are a way to organize code by grouping related classes, functions, variables, and other identifiers into named containers. This helps prevent naming conflicts and makes it easier to manage and maintain large codebases. The C++ Standard Library itself uses namespaces extensively to organize its components.
Here’s how you can define and use namespaces in C++:
- Defining a Namespace: You can define your own namespace using the
namespace
keyword:
// Define a namespace called "MyNamespace"
namespace MyNamespace {
int x = 42;
void foo() {
// ...
}
}
- Accessing Namespaced Elements: To access elements within a namespace, you can use the
::
scope resolution operator:
int main() {
// Access the variable 'x' from the namespace 'MyNamespace'
int value = MyNamespace::x;
// Access the function 'foo' from the namespace 'MyNamespace'
MyNamespace::foo();
return 0;
}
- Using Directives: You can simplify your code by using
using
directives to bring the entire namespace or specific elements into the current scope:
using namespace MyNamespace; // Import all elements from 'MyNamespace' into the current scope
int main() {
int value = x; // 'x' is now accessible directly
foo(); // 'foo' is also accessible directly
return 0;
}
Be cautious when using using namespace
directives, as they can potentially lead to naming conflicts if different namespaces have identifiers with the same name.
- Nested and Anonymous Namespaces: You can nest namespaces to create hierarchical organization:
namespace Outer {
int x = 42;
namespace Inner {
int y = 100;
}
}
int main() {
int value1 = Outer::x;
int value2 = Outer::Inner::y;
return 0;
}
You can also create anonymous namespaces, which have the effect of making identifiers within them effectively local to the translation unit (source file):
namespace {
int localVar = 10; // This is not accessible outside this source file
}
int main() {
int value = localVar; // Error: 'localVar' is not accessible here
return 0;
}
Namespaces provide a powerful mechanism for organizing and managing code in C++. They are particularly useful in large projects and when dealing with third-party libraries to prevent conflicts between different parts of the codebase.