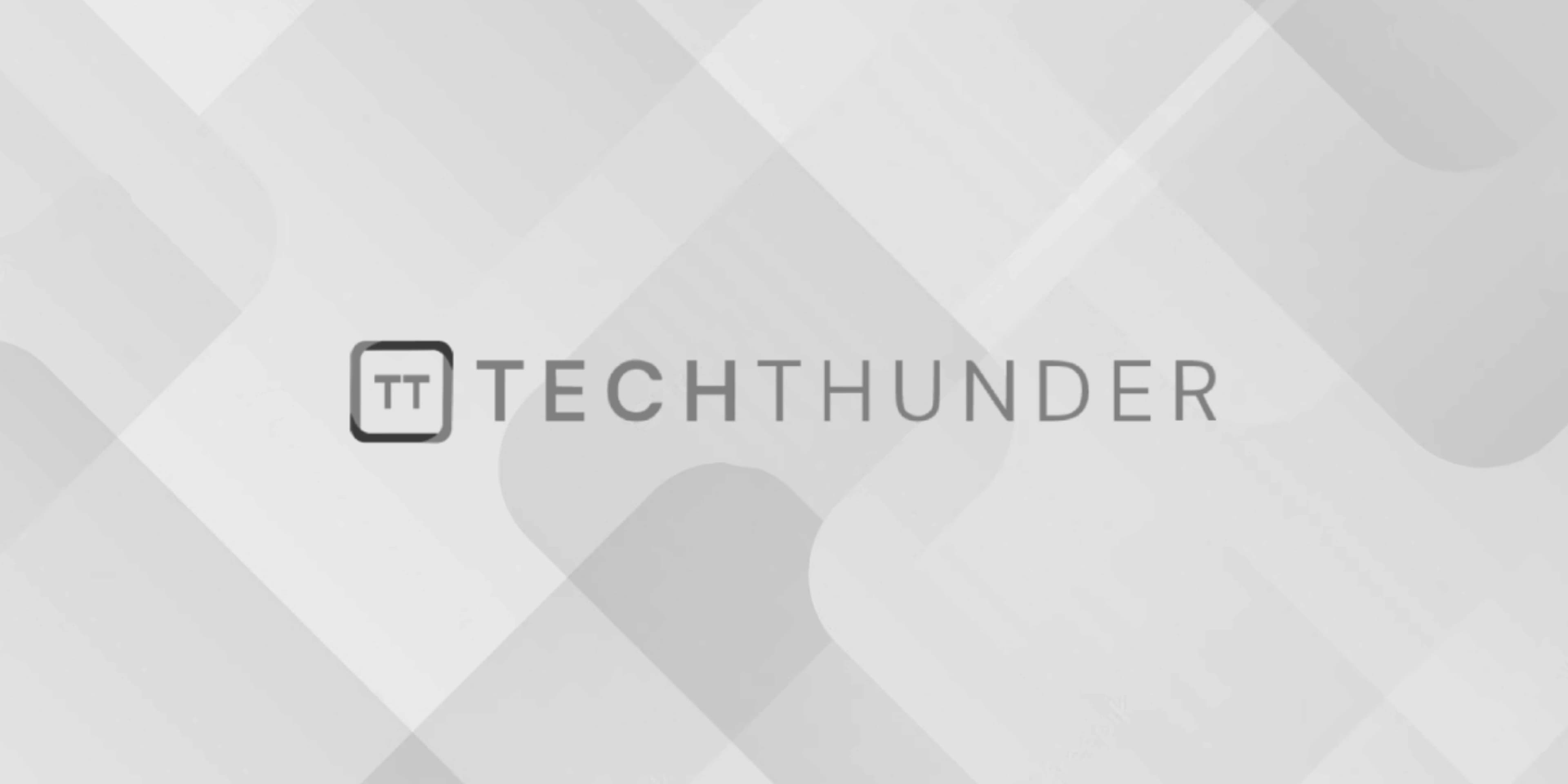
142 views
Opening and Closing a File in C in C++ Pdf
To open and close a file in C++ for reading and writing, you can use the fstream
class, which provides file stream capabilities. Here’s how to open and close a file in C++ using fstream
for both reading and writing, and then convert it to a PDF:
C++
#include <iostream>
#include <fstream>
#include <string>
int main() {
// Open a file for writing (or create if it doesn't exist)
std::ofstream outFile("example.txt");
if (!outFile.is_open()) {
std::cerr << "Failed to open the file for writing." << std::endl;
return 1;
}
// Write some data to the file
outFile << "Hello, World!" << std::endl;
outFile << "This is a test file." << std::endl;
// Close the file
outFile.close();
// Open the same file for reading
std::ifstream inFile("example.txt");
if (!inFile.is_open()) {
std::cerr << "Failed to open the file for reading." << std::endl;
return 1;
}
// Read and display the contents of the file
std::string line;
while (std::getline(inFile, line)) {
std::cout << line << std::endl;
}
// Close the file
inFile.close();
return 0;
}
In this example:
- We first open a file named “example.txt” for writing using
std::ofstream
. If the file doesn’t exist, it will be created. If it fails to open, an error message is displayed. - We write some data to the file using the
<<
operator. - We then close the file using the
close
method. - Next, we open the same file for reading using
std::ifstream
. Again, if it fails to open, an error message is displayed. - We read and display the contents of the file line by line using
std::getline
. - Finally, we close the file for reading.
To convert this text file into a PDF, you would typically need a library or tool designed for PDF generation, as a simple text file doesn’t have the formatting and structure necessary for a PDF document. Libraries like PDFlib or tools like LaTeX can be used to create PDF documents from text content, but they require additional setup and configuration.