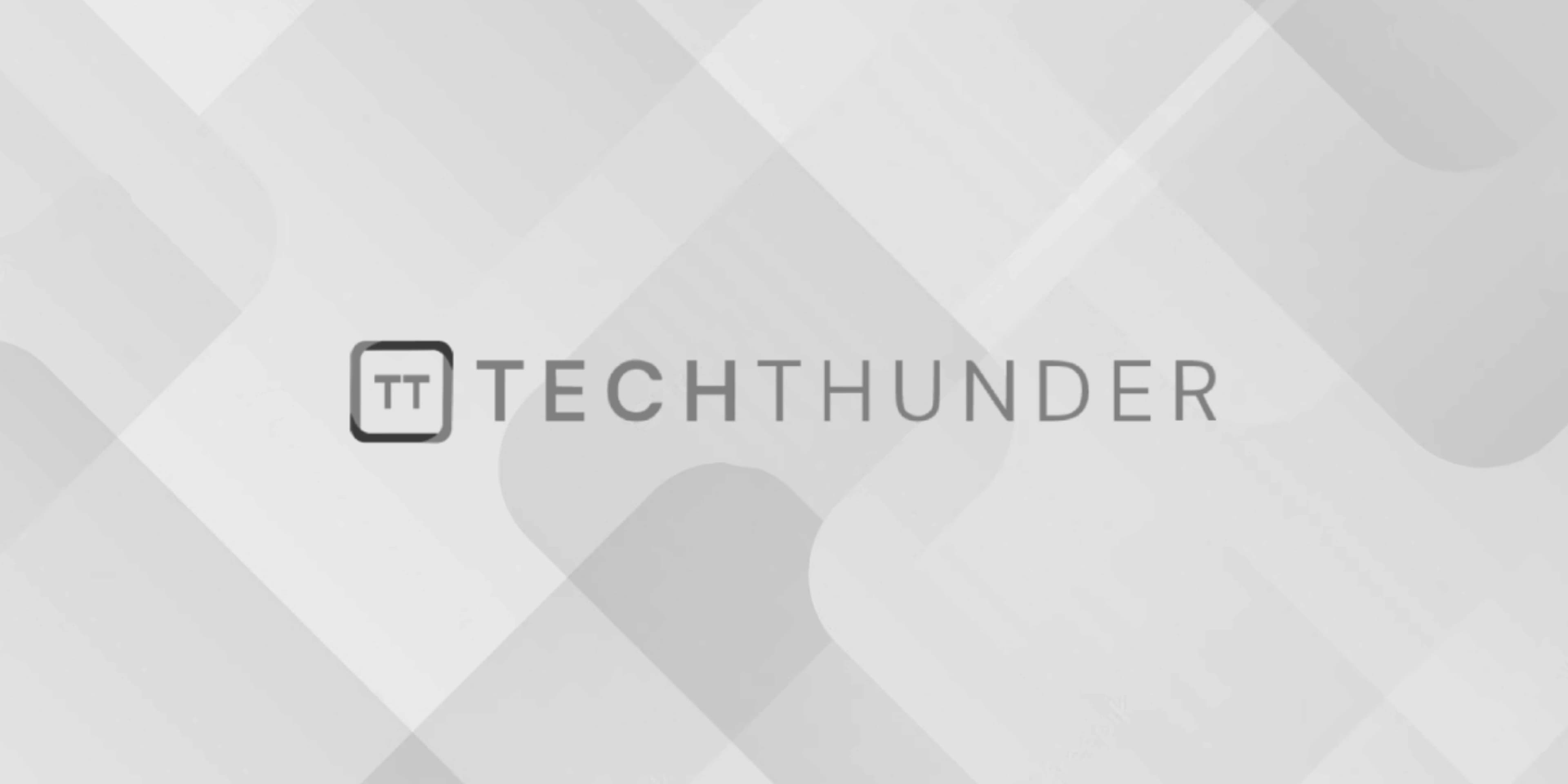
Diamond Problem in C++
The “Diamond Problem” is a specific issue that can arise in C++ when a class inherits from multiple base classes that have a common base class. This situation leads to ambiguity in the derived class because it inherits multiple instances of the common base class. It is called the “Diamond Problem” because the inheritance diagram forms a diamond shape.
Here’s a visual representation of the Diamond Problem:
A
/ \
B C
\ /
D
In this diagram, class D
inherits from both classes B
and C
, and both B
and C
inherit from class A
. So, when you create an object of class D
, there are two copies of class A
in its inheritance hierarchy, leading to ambiguity.
To resolve the Diamond Problem in C++, you can use one of the following methods:
- Virtual Inheritance: You can use the
virtual
keyword during inheritance to indicate that the base class should be virtually inherited. This means that there will be only one instance of the common base class in the hierarchy.
class A {
// ...
};
class B : virtual public A {
// ...
};
class C : virtual public A {
// ...
};
class D : public B, public C {
// ...
};
Using virtual inheritance ensures that there’s only one instance of class A
in class D
.
- Override Ambiguous Methods: If you have ambiguous methods due to the Diamond Problem, you can override them in the derived class to provide a specific implementation.
class A {
public:
virtual void foo() { /* A's implementation */ }
};
class B : public A {
public:
void foo() override { /* B's implementation */ }
};
class C : public A {
public:
void foo() override { /* C's implementation */ }
};
class D : public B, public C {
public:
// Override the ambiguous foo method in D
void foo() override { /* D's implementation */ }
};
In this case, you provide an implementation of foo
in class D
to resolve the ambiguity.
- Use Explicit Scope Resolution: You can explicitly specify the base class when calling ambiguous methods to disambiguate the call.
D obj;
obj.B::foo(); // Call B's foo
obj.C::foo(); // Call C's foo
This allows you to choose which version of the method to call.
The choice of method depends on your specific use case and design requirements. Virtual inheritance is often used when you want to ensure a single instance of the common base class, while method overriding and explicit scope resolution are used when you need more control over the behavior of derived classes.