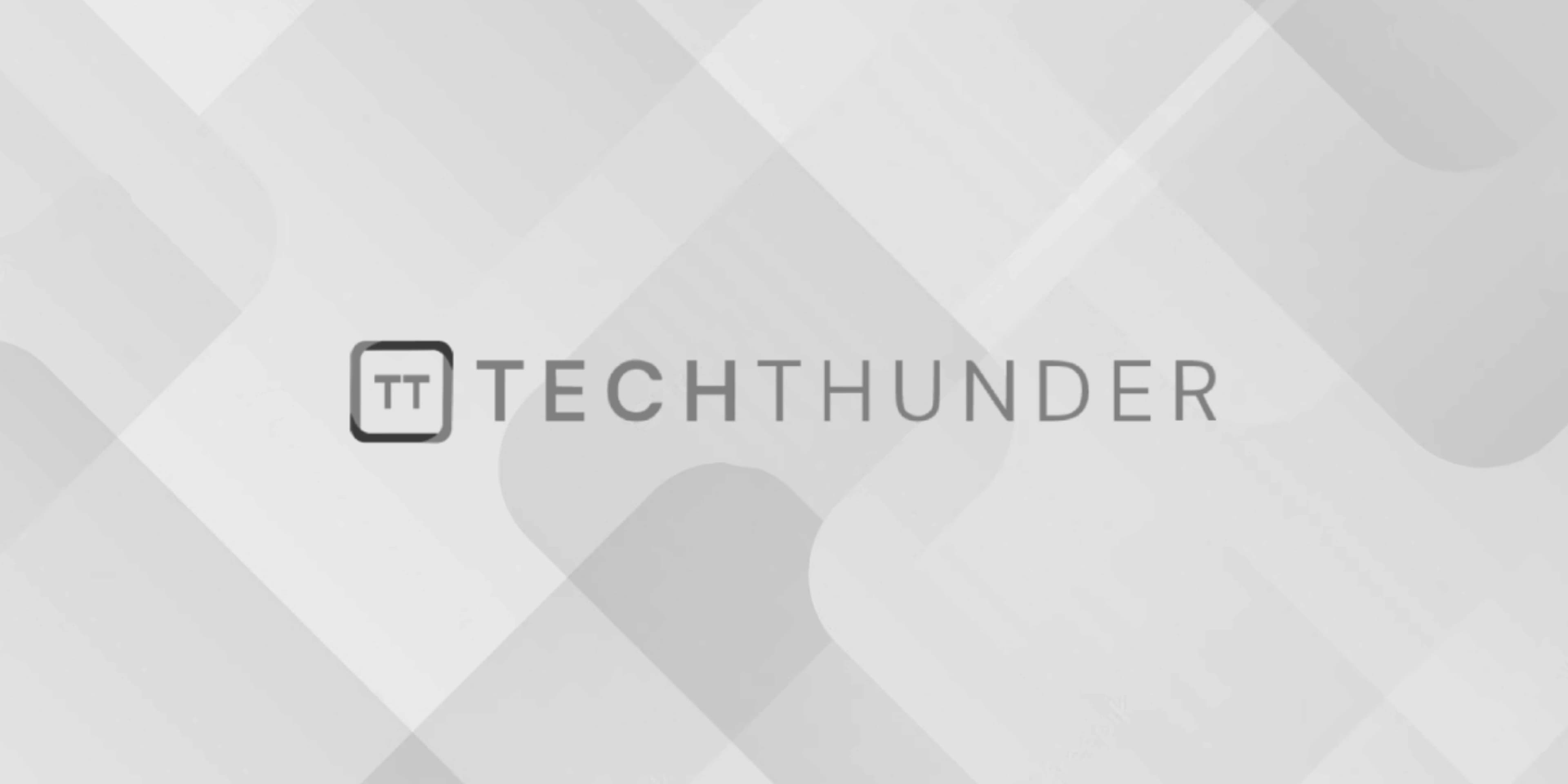
302 views
C++ DSA
C++ is a versatile programming language that’s widely used for various applications, including data structures and algorithms (DSA) implementations. Data structures and algorithms are essential topics in computer science, as they help programmers efficiently organize and manipulate data to solve problems. Here’s a brief overview of some common data structures and algorithms in the context of C++:
Data Structures:
- Arrays: A collection of elements of the same type stored in contiguous memory locations.
- Linked Lists: A collection of nodes, where each node contains both data and a reference to the next node in the sequence.
- Stacks: A linear data structure that follows the Last-In-First-Out (LIFO) principle.
- Queues: A linear data structure that follows the First-In-First-Out (FIFO) principle.
- Trees: Hierarchical structures with nodes connected by edges, commonly used for organizing hierarchical data.
- Graphs: A collection of nodes (vertices) and edges that connect pairs of nodes.
Algorithms:
- Sorting Algorithms: Methods to rearrange elements in a particular order, such as bubble sort, insertion sort, selection sort, merge sort, and quicksort.
- Searching Algorithms: Methods to find a particular element in a data structure, such as linear search and binary search.
- Recursion: A technique where a function calls itself to solve a smaller instance of a problem.
- Dynamic Programming: A technique used to solve problems by breaking them down into smaller subproblems and storing their solutions to avoid redundant computations