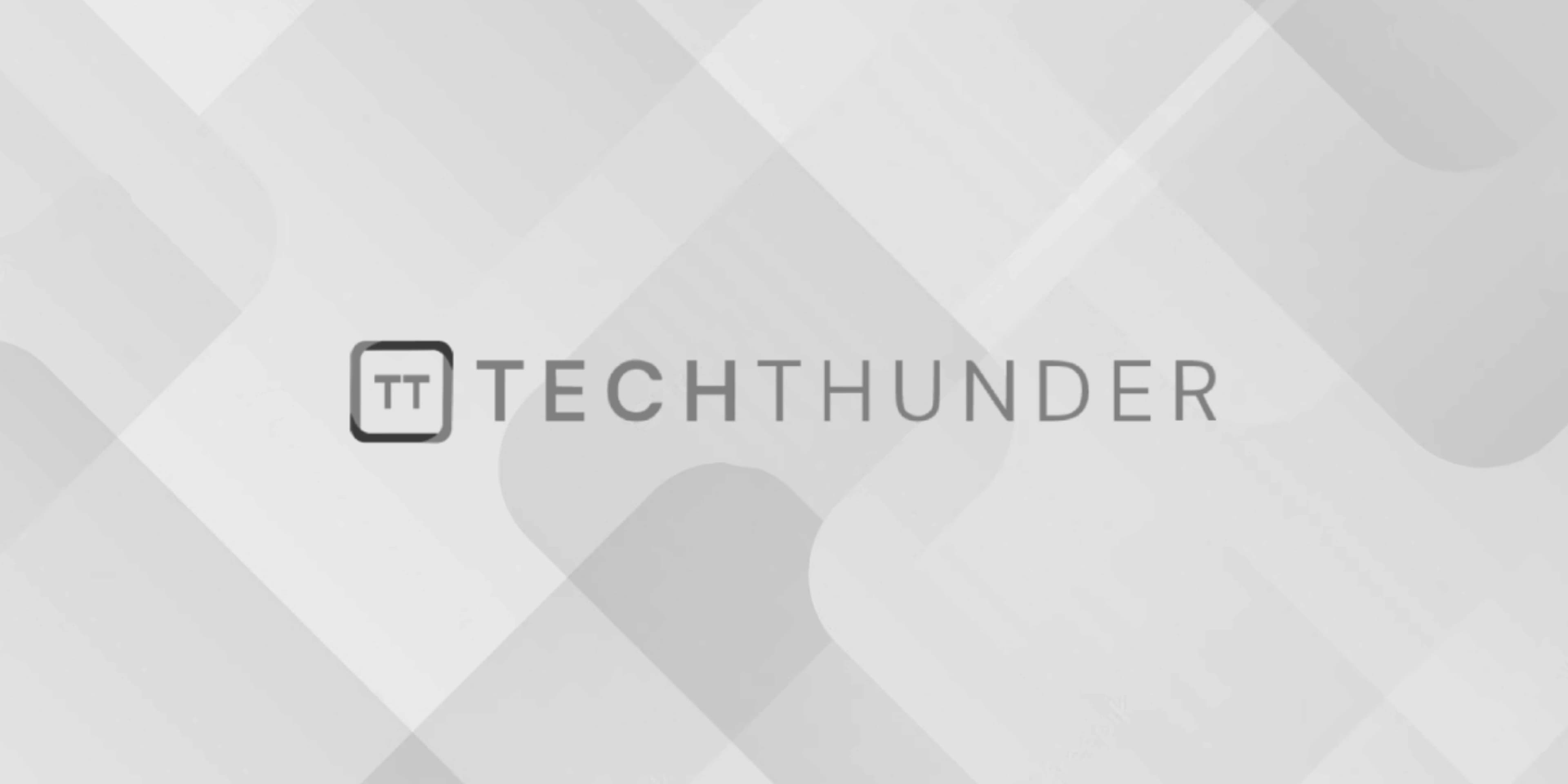
C++ getline()
The C++ getline()
is a commonly used function for reading a line of text from an input stream, such as the standard input (keyboard) or a file. It is a member function of the std::istream
class and is often used to read user input.
Here’s the basic syntax of getline()
:
#include <iostream>
#include <string>
int main() {
std::string line;
std::cout << "Enter a line of text: ";
std::getline(std::cin, line); // Read a line of text from standard input
std::cout << "You entered: " << line << std::endl;
return 0;
}
In this example:
- We include the necessary header files for
iostream
andstring
. - We declare a
std::string
variable namedline
to store the input. - We prompt the user to enter a line of text using
std::cout
. - We use
std::getline(std::cin, line)
to read a line of text from the standard input (keyboard) and store it in theline
variable.std::cin
is the standard input stream. - Finally, we print out the line of text that was entered.
getline()
reads characters from the input stream until it encounters a newline character (‘\n’) or reaches the end of the stream. It stores the read characters, including the newline character, in the string variable, which allows you to read entire lines of text.
Here’s how getline()
works:
- It reads characters from the input stream until it encounters a newline character (‘\n’) or the end of the stream.
- It stores the characters, including the newline character, in the specified string.
- The newline character (‘\n’) is removed from the input stream, but it is included in the string.
This function is useful for reading user input because it can handle input with spaces and doesn’t stop reading at the first whitespace character. It reads until a newline character is encountered, which means it can read entire lines of text, even if they contain spaces.
You can also use getline()
to read lines from files by replacing std::cin
with a file input stream (std::ifstream
).