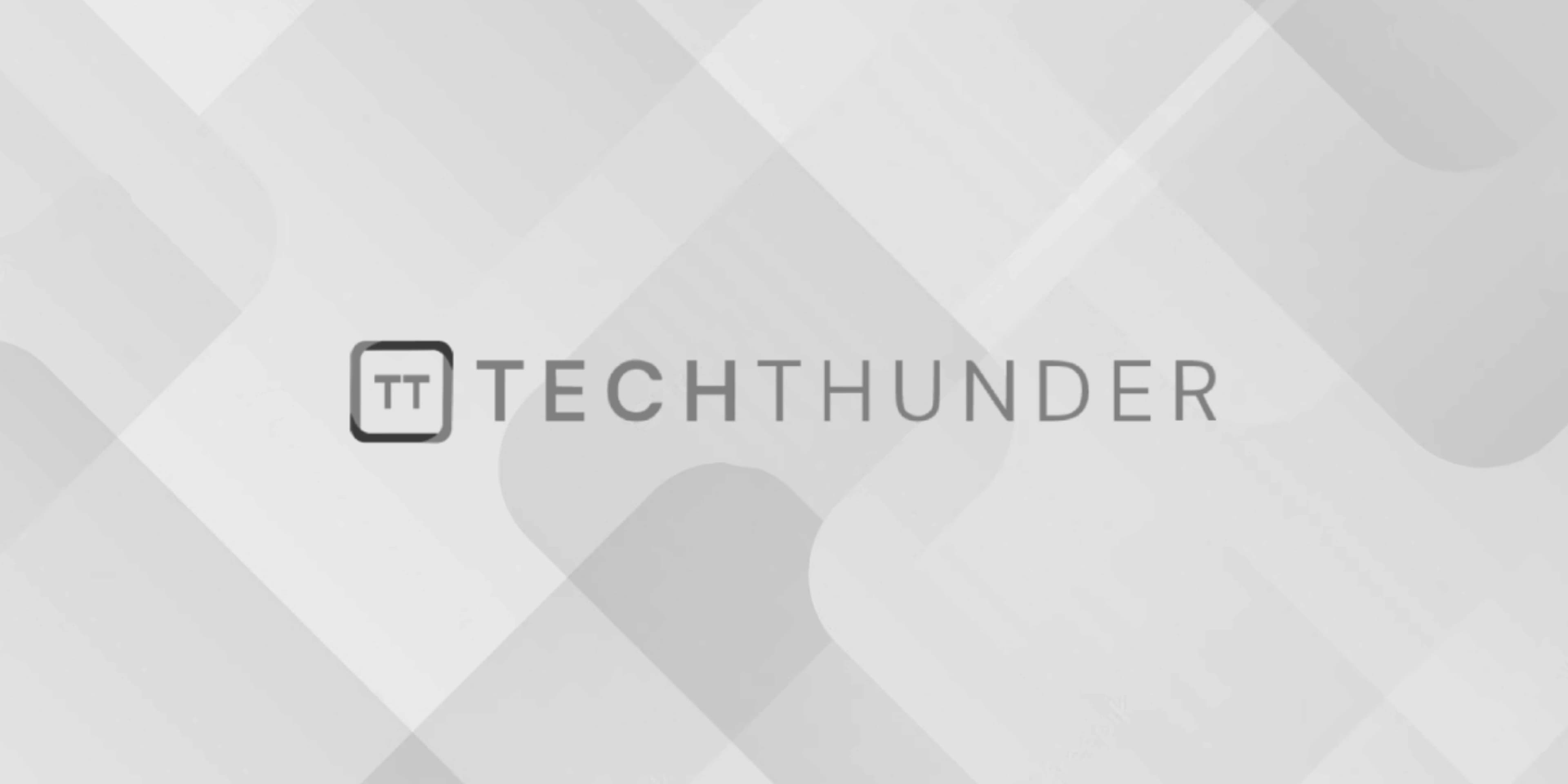
184 views
Adding Two Objects in C++
The C++can add two objects of a user-defined class by defining an appropriate addition operator (operator+
) for that class. Here’s an example of how to add two objects of a custom class:
C++
#include <iostream>
class MyClass {
public:
MyClass(int value) : data(value) {}
// Overload the addition operator (+) for adding two objects
MyClass operator+(const MyClass& other) const {
return MyClass(data + other.data);
}
void display() const {
std::cout << "Data: " << data << std::endl;
}
private:
int data;
};
int main() {
MyClass obj1(5);
MyClass obj2(10);
MyClass result = obj1 + obj2; // Add two objects
std::cout << "Result of addition: ";
result.display();
return 0;
}
In this example:
- We define a class called
MyClass
with a private integer memberdata
, a constructor to initialize it, and adisplay
member function to print the value. - We overload the
+
operator by defining theoperator+
member function. This function takes a constant reference to anotherMyClass
object and returns a newMyClass
object containing the sum of thedata
members of the two objects. - In the
main
function, we create twoMyClass
objects,obj1
andobj2
, and then add them together using the+
operator, resulting in a newMyClass
object,result
. - Finally, we display the result using the
display
member function.
When you run this program, it will add the data
members of obj1
and obj2
and display the result, which should be 15
in this case. You can customize the class and the addition operation to suit your specific needs.