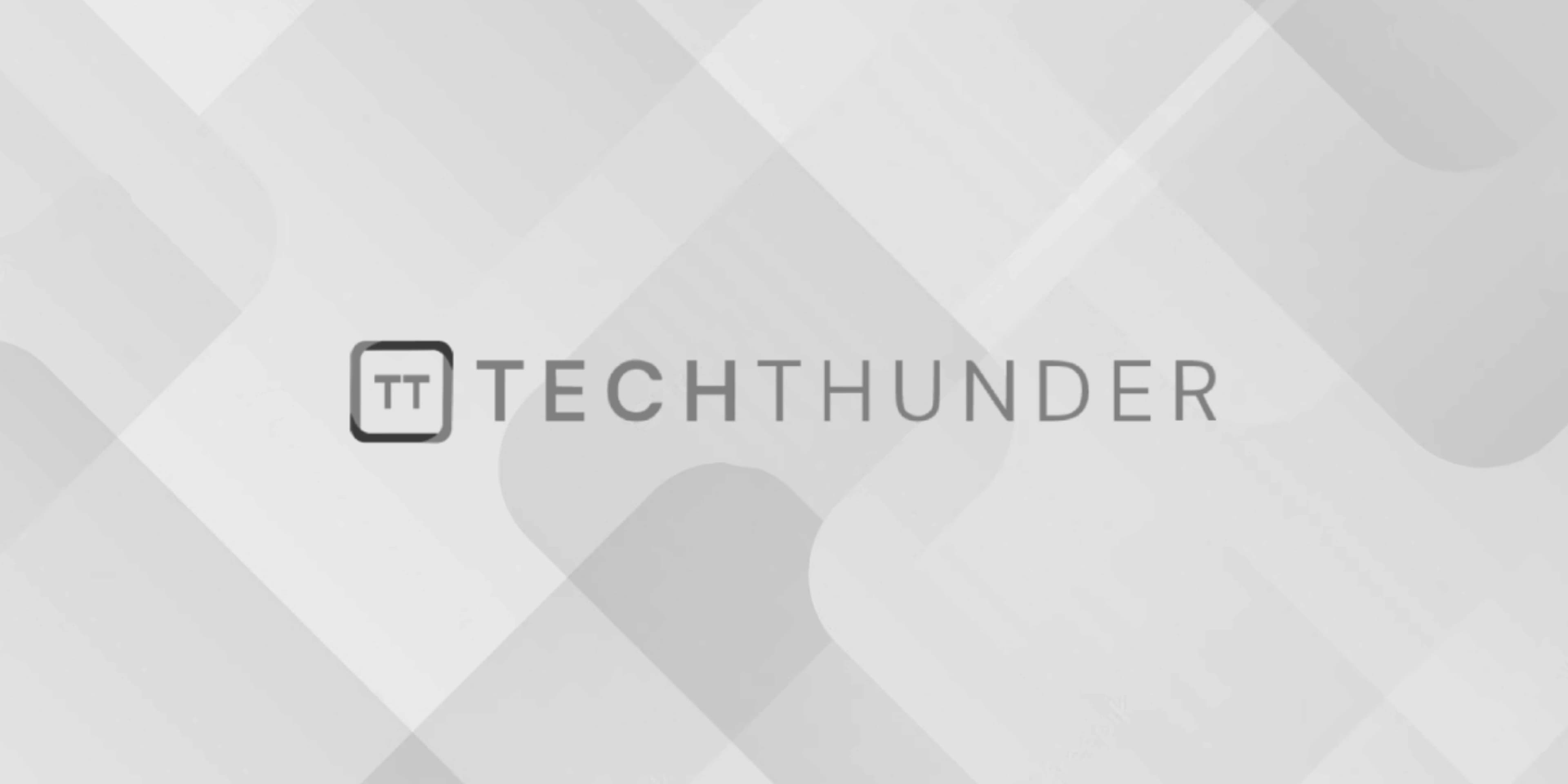
C++ Copy Constructor
The C++ copy constructor is a special member function that creates a new object by copying the values of another object of the same class. It is invoked when an object is being copied, whether it’s being passed by value, returned by value, or explicitly copied using the copy constructor. The copy constructor is often used to perform a deep copy of the object, ensuring that the copied object is a separate instance with its own memory.
Here’s how you define and use a copy constructor:
class MyClass {
public:
int data;
// Copy constructor declaration
MyClass(const MyClass& other) {
data = other.data;
}
};
In this example, we’ve defined a copy constructor for the MyClass
class that takes a reference to another MyClass
object (const MyClass& other
) and initializes the data
member of the current object with the data
member of the other object.
Here’s how you use the copy constructor:
int main() {
MyClass obj1;
obj1.data = 42;
// Using the copy constructor to create obj2 as a copy of obj1
MyClass obj2 = obj1;
// obj1 and obj2 now have the same data value
std::cout << "obj1.data: " << obj1.data << std::endl;
std::cout << "obj2.data: " << obj2.data << std::endl;
return 0;
}
In this main
function, we create two objects, obj1
and obj2
. We use the copy constructor to initialize obj2
as a copy of obj1
. As a result, both objects have the same data
value.
Keep in mind the following important points about copy constructors:
- Default Copy Constructor: If you don’t define a copy constructor for your class, C++ provides a default copy constructor that performs a shallow copy (i.e., it copies the values of data members). However, for classes with dynamically allocated memory or resources, a deep copy may be required, and you should provide a custom copy constructor.
- Rule of Three (Rule of Five in C++11 and later): When you define a custom copy constructor, it’s often necessary to define a custom destructor and copy assignment operator (and move constructor and move assignment operator in C++11 and later) to manage resources properly. This is known as the Rule of Three (Rule of Five).
- Passing Objects by Value: When you pass an object by value to a function or return it by value from a function, the copy constructor is invoked. This can impact performance, especially for large objects, so it’s essential to be aware of copy operations in your code.
- Const-Correctness: It’s a good practice to make the copy constructor parameter a constant reference (
const MyClass&
) to ensure that the source object is not modified during the copy operation.
Copy constructors are an important part of C++ class design and are used to manage the proper copying and initialization of objects.