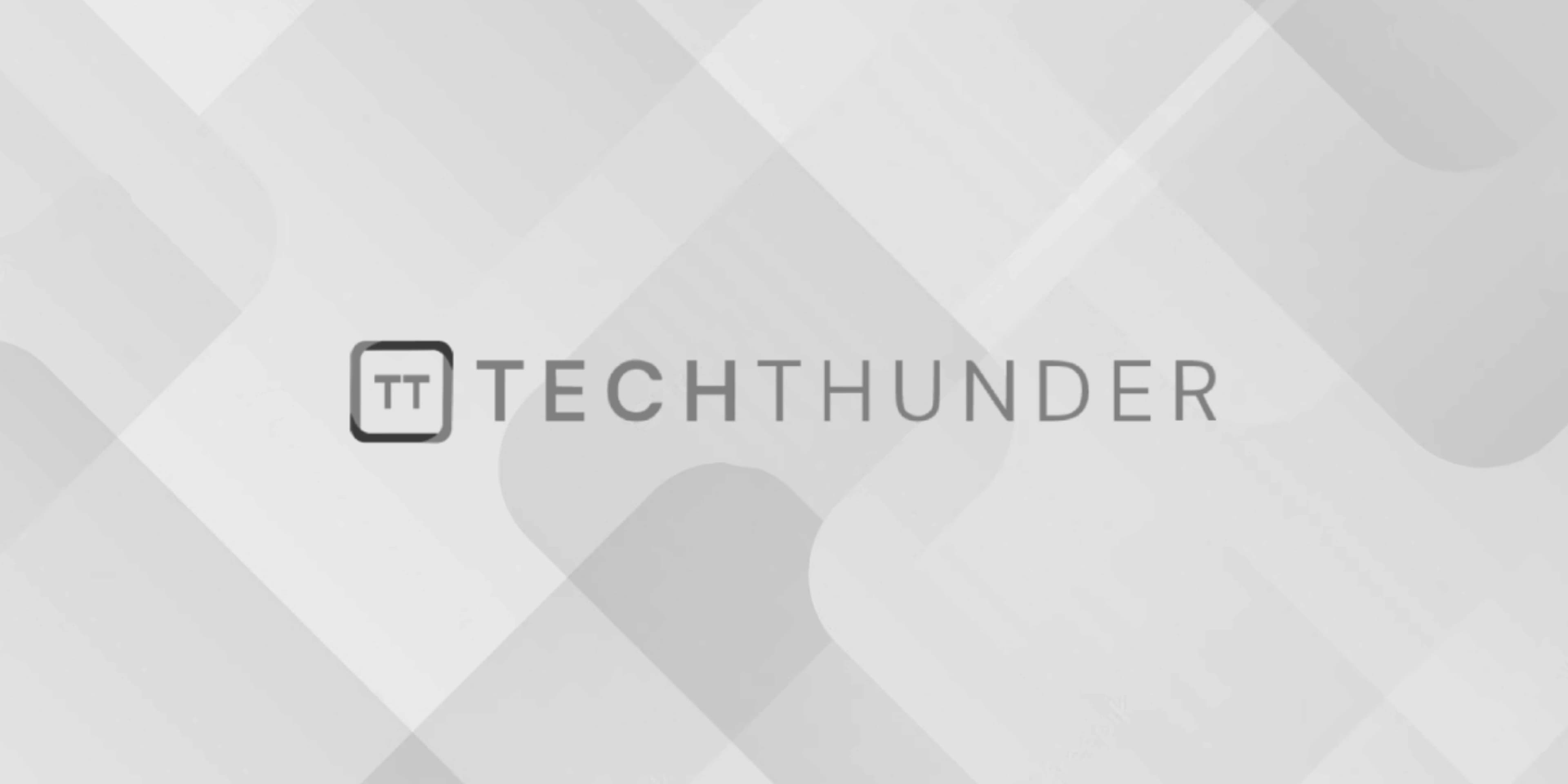
Actual Argument and Formal Argument in C++
The C++ “actual arguments” and “formal arguments” are terms used to describe the values or expressions passed to a function when it’s called (actual arguments) and the parameters declared in the function’s definition (formal arguments) that receive these values. These terms are often used interchangeably with “actual parameters” and “formal parameters.”
Here’s a breakdown of these concepts:
- Formal Arguments (Formal Parameters or Formal Parameters):
- Formal arguments are the parameters declared in a function’s parameter list within its definition.
- They serve as placeholders for the values that will be passed to the function when it is called.
- Formal arguments have names, data types, and possibly default values specified in the function’s signature.
void exampleFunction(int formalParam1, double formalParam2) {
// 'formalParam1' and 'formalParam2' are formal arguments (parameters)
}
- Actual Arguments (Actual Parameters or Actual Parameters):
- Actual arguments are the values or expressions provided in a function call when invoking the function.
- They correspond to the formal arguments declared in the function’s definition.
- Actual arguments are also referred to as actual parameters or actual parameters.
- These values or expressions are passed to the function and assigned to the formal arguments for use within the function’s body.
int actualArg1 = 5;
double actualArg2 = 3.14;
exampleFunction(actualArg1, actualArg2); // 'actualArg1' and 'actualArg2' are actual arguments (parameters)
In the example above, int formalParam1
and double formalParam2
in the exampleFunction
function are formal arguments. When the function is called with actualArg1
and actualArg2
as arguments, actualArg1
corresponds to int formalParam1
(actual argument for formalParam1
), and actualArg2
corresponds to double formalParam2
(actual argument for formalParam2
).
The purpose of distinguishing formal and actual arguments is to allow functions to work with data passed from the calling code dynamically. It allows you to reuse the same function with different input values without having to rewrite the function’s code. Understanding these concepts is fundamental when working with functions and function calls in C++.