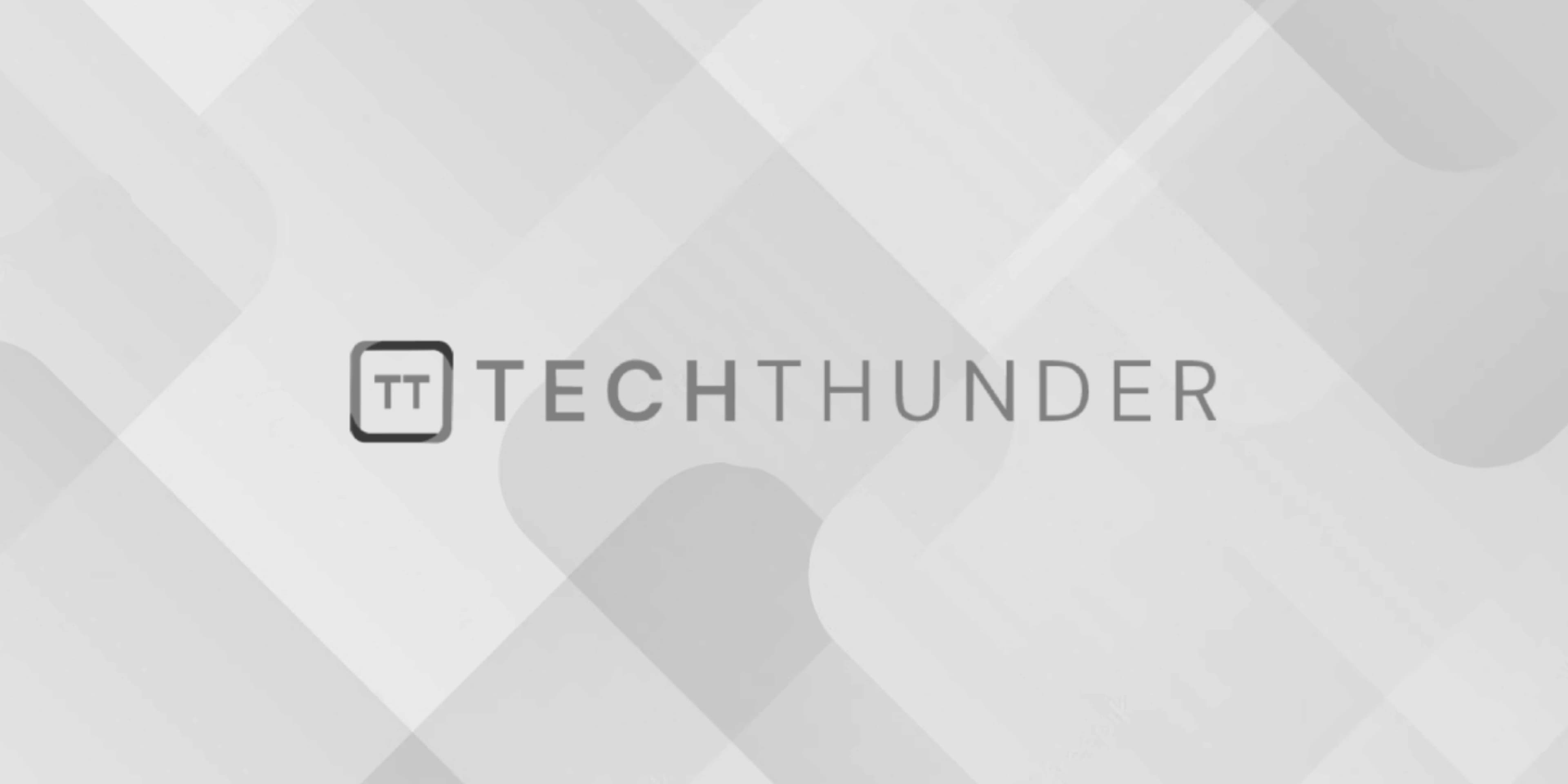
265 views
Bit manipulation C++
Bit manipulation in C++ involves manipulating individual bits within an integer (or other bit-based data types) using bitwise operators. Bit manipulation can be used for a variety of purposes, including setting, clearing, toggling, and checking specific bits within an integer. Here are some common bitwise operators and operations in C++:
- Bitwise AND (
&
) Operator:
- This operator performs a bitwise AND operation between two integers, resulting in a new integer with bits set to 1 only if both corresponding bits in the operands are 1.
C++
int a = 5; // binary: 0101
int b = 3; // binary: 0011
int result = a & b; // binary: 0001 (decimal: 1)
- Bitwise OR (
|
) Operator:
- This operator performs a bitwise OR operation between two integers, resulting in a new integer with bits set to 1 if either of the corresponding bits in the operands is 1.
C++
int a = 5; // binary: 0101
int b = 3; // binary: 0011
int result = a | b; // binary: 0111 (decimal: 7)
- Bitwise XOR (
^
) Operator:
- This operator performs a bitwise XOR (exclusive OR) operation between two integers, resulting in a new integer with bits set to 1 if the corresponding bits in the operands are different.
C++
int a = 5; // binary: 0101
int b = 3; // binary: 0011
int result = a ^ b; // binary: 0110 (decimal: 6)
- Bitwise NOT (
~
) Operator:
- This operator performs a bitwise NOT operation on an integer, flipping all the bits from 0 to 1 and vice versa.
C++
int a = 5; // binary: 0101
int result = ~a; // binary: 1010 (decimal: -6 on most systems due to two's complement representation)
- Left Shift (
<<
) Operator:
- This operator shifts the bits of an integer to the left by a specified number of positions, filling in with 0s on the right.
C++
int a = 5; // binary: 0101
int shifted = a << 2; // binary: 010100 (decimal: 20)
- Right Shift (
>>
) Operator:
- This operator shifts the bits of an integer to the right by a specified number of positions, filling in with 0s on the left for non-negative integers and with the sign bit for negative integers (arithmetic right shift).
C++
int a = 20; // binary: 010100
int shifted = a >> 2; // binary: 000101 (decimal: 5)
Bit manipulation is commonly used in various low-level programming tasks, such as optimizing code, working with hardware, or solving specific algorithmic problems that require precise bit-level control.