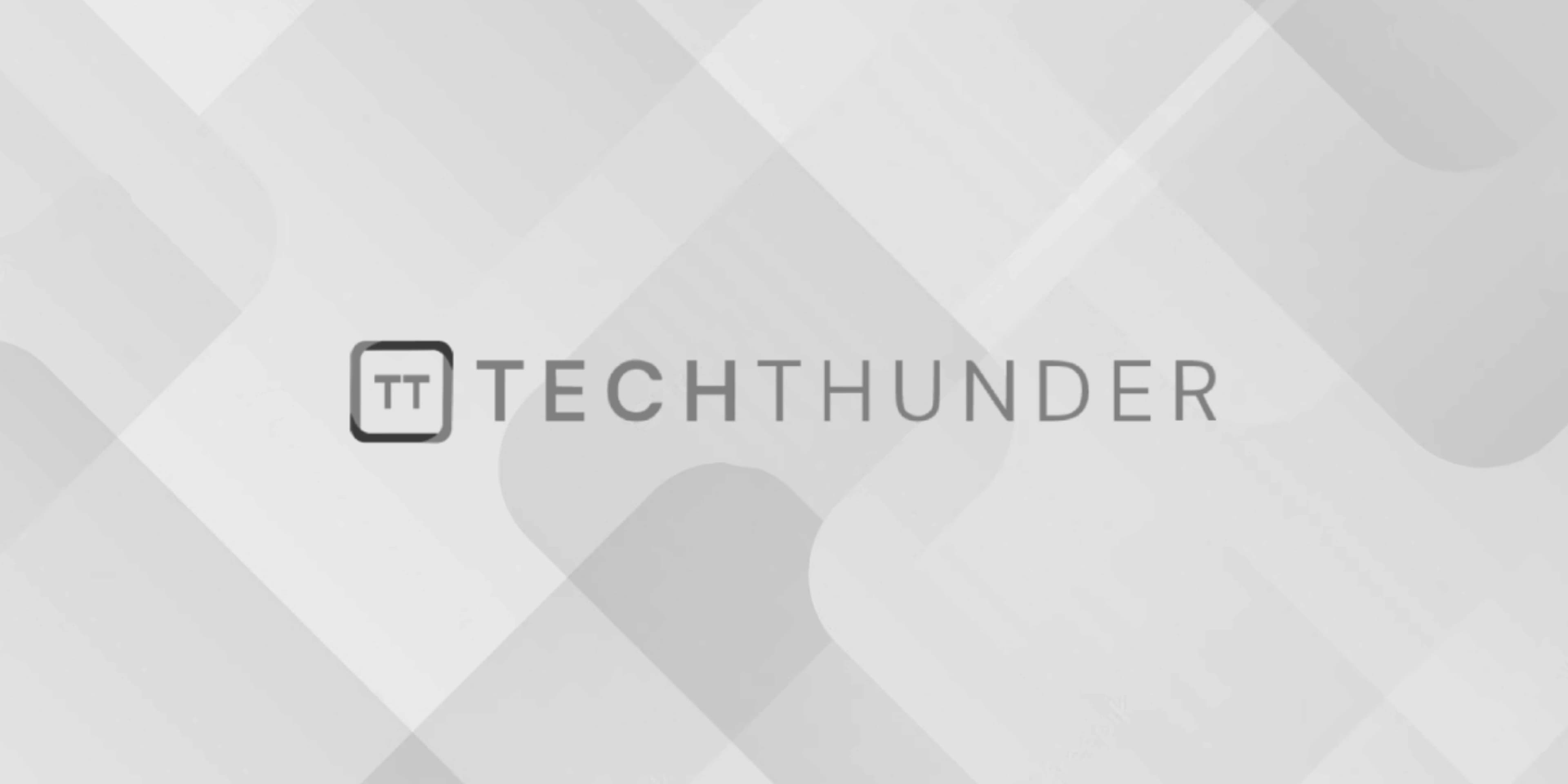
114 views
C++ Break Statement
The C++ break
statement is used to exit a loop prematurely. It is typically used in for
, while
, and do-while
loops to terminate the loop before the loop condition is met.
Here’s an example of using the break
statement in a for
loop:
C++
#include <iostream>
int main() {
for (int i = 1; i <= 10; ++i) {
if (i == 5) {
break; // Exit the loop when i equals 5
}
std::cout << i << " ";
}
std::cout << std::endl;
return 0;
}
Output:
Plaintext
1 2 3 4
In this example, when i
equals 5, the break
statement is executed, causing the loop to terminate immediately.
You can also use break
in while
and do-while
loops:
C++
#include <iostream>
int main() {
int i = 1;
while (i <= 10) {
if (i == 5) {
break; // Exit the loop when i equals 5
}
std::cout << i << " ";
++i;
}
std::cout << std::endl;
return 0;
}
Output:
Plaintext
1 2 3 4
C++
#include <iostream>
int main() {
int i = 1;
do {
if (i == 5) {
break; // Exit the loop when i equals 5
}
std::cout << i << " ";
++i;
} while (i <= 10);
std::cout << std::endl;
return 0;
}
Output:
Plaintext
1 2 3 4
The break
statement is useful when you want to prematurely terminate a loop based on a certain condition, without having to wait for the loop condition itself to evaluate to false.