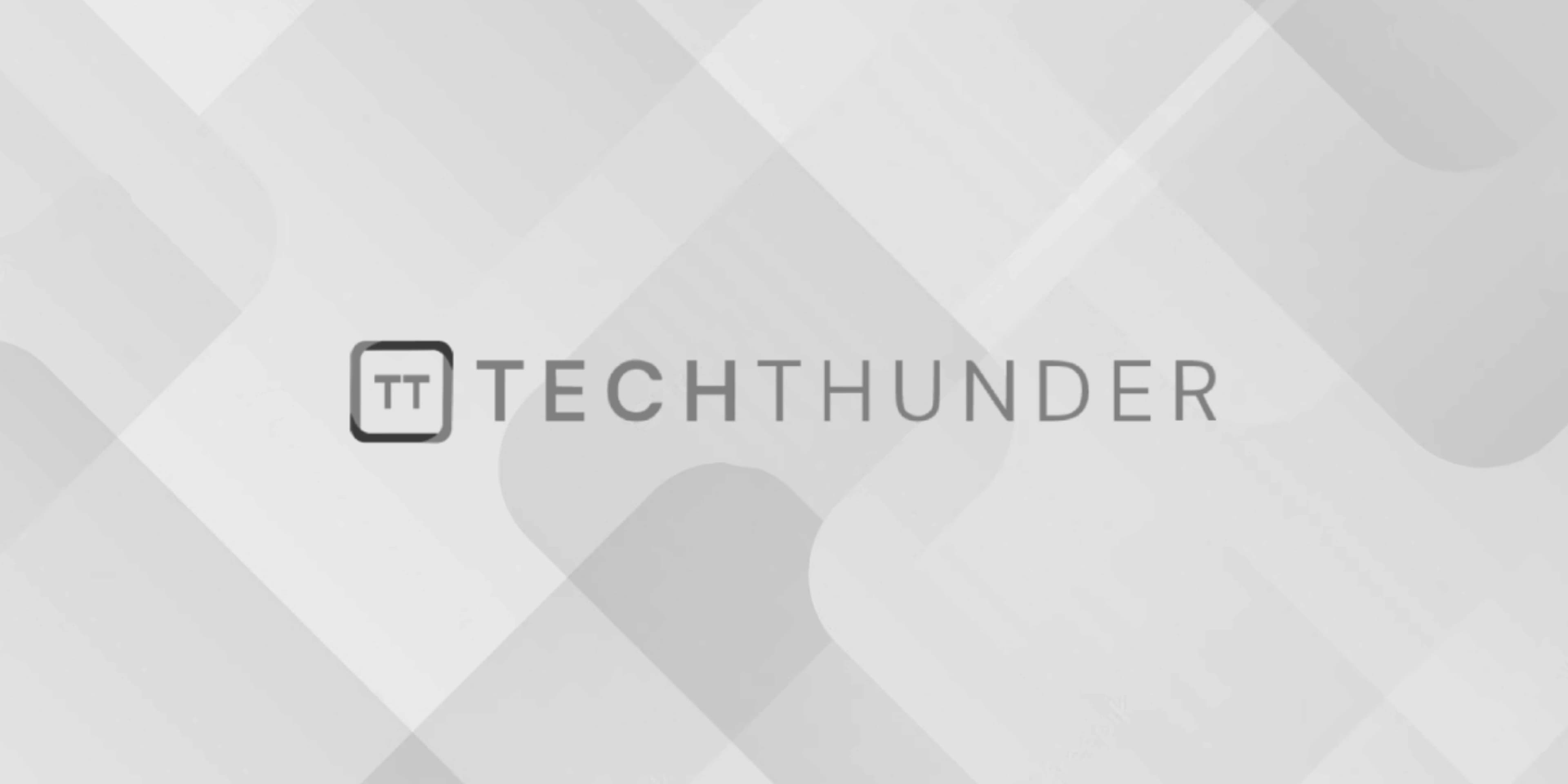
C++ Output Iterator
The output iterator in C++ is an iterator that allows you to write data into a container or stream. Output iterators are part of the C++ Standard Library’s iterator category hierarchy, and they are commonly used to perform output operations on containers like vectors, arrays, and streams like files and standard output.
Output iterators are primarily used with algorithms that write values to a sequence or a stream, such as the std::copy
algorithm. They provide a way to abstract away the underlying container or stream, allowing you to write data to different types of destinations using the same algorithm.
Here’s an example of using an output iterator with the std::copy
algorithm to copy data from one container to another:
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> source = {1, 2, 3, 4, 5};
std::vector<int> destination;
// Using an output iterator to copy data from source to destination
std::copy(source.begin(), source.end(), std::back_inserter(destination));
// Print the elements in the destination vector
for (const int& value : destination) {
std::cout << value << " ";
}
std::cout << std::endl;
return 0;
}
In this example:
std::back_inserter(destination)
returns an output iterator that appends elements to thedestination
vector.- The
std::copy
algorithm copies elements fromsource
todestination
using the output iterator returned bystd::back_inserter
. - As a result, the
destination
vector contains the same elements as thesource
vector.
Output iterators are typically used with algorithms that expect an output iterator as an argument, such as std::copy
, std::fill
, and others. They provide a convenient way to abstract away the details of writing data into different types of containers or streams, making your code more versatile and reusable.