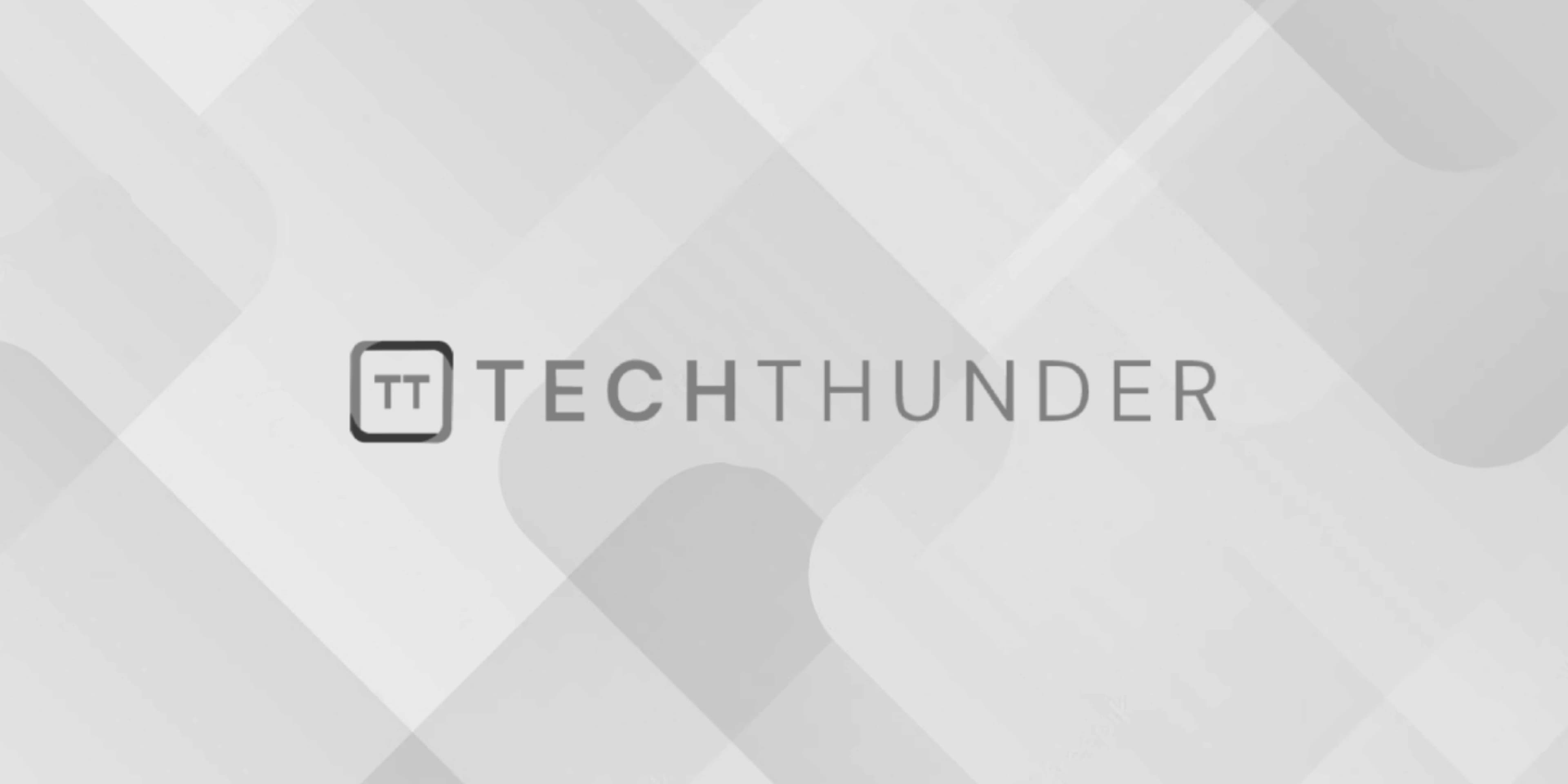
C++ Opaque Pointer
An opaque pointer (also known as an opaque data type or incomplete type) is a design pattern in C++ where the internal implementation details of a class or data structure are hidden from the user of that class or data structure. The user of the class is only provided with a pointer to the type, but they do not have access to the details of the type’s members or implementation. This helps in encapsulation and information hiding, promoting more robust and maintainable code.
The opaque pointer pattern is often implemented using a combination of a forward declaration and a pointer to an incomplete type in a header file. Here’s a simple example:
// MyType.h - Header file
// Forward declaration of the incomplete type
class MyTypeImpl;
// Declaration of the public interface for MyType
class MyType {
public:
MyType(); // Constructor
~MyType(); // Destructor
void doSomething(); // Public member function
private:
MyTypeImpl* pImpl; // Opaque pointer to the incomplete type
};
In this example:
MyTypeImpl
is the incomplete type that contains the private implementation details ofMyType
.MyType
is the public interface class that provides access to the functionality ofMyType
. Users ofMyType
can create instances, call public member functions, and manage the object’s lifetime.
Here’s the implementation of the MyType
class:
// MyType.cpp - Implementation file
#include "MyType.h"
// Definition of the incomplete type MyTypeImpl
class MyTypeImpl {
public:
void doSomethingPrivate() {
// Implementation details here
}
};
MyType::MyType() : pImpl(new MyTypeImpl()) {}
MyType::~MyType() {
delete pImpl;
}
void MyType::doSomething() {
pImpl->doSomethingPrivate(); // Accessing the private implementation
}
In this implementation:
MyTypeImpl
is a separate class containing the private implementation details.- In the constructor of
MyType
, we allocate memory for the private implementation (MyTypeImpl
) and store a pointer to it. - The
doSomething
function ofMyType
can access and call the private functions and members of theMyTypeImpl
class via the opaque pointer.
Using an opaque pointer allows the library or class author to change the internal implementation details of MyType
without affecting users of the class, as long as the public interface remains the same. It provides encapsulation and allows for more flexibility and maintainability in your code.