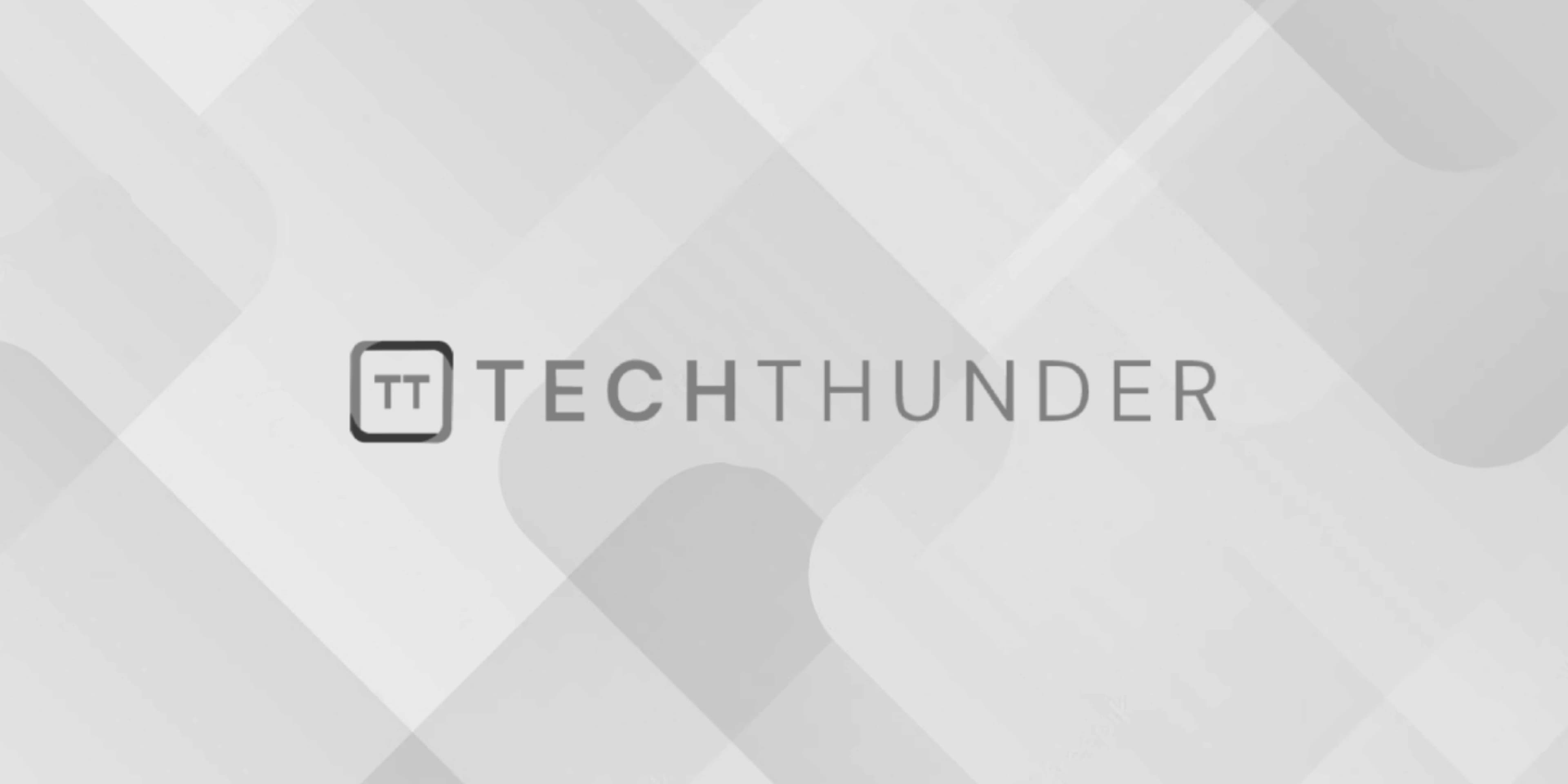
231 views
C++ this Pointer
The this
pointer is a special pointer that is automatically created within the scope of non-static member functions of a class. It points to the instance (object) of the class on which the member function is called. The this
pointer allows member functions to access the data members and member functions of the object to which they belong.
Here are some key points about the this
pointer in C++:
- Pointer to the Current Object: Inside a member function of a class,
this
is a pointer that holds the address of the current object (the object on which the member function is called). It allows you to access the object’s data members and member functions. - Implicit Usage: You don’t need to declare or initialize the
this
pointer explicitly. It is automatically available within the scope of non-static member functions. - Usefulness: The
this
pointer is useful in scenarios where the names of the function parameters or local variables conflict with the names of data members. It helps disambiguate between data members and local variables. - Accessing Members: You can use the
this
pointer to access data members and call member functions of the current object.
Here’s a simple example illustrating the use of the this
pointer:
C++
#include <iostream>
class MyClass {
public:
void setData(int data) {
this->data = data; // Using 'this' to access the data member
}
int getData() const {
return data; // Accessing the data member directly
}
void printAddress() {
std::cout << "Object address: " << this << std::endl;
}
private:
int data;
};
int main() {
MyClass obj1, obj2;
obj1.setData(42);
obj2.setData(99);
std::cout << "Data in obj1: " << obj1.getData() << std::endl;
std::cout << "Data in obj2: " << obj2.getData() << std::endl;
obj1.printAddress();
obj2.printAddress();
return 0;
}
In this example:
- The
setData
member function uses thethis
pointer to assign the value of thedata
member for the current object. - The
getData
member function directly accesses thedata
member. - The
printAddress
member function prints the address of the current object usingthis
.
The this
pointer is especially valuable in larger classes or when dealing with complex class hierarchies, as it helps ensure that the correct object’s members are being accessed and manipulated within member functions.