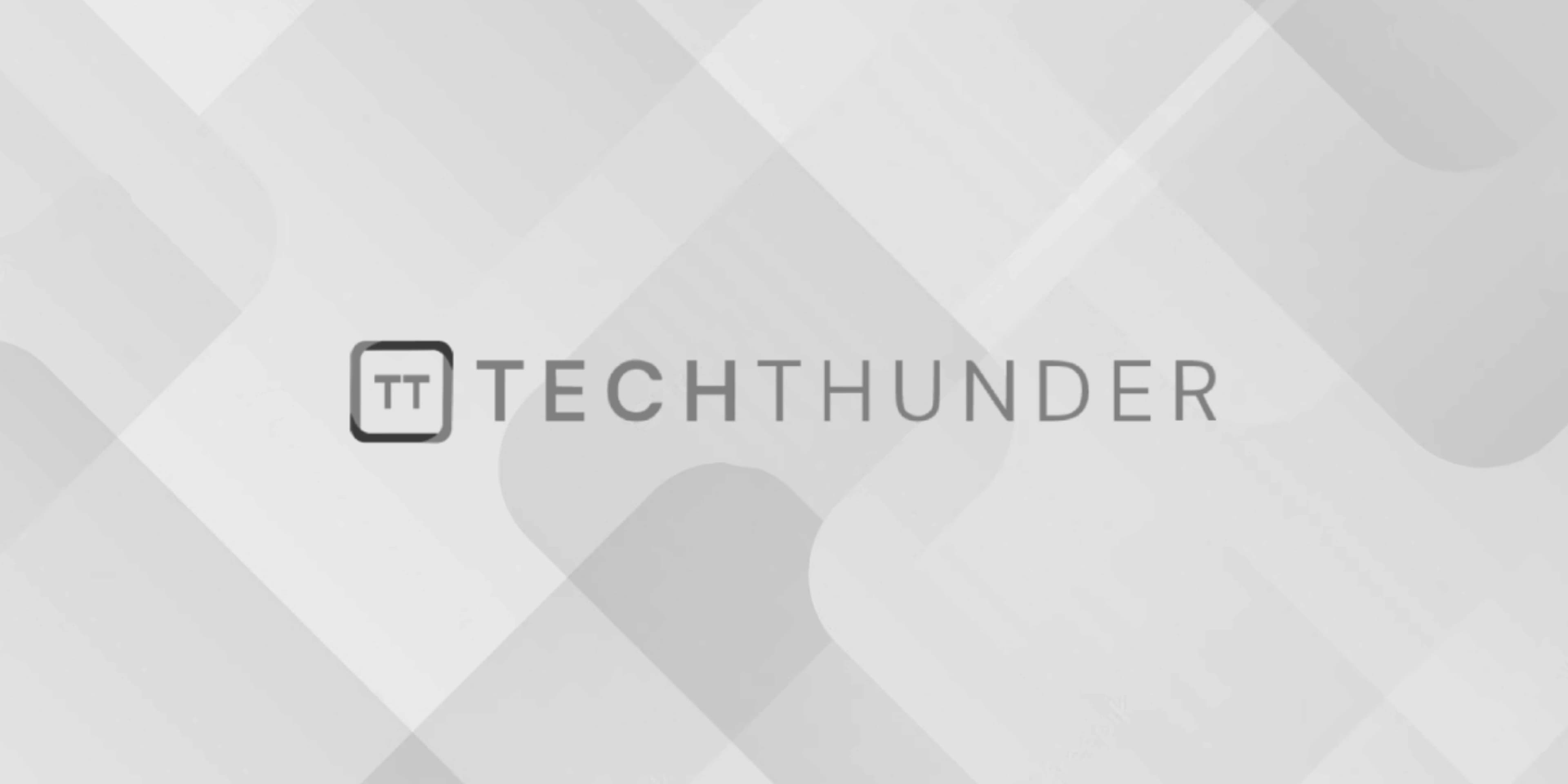
Function Pointer in C++
The C++ function pointer is a variable that stores the address of a function. Function pointers allow you to call functions dynamically at runtime, which can be useful in various scenarios, such as implementing callback mechanisms, creating function tables, or implementing polymorphism. Understanding function pointers is important for advanced C++ programming.
Here’s how you can declare, initialize, and use function pointers in C++:
Declaration of Function Pointers:
The syntax for declaring a function pointer is as follows:
return_type (*pointer_name)(parameter_type1, parameter_type2, ...);
return_type
is the return type of the function.pointer_name
is the name of the function pointer.parameter_type1
,parameter_type2
, etc., are the types of parameters the function takes.
For example:
int (*add)(int, int);
This declares a function pointer named add
that can point to a function taking two int
parameters and returning an int
.
Initialization of Function Pointers:
Function pointers can be initialized with the address of a function that matches their signature. You can also initialize them to nullptr
if they are not immediately needed.
int addFunc(int a, int b) {
return a + b;
}
int subtractFunc(int a, int b) {
return a - b;
}
int (*operation)(int, int); // Declaration of function pointer
operation = &addFunc; // Initialize with address of addFunc
// Alternatively: operation = addFunc;
// Call the function through the pointer
int result = operation(5, 3); // Calls addFunc(5, 3)
operation = &subtractFunc; // Change the pointer to point to subtractFunc
result = operation(5, 3); // Calls subtractFunc(5, 3)
Using Function Pointers:
You can use function pointers to call functions dynamically, select functions at runtime, or pass functions as arguments to other functions.
int operate(int a, int b, int (*func)(int, int)) {
return func(a, b);
}
int result1 = operate(5, 3, addFunc); // Calls addFunc(5, 3)
int result2 = operate(5, 3, subtractFunc); // Calls subtractFunc(5, 3)
Function pointers are particularly useful in scenarios where you want to implement callback functions, create plugin architectures, or implement design patterns like the Strategy Pattern, where different algorithms can be selected at runtime.
Keep in mind that function pointers must match the signature (return type and parameter types) of the functions they point to; otherwise, you may encounter undefined behavior. Additionally, be cautious about function pointer typecasting, as it can lead to issues if not done correctly.