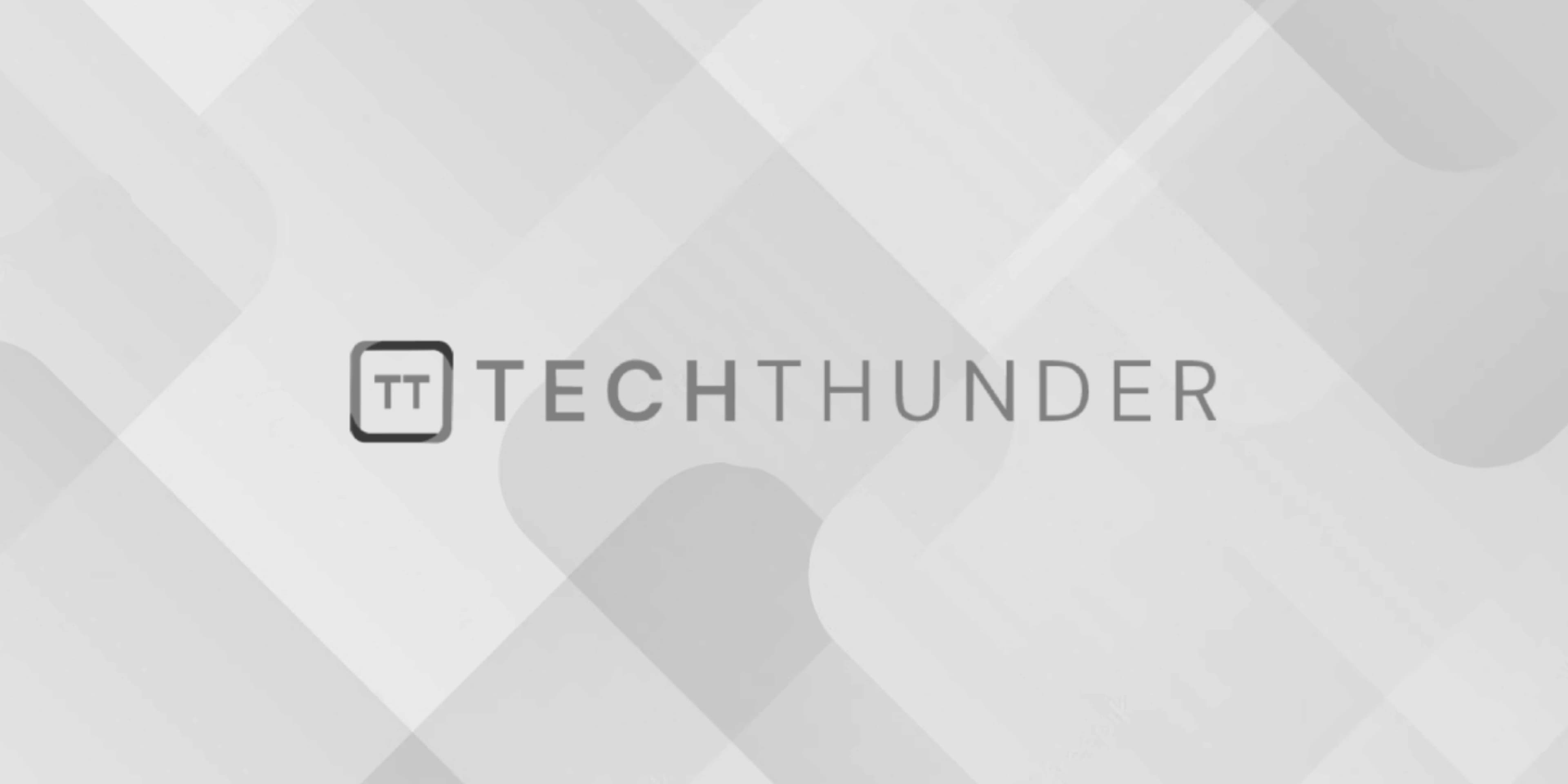
cstdlib in C++
The C++ cstdlib
header (also known as <cstdlib>
) provides a collection of functions that deal with basic C library functionalities, including memory allocation, random number generation, and string conversion. Here are some commonly used functions and features provided by the cstdlib
header:
- Memory Management:
malloc
,calloc
,realloc
: Functions for memory allocation.free
: Function to deallocate memory allocated bymalloc
,calloc
, orrealloc
.
- Random Number Generation:
rand
: Generates a pseudo-random integer between 0 andRAND_MAX
.srand
: Seeds the random number generator with a given value. Note: The random number generation in<cstdlib>
is considered basic and not suitable for cryptographic purposes. If you need higher quality randomness, consider using the<random>
header.
- String Conversion:
atoi
,atol
,atoll
: Functions to convert strings to integers or long integers.strtol
,strtoul
,strtod
: Functions for more flexible string-to-numeric conversions.
- Exit Functions:
exit
: Terminates the program immediately.atexit
: Registers a function to be called at program termination.
- Environment Management:
getenv
: Retrieves the value of an environment variable.system
: Executes a command as if it were a shell command.
- Other Utilities:
abs
,labs
,llabs
: Functions to compute the absolute value of an integer or long integer.div
,ldiv
,lldiv
: Functions to compute quotient and remainder of integer division.
Here’s a simple example demonstrating the use of some functions from <cstdlib>
:
#include <iostream>
#include <cstdlib>
int main() {
int randomValue = rand(); // Generate a random integer
std::cout << "Random value: " << randomValue << std::endl;
char str[] = "12345";
int intValue = atoi(str); // Convert string to integer
std::cout << "Converted value: " << intValue << std::endl;
return 0;
}
Remember to include the <cstdlib>
header at the beginning of your program to use these functions. Keep in mind that many of the functions in this header come from the C standard library, so they might not be the most C++-idiomatic way of performing these tasks. In modern C++ code, you might prefer to use features from the C++ Standard Library or other third-party libraries for more robust and modern functionalities.