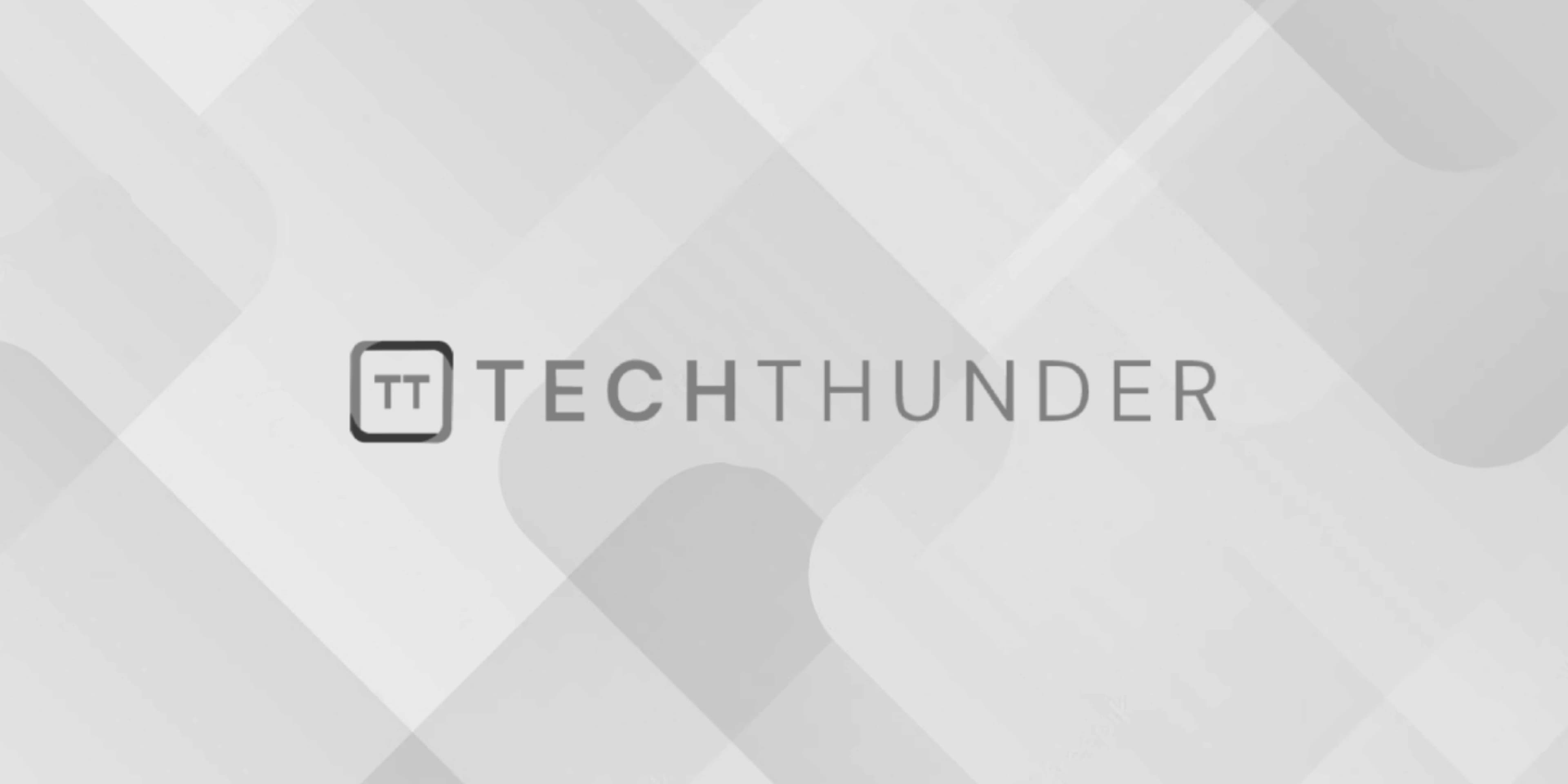
Socket Programming in C++
Socket programming in C++ allows you to create network applications that can communicate over the internet or a local network using sockets. Sockets provide a standard interface for network communication, and C++ provides the necessary libraries to work with sockets. Here’s a basic overview of socket programming in C++:
Socket Types
In socket programming, there are two main types of sockets:
- Server Socket (Listening Socket): This type of socket is used by the server to listen for incoming connections. It waits for client requests and creates a new socket for each incoming connection.
- Client Socket: Client sockets are used by clients to initiate a connection to the server.
Socket Library
To work with sockets in C++, you need to include the <iostream>
, <cstring>
, and <sys/socket.h>
headers. Here’s a basic example of a server and client socket in C++:
Server Socket (Listening Socket) Example
#include <iostream>
#include <cstring>
#include <sys/socket.h>
#include <netinet/in.h>
#include <unistd.h>
int main() {
// Create a socket
int serverSocket = socket(AF_INET, SOCK_STREAM, 0);
if (serverSocket == -1) {
perror("Error creating socket");
return 1;
}
// Bind the socket to an address and port
sockaddr_in serverAddress;
serverAddress.sin_family = AF_INET;
serverAddress.sin_addr.s_addr = INADDR_ANY;
serverAddress.sin_port = htons(8080);
if (bind(serverSocket, (struct sockaddr*)&serverAddress, sizeof(serverAddress)) == -1) {
perror("Error binding socket");
return 1;
}
// Listen for incoming connections
if (listen(serverSocket, 5) == -1) {
perror("Error listening on socket");
return 1;
}
// Accept incoming connections
int clientSocket;
sockaddr_in clientAddress;
socklen_t clientAddrSize = sizeof(clientAddress);
while (true) {
clientSocket = accept(serverSocket, (struct sockaddr*)&clientAddress, &clientAddrSize);
if (clientSocket == -1) {
perror("Error accepting connection");
continue;
}
// Handle the client connection (read/write data)
// ...
close(clientSocket); // Close the client socket
}
close(serverSocket); // Close the server socket
return 0;
}
Client Socket Example
#include <iostream>
#include <cstring>
#include <sys/socket.h>
#include <netinet/in.h>
#include <unistd.h>
int main() {
// Create a socket
int clientSocket = socket(AF_INET, SOCK_STREAM, 0);
if (clientSocket == -1) {
perror("Error creating socket");
return 1;
}
// Connect to the server
sockaddr_in serverAddress;
serverAddress.sin_family = AF_INET;
serverAddress.sin_port = htons(8080);
if (inet_pton(AF_INET, "127.0.0.1", &serverAddress.sin_addr) <= 0) {
perror("Invalid server address");
return 1;
}
if (connect(clientSocket, (struct sockaddr*)&serverAddress, sizeof(serverAddress)) == -1) {
perror("Error connecting to server");
return 1;
}
// Send and receive data with the server
// ...
close(clientSocket); // Close the client socket
return 0;
}
Important Functions and Concepts
socket()
: Creates a socket and returns a socket descriptor.bind()
: Associates a socket with a specific IP address and port number.listen()
: Puts the server socket in a listening state, waiting for incoming connections.accept()
: Accepts an incoming client connection, creating a new socket for communication with the client.connect()
: Initiates a connection to a remote server.send()
andrecv()
: Used for sending and receiving data over the socket.close()
: Closes a socket when you’re done with it.
Please note that error handling and additional code for sending and receiving data are required to create functional server and client applications. Additionally, you may want to explore higher-level networking libraries or frameworks for more complex networking tasks, as raw socket programming can be low-level and error-prone.