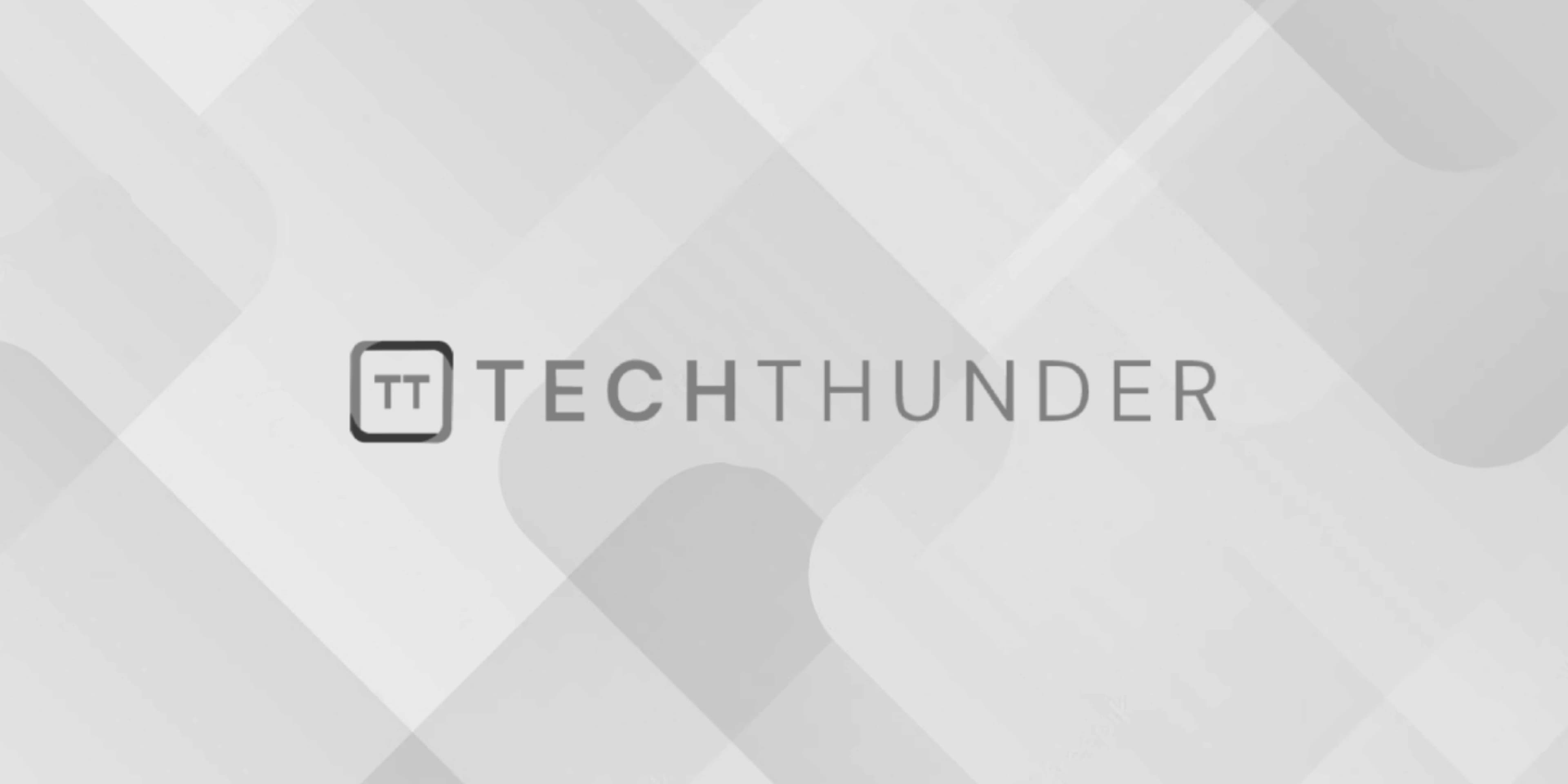
146 views
C++ class to prevent object copies
The C++, if you wao prevent object copies of a class, you can achieve this by declaring a private copy constructor and a private copy assignment operator (operator=). By making these members private, you prevent other code from making copies of objects of that class. This is often referred to as disabling or deleting the copy constructor and copy assignment operator. Here’s an example:
C++
class NoCopyClass {
public:
// Public constructor
NoCopyClass(int value) : data(value) {}
// Public member function
void printData() const {
std::cout << "Data: " << data << std::endl;
}
private:
int data;
// Private copy constructor (disabled)
NoCopyClass(const NoCopyClass& other) = delete;
// Private copy assignment operator (disabled)
NoCopyClass& operator=(const NoCopyClass& other) = delete;
};
int main() {
NoCopyClass obj1(42);
obj1.printData();
// Attempting to create a copy will result in a compilation error
// NoCopyClass obj2 = obj1; // Error: use of deleted function
return 0;
}
In this example:
- We define a class called
NoCopyClass
with a privatedata
member and a public constructor and member function. - We make the copy constructor and copy assignment operator private and use the
= delete
syntax to explicitly disable them. - In the
main
function, we create an objectobj1
ofNoCopyClass
and use its public member function. - If you attempt to create a copy of
obj1
, likeNoCopyClass obj2 = obj1;
, it will result in a compilation error due to the deleted copy constructor and copy assignment operator.
By disabling the copy operations in this manner, you ensure that objects of the NoCopyClass
cannot be copied, enforcing a design choice that prevents unintentional object copies.