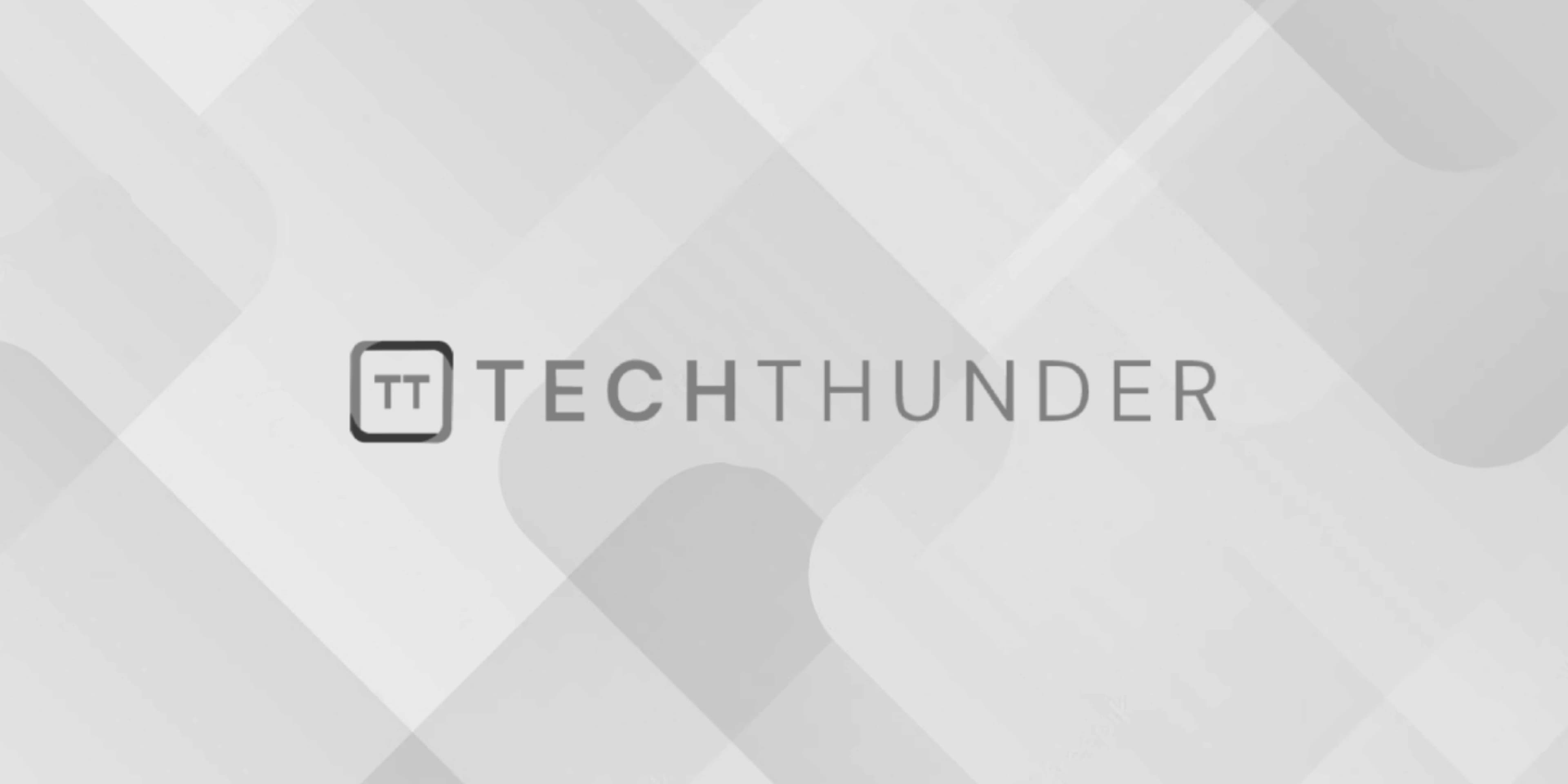
How to generate random number between 1 to 10 in C++
The C++ generate random numbers within a specific range using the <random>
header, which provides various classes and functions for random number generation. To generate a random number between 1 and 10, you can use the std::uniform_int_distribution
class along with a random number generator engine, such as std::mt19937
(Mersenne Twister) or std::default_random_engine
.
Here’s an example of how to generate a random number between 1 and 10:
#include <iostream>
#include <random>
int main() {
// Create a random number generator engine
std::random_device rd; // Seed from hardware entropy source (if available)
std::mt19937 rng(rd()); // Mersenne Twister engine with rd as seed
// Create a uniform distribution for integers in the range [1, 10]
std::uniform_int_distribution<int> distribution(1, 10);
// Generate a random number
int random_number = distribution(rng);
std::cout << "Random number between 1 and 10: " << random_number << std::endl;
return 0;
}
In this example, std::random_device
is used to obtain a random seed from the hardware entropy source (if available) to initialize the random number generator engine std::mt19937
. The std::uniform_int_distribution
is then used to specify the range [1, 10] for generating integers. Finally, distribution(rng)
generates a random number between 1 and 10 using the specified engine and distribution.
Remember to include the <random>
header to use the random number generation facilities. Keep in mind that the exact method and quality of random number generation might vary depending on the platform and C++ standard library implementation.