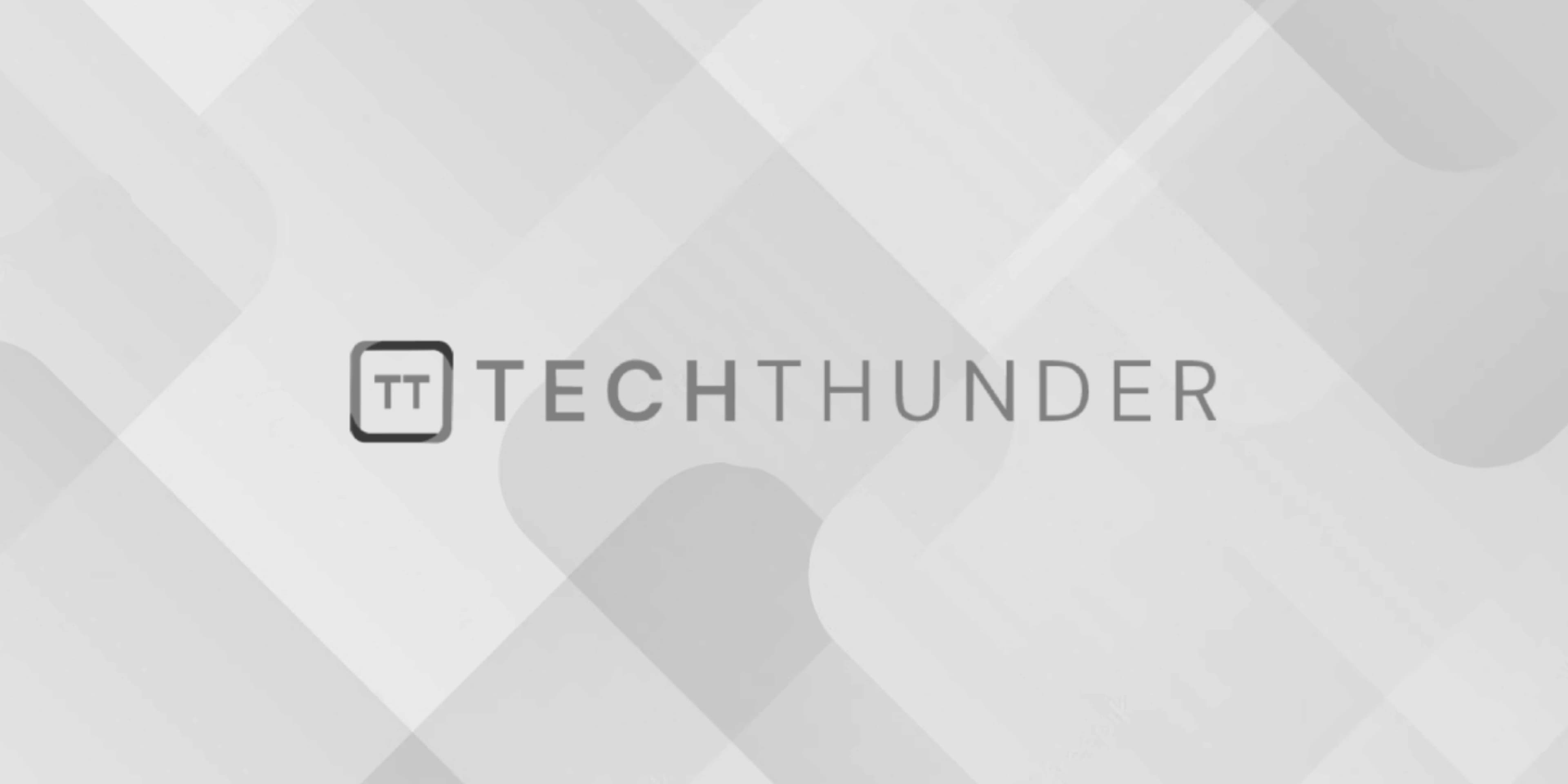
Upcasting and Downcasting in C++
Upcasting and downcasting are two fundamental concepts in C++ related to inheritance and working with class hierarchies. They allow you to convert pointers or references to base and derived classes and vice versa.
- Upcasting: Upcasting involves converting a pointer or reference from a derived class to a base class. It’s a safe and implicit conversion because the derived class object contains all the members of the base class.
class Base {
// Base class members
};
class Derived : public Base {
// Derived class members
};
int main() {
Derived derivedObj;
Base* basePtr = &derivedObj; // Upcasting
return 0;
}
In the example above, we have upcasted a pointer from Derived
to Base
. This allows you to access the base class members through basePtr
.
- Downcasting: Downcasting involves converting a pointer or reference from a base class to a derived class. It’s a potentially unsafe operation because the base class might not have all the information needed for the derived class.
class Base {
// Base class members
};
class Derived : public Base {
// Derived class members
};
int main() {
Base baseObj;
Derived* derivedPtr = dynamic_cast<Derived*>(&baseObj); // Downcasting
if (derivedPtr) {
// Successfully downcasted
// Access derived class members through derivedPtr
} else {
// Downcast failed
}
return 0;
}
In this example, we have attempted to downcast a pointer from Base
to Derived
using dynamic_cast
. dynamic_cast
performs a runtime check to ensure that the downcast is valid. If the downcast is valid, it returns a pointer to the derived class; otherwise, it returns nullptr.
Using dynamic_cast
helps prevent invalid downcasting and provides a safe way to perform this operation. It is typically used in situations where you need to work with a base class pointer or reference but want to access derived class-specific functionality if available.
Note that downcasting without dynamic_cast
can be dangerous and should be avoided unless you are absolutely certain about the object’s actual type.
Upcasting and downcasting are essential for working with class hierarchies and implementing polymorphism in C++. However, it’s crucial to use downcasting with caution, validate it when necessary, and consider alternative design patterns when frequent downcasting is required.