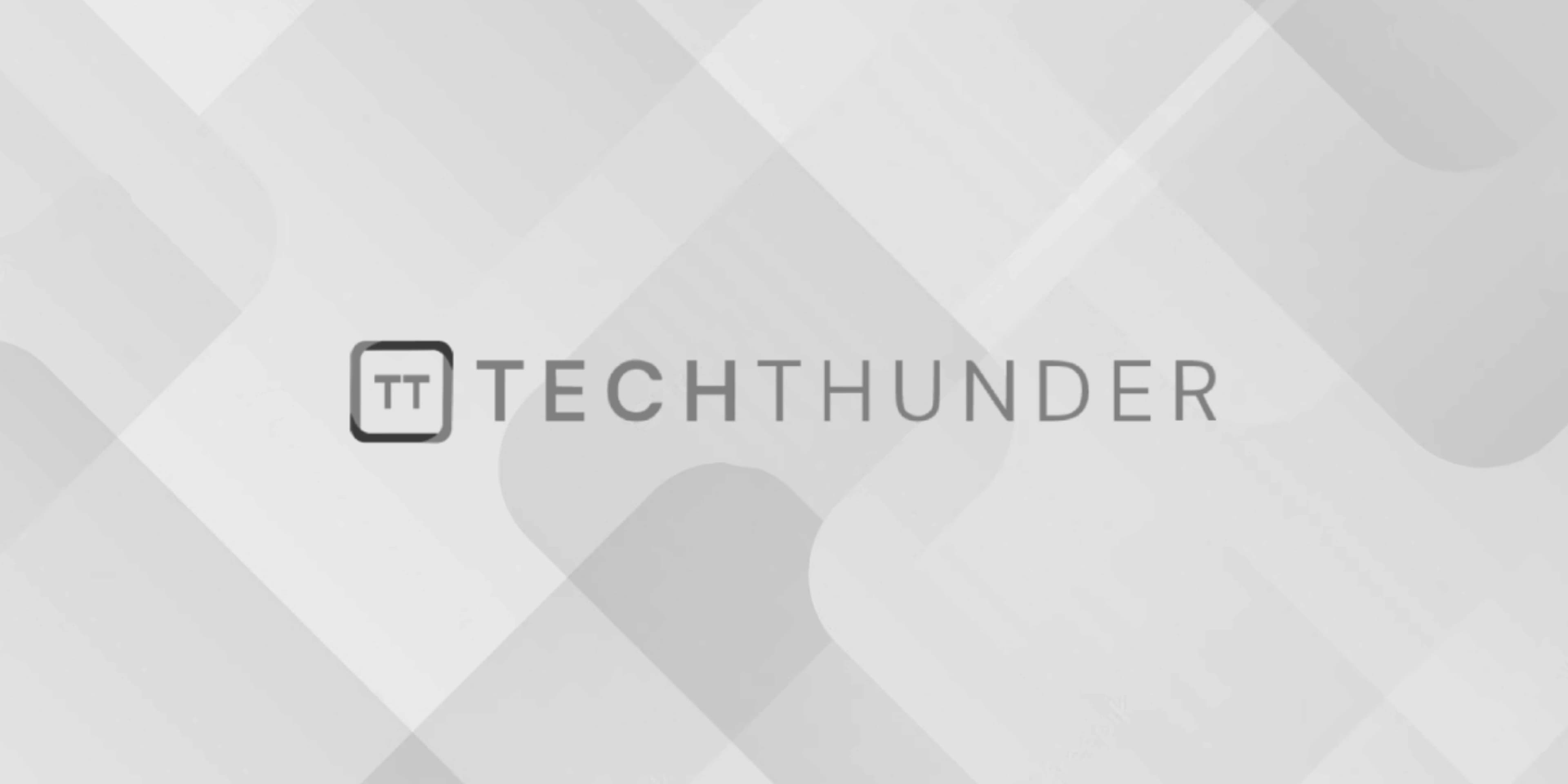
162 views
The distinction between the C++ copy constructor and assignment operator
The C++ copy constructor and assignment operator are two important concepts related to object copying and assignment in classes. They serve distinct purposes and are used in different situations:
- Copy Constructor:
- The copy constructor is a special member function in a class that is called when a new object is created as a copy of an existing object.
- It takes one argument, which is an instance of the same class.
- It is typically used when:
- Creating a new object as a copy of an existing object, such as when passing an object by value to a function or when initializing a new object with an existing one.
- Creating a new object as a return value from a function.
- The copy constructor is invoked automatically by the C++ compiler when needed, and you can provide your own implementation if necessary. Example of a copy constructor:
C++
class MyClass {
public:
// Copy constructor
MyClass(const MyClass& other) {
// Copy member variables from 'other' to 'this'
}
};
MyClass obj1;
MyClass obj2 = obj1; // Calls the copy constructor to create 'obj2' as a copy of 'obj1'
- Assignment Operator (
operator=
):
- The assignment operator is a member function that is used for assigning the values of one object to another object of the same class after both objects have already been created.
- It is used when you want to make an existing object’s data equivalent to another existing object’s data.
- The assignment operator takes one argument, which is an instance of the same class.
- You can provide your own implementation of the assignment operator, which is invoked when you use the assignment operator (
=
) between two objects. Example of an assignment operator:
C++
class MyClass {
public:
// Assignment operator
MyClass& operator=(const MyClass& other) {
if (this == &other) {
return *this; // Handle self-assignment gracefully
}
// Copy member variables from 'other' to 'this'
return *this;
}
};
MyClass obj1;
MyClass obj2;
obj2 = obj1; // Calls the assignment operator to make 'obj2' equivalent to 'obj1'
In summary, the copy constructor is used for creating a new object as a copy of an existing one, while the assignment operator is used for making an existing object equivalent to another existing object. Both are crucial for handling object copying and assignment in C++ classes, and it’s essential to implement them correctly, especially when dealing with dynamic memory allocation or resource management.