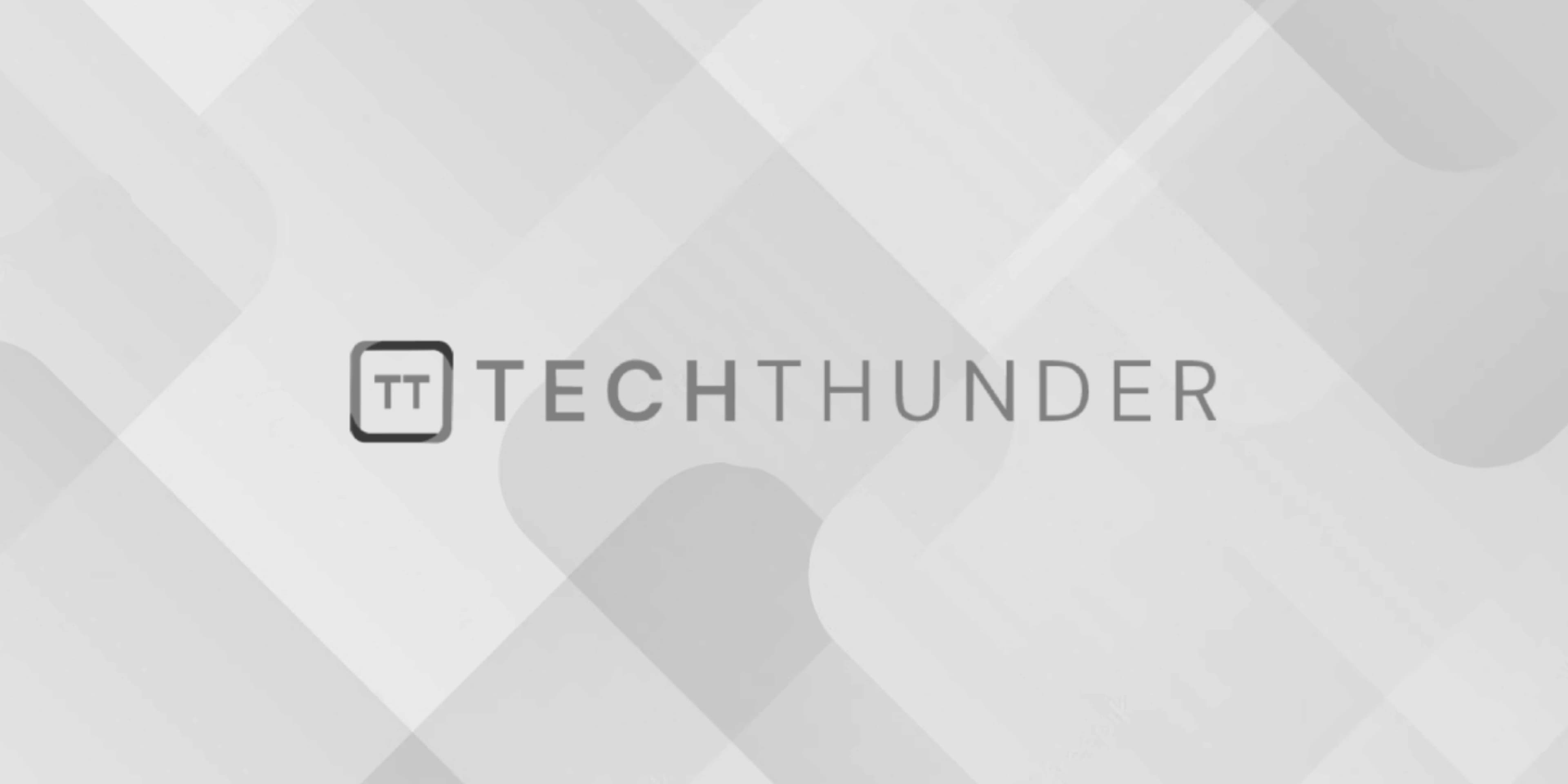
School Fee Enquiry System in C++
Creating a complete “School Fee Enquiry System” in C++ would be a substantial project, but I can provide you with a simplified example that demonstrates the basic structure and functionality of such a system. In a real-world application, you would typically use a database to store and manage student and fee information. In this simplified example, we’ll use data structures to represent this information.
Here’s a basic outline of a C++ program for a simplified School Fee Enquiry System:
#include <iostream>
#include <string>
#include <vector>
// Structure to represent a student
struct Student {
int rollNumber;
std::string name;
double feesPaid;
double totalFees;
};
// Function to display student details
void displayStudent(const Student& student) {
std::cout << "Roll Number: " << student.rollNumber << std::endl;
std::cout << "Name: " << student.name << std::endl;
std::cout << "Fees Paid: " << student.feesPaid << std::endl;
std::cout << "Total Fees: " << student.totalFees << std::endl;
}
int main() {
std::vector<Student> students;
// Add some sample student data
students.push_back({101, "Alice", 2000.0, 5000.0});
students.push_back({102, "Bob", 3000.0, 5000.0});
students.push_back({103, "Charlie", 4000.0, 5000.0});
// Main menu
int choice;
do {
std::cout << "\nSchool Fee Enquiry System" << std::endl;
std::cout << "1. Display Student Details" << std::endl;
std::cout << "2. Exit" << std::endl;
std::cout << "Enter your choice: ";
std::cin >> choice;
switch (choice) {
case 1: {
int rollNumber;
std::cout << "Enter Roll Number: ";
std::cin >> rollNumber;
bool found = false;
// Search for the student by roll number
for (const Student& student : students) {
if (student.rollNumber == rollNumber) {
displayStudent(student);
found = true;
break;
}
}
if (!found) {
std::cout << "Student not found." << std::endl;
}
break;
}
case 2:
std::cout << "Exiting the program." << std::endl;
break;
default:
std::cout << "Invalid choice. Please try again." << std::endl;
}
} while (choice != 2);
return 0;
}
This simplified example includes the following features:
- A
Student
structure to represent student information, including roll number, name, fees paid, and total fees. - A
displayStudent
function to display a student’s details. - A vector to store a collection of students as sample data.
- A menu-driven interface that allows you to display student details by entering a roll number.
Please note that this is a basic example for demonstration purposes. In a real-world application, you would need to implement more advanced features, such as data persistence, data validation, and user authentication, and possibly use a database to store and manage the information.