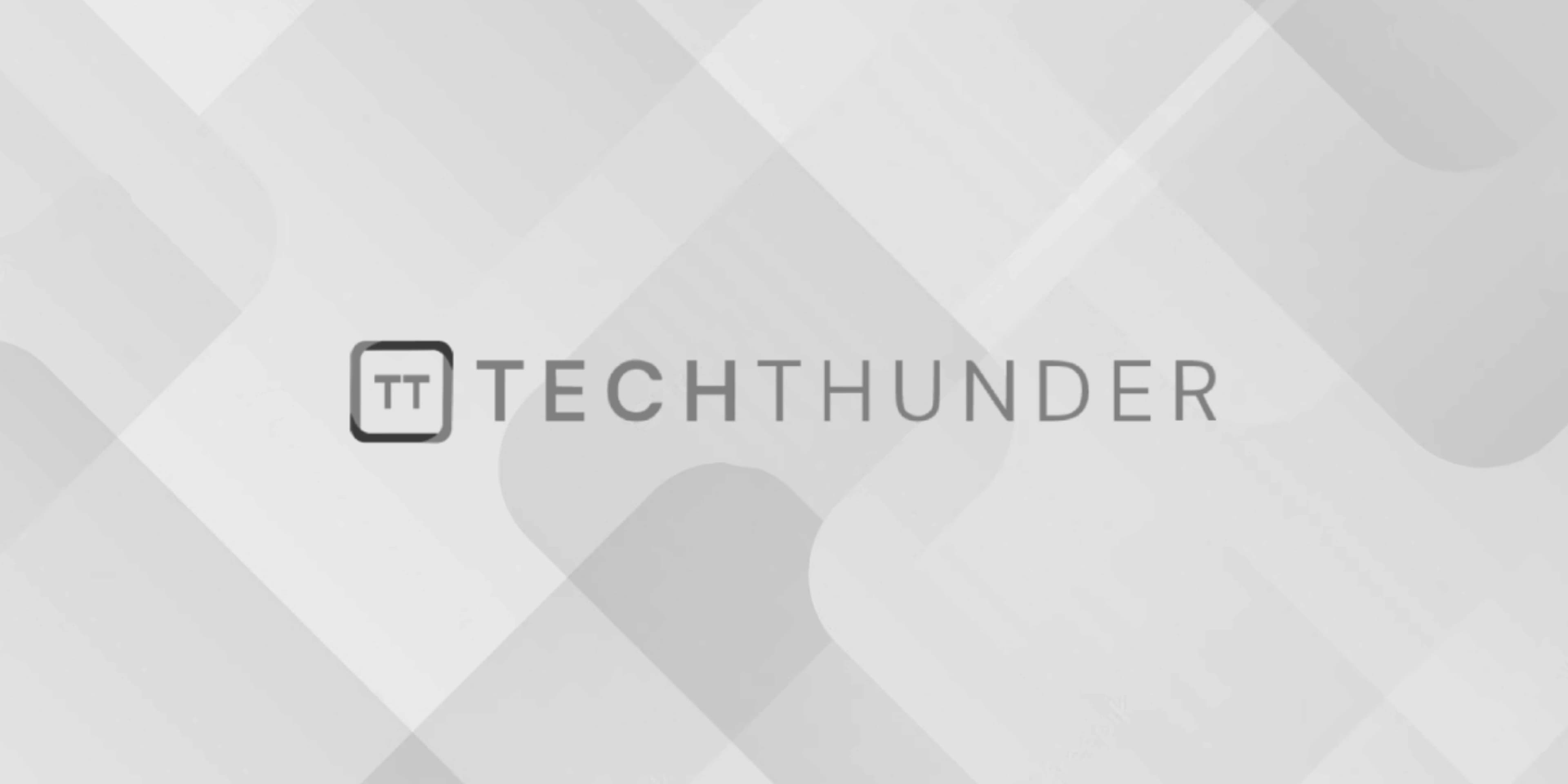
C++ Void Pointer
The C++ void*
(void pointer) is a special type of pointer that can point to objects of any data type. It is used for a variety of purposes, including generic programming, dynamic memory allocation, and interfacing with C code. However, because void*
is type-unsafe, you need to be careful when using it to avoid type-related errors.
Here are some common use cases and considerations for void*
pointers in C++:
1. Dynamic Memory Allocation:
void*
pointers are often used in conjunction with memory allocation functions like malloc
(in C) or new
(in C++) to allocate memory for objects of unknown or varying types.
Using malloc
and free
(C):
void* ptr = malloc(sizeof(int)); // Allocate memory for an integer
if (ptr != nullptr) {
int* intPtr = static_cast<int*>(ptr); // Cast to the appropriate type
*intPtr = 42; // Assign a value
free(ptr); // Deallocate memory
}
Using new
and delete
(C++):
void* ptr = new int; // Allocate memory for an integer
if (ptr != nullptr) {
int* intPtr = static_cast<int*>(ptr); // Cast to the appropriate type
*intPtr = 42; // Assign a value
delete intPtr; // Deallocate memory
}
2. Generic Programming:
void*
pointers are sometimes used in generic programming to create data structures or algorithms that can work with various data types without needing to know their specific types at compile time. This can be useful for creating container classes like linked lists or binary trees that can store elements of different types.
3. Interfacing with C Code:
When working with C libraries or APIs, you may encounter functions that use void*
pointers to pass data between C and C++ code. You’ll need to cast these pointers to the appropriate types when you know their actual types.
4. Type Safety and Casting:
Using void*
pointers requires careful type casting to avoid type-related errors. You should always cast them back to the correct data type before dereferencing them. The static_cast
, dynamic_cast
, or reinterpret_cast
operators can be used for type casting, depending on the situation.
void* ptr = /* ... */;
int* intPtr = static_cast<int*>(ptr); // Casting void* to int*
5. Potential Pitfalls:
Using void*
pointers can lead to runtime errors if not used carefully. For example, dereferencing an incorrectly cast void*
pointer or accessing it after the memory it points to has been deallocated can result in undefined behavior.
In modern C++, especially when dealing with type-safe code, it’s often better to avoid using void*
pointers when possible and rely on C++ features like templates and polymorphism to achieve type safety. However, there are situations, such as when working with legacy code or interfacing with C libraries, where void*
pointers remain useful. In such cases, use them with caution and ensure proper type casting and memory management.