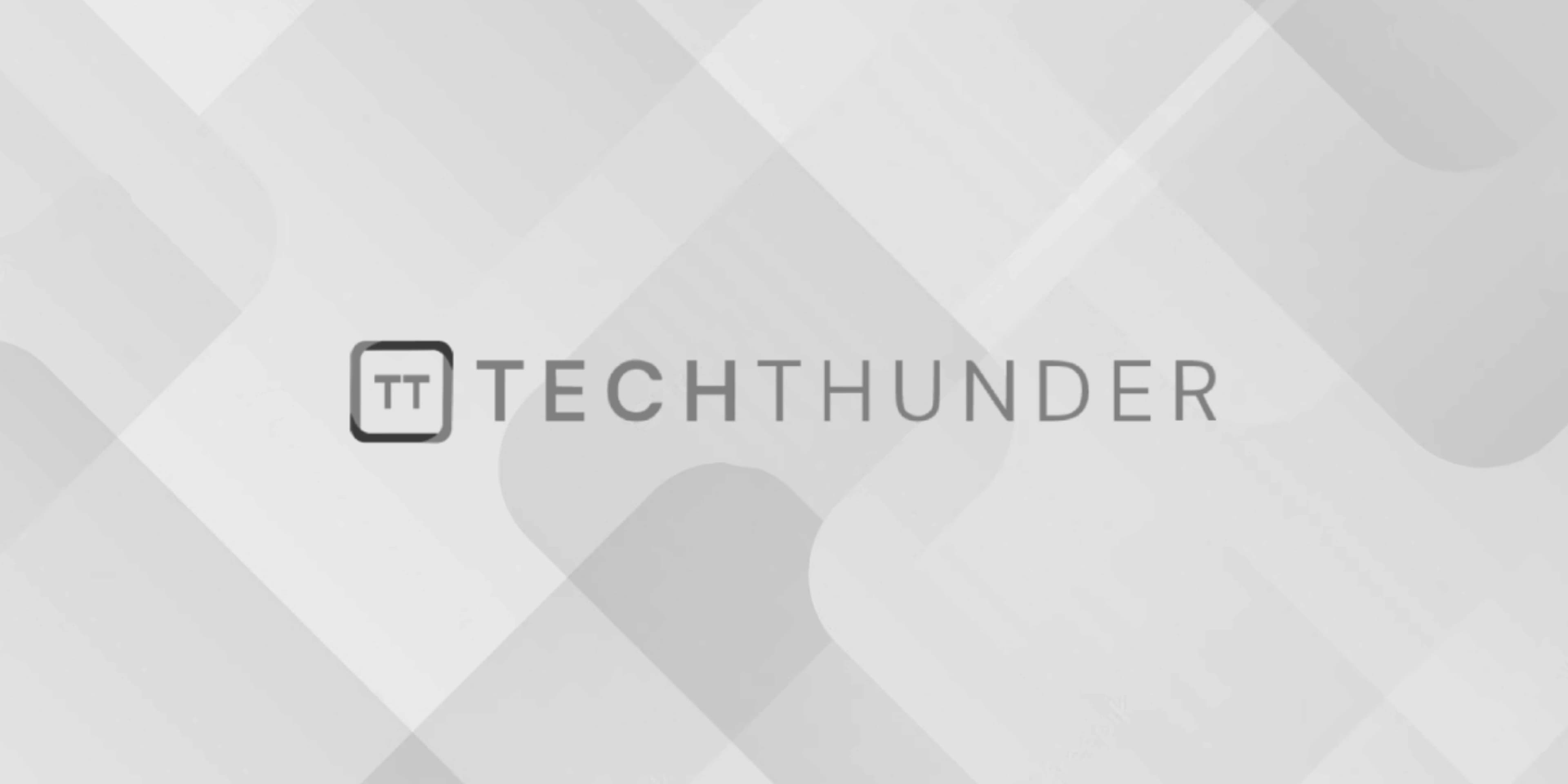
172 views
Armstrong Number in C++
An Armstrong number (also known as a narcissistic number or a pluperfect digital invariant) is a number that is equal to the sum of its own digits each raised to the power of the number of digits. In other words, an n-digit number is an Armstrong number if the sum of its digits, each raised to the nth power, is equal to the number itself.
Here’s a C++ program to check if a given number is an Armstrong number:
C++
#include <iostream>
#include <cmath>
int main() {
int number, originalNumber, remainder, result = 0, n;
// Input a number
std::cout << "Enter an integer: ";
std::cin >> number;
originalNumber = number;
// Calculate the number of digits in the number
n = static_cast<int>(log10(number)) + 1;
// Check if it's an Armstrong number
while (originalNumber != 0) {
remainder = originalNumber % 10;
result += pow(remainder, n);
originalNumber /= 10;
}
if (result == number) {
std::cout << number << " is an Armstrong number." << std::endl;
} else {
std::cout << number << " is not an Armstrong number." << std::endl;
}
return 0;
}
In this program:
- The user is prompted to enter an integer.
- The program calculates the number of digits in the given number using
log10(number) + 1
. - It then enters a loop to extract the digits of the number one by one, raise each digit to the power of
n
, and add the result toresult
. - Finally, it checks if
result
is equal to the original number. If they are equal, the number is an Armstrong number; otherwise, it’s not.
Compile and run this program to check if a given number is an Armstrong number.