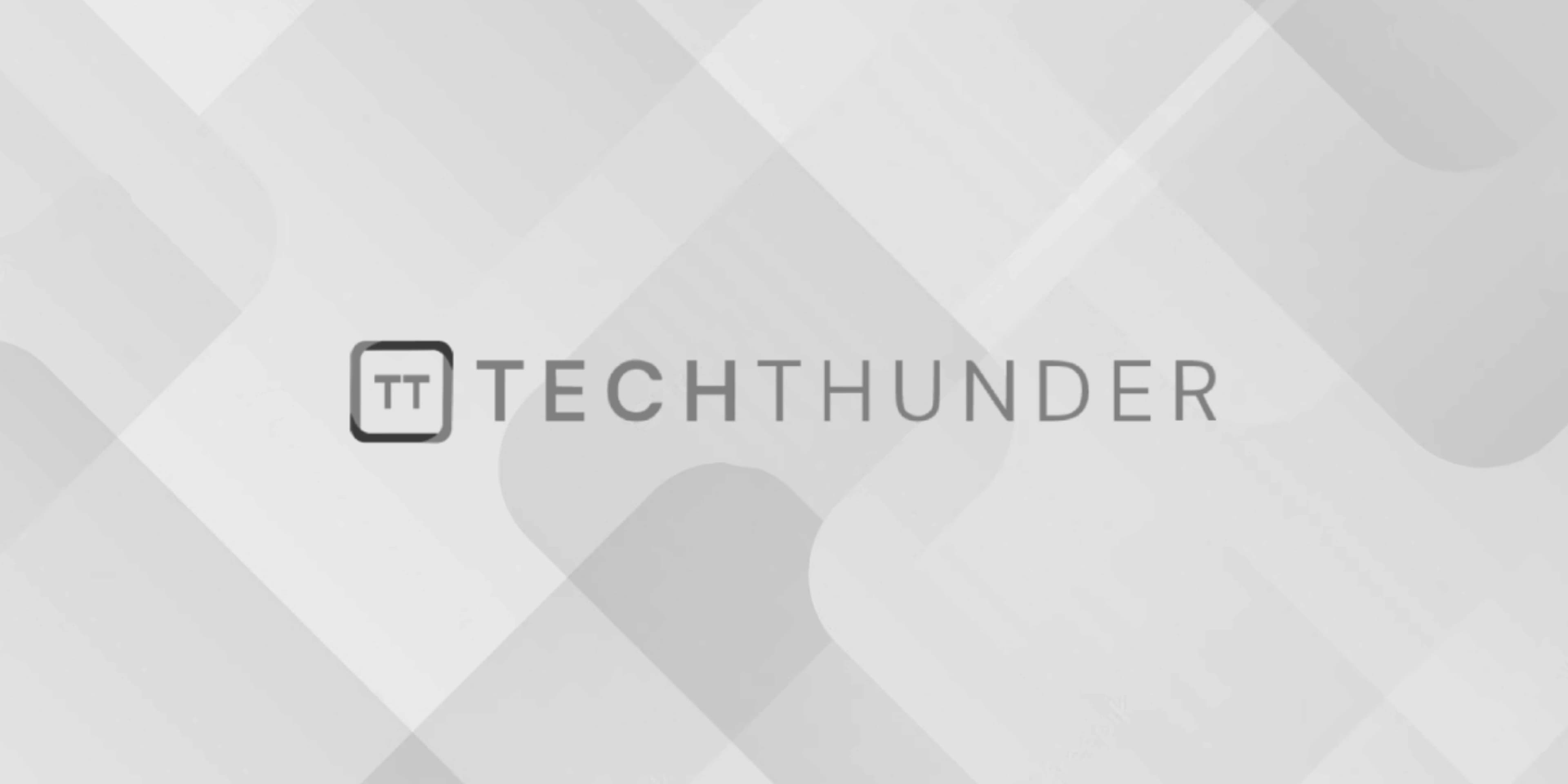
Simple Car Racing Game Code in C++
Creating a complete car racing game in C++ is a complex task that would involve graphics and user input handling, which typically requires a graphics library or framework like SDL, SFML, or a game engine like Unity or Unreal Engine. However, I can provide you with a simple text-based car racing game as a starting point.
This example uses the console for input and output and demonstrates basic game mechanics. You can expand and enhance it as needed:
#include <iostream>
#include <cstdlib>
#include <ctime>
#include <conio.h> // For _kbhit() and _getch() on Windows
using namespace std;
const int roadWidth = 20;
int carPosition = roadWidth / 2;
bool gameover = false;
void Draw() {
system("cls"); // Clear the console screen
// Draw the road
for (int i = 0; i < roadWidth; ++i) {
if (i == 0 || i == roadWidth - 1)
cout << "#"; // Boundary of the road
else
cout << "-";
}
cout << endl;
// Draw the car
for (int i = 0; i < roadWidth; ++i) {
if (i == carPosition)
cout << "C"; // Car
else
cout << " ";
}
cout << endl;
// Draw the road
for (int i = 0; i < roadWidth; ++i) {
if (i == 0 || i == roadWidth - 1)
cout << "#"; // Boundary of the road
else
cout << "-";
}
cout << endl;
}
void Input() {
if (_kbhit()) { // Check if a key is pressed
char key = _getch(); // Get the pressed key
switch (key) {
case 'a':
if (carPosition > 1)
carPosition--;
break;
case 'd':
if (carPosition < roadWidth - 2)
carPosition++;
break;
case 'q':
gameover = true;
break;
}
}
}
void Logic() {
// No special logic in this simple example
}
int main() {
srand(static_cast<unsigned>(time(0))); // Seed for random numbers
while (!gameover) {
Draw();
Input();
Logic();
// Sleep for a short time to control the game speed
// Adjust this value to make the game faster or slower
// Sleep(milliseconds);
}
cout << "Game Over!" << endl;
return 0;
}
This code defines a simple text-based car racing game where you control a car with ‘a’ (left) and ‘d’ (right) keys. Press ‘q’ to quit the game. The game loop in main()
handles drawing the road and car, taking input from the user, and simulating game logic. You can customize and extend this code to make it more interesting and visually appealing, including adding features like obstacles, scoring, and more advanced graphics.