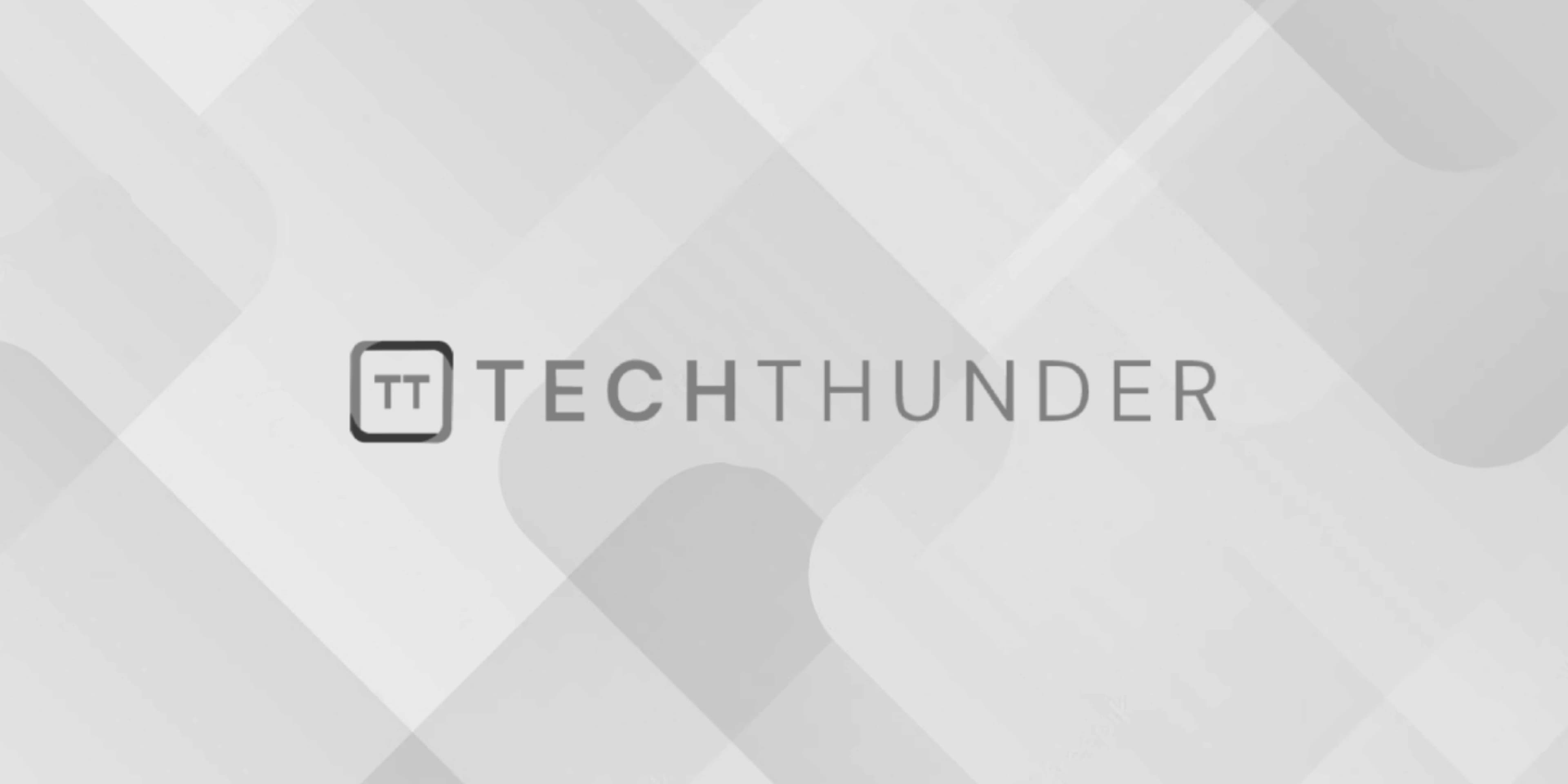
118 views
Scope Resolution Operator in C++
The C++ scope resolution operator (::
) is used to access members (variables, functions, or types) that are defined in a particular scope or namespace. It allows you to specify the exact scope or namespace from which you want to access a member, especially when there is ambiguity or when you want to access global or non-local entities.
Here are some common uses of the scope resolution operator in C++:
- Accessing Global Variables and Functions: You can use
::
to access global variables and functions from within a local scope or a class member function.
C++
int globalVar = 42;
int main() {
int localVar = 10;
std::cout << globalVar << std::endl; // Accessing globalVar using ::
return 0;
}
- Accessing Static Class Members: When dealing with static members of a class, you use the scope resolution operator to access them.
C++
class MyClass {
public:
static int staticVar;
};
int MyClass::staticVar = 100; // Definition outside the class
int main() {
int localVar = MyClass::staticVar; // Accessing staticVar using ::
return 0;
}
- Resolving Namespace Conflicts: When there are multiple namespaces with conflicting names, you can use the scope resolution operator to specify which namespace a particular identifier belongs to.
C++
namespace A {
int value = 5;
}
namespace B {
int value = 10;
}
int main() {
int aValue = A::value; // Accessing A::value
int bValue = B::value; // Accessing B::value
return 0;
}
- Accessing Nested Class Members: In the case of nested classes, you can use the scope resolution operator to access members of the inner class from the outer class.
C++
class Outer {
public:
class Inner {
public:
static int innerVar;
};
};
int Outer::Inner::innerVar = 42; // Accessing Inner::innerVar using ::
int main() {
int localVar = Outer::Inner::innerVar;
return 0;
}
- Accessing Enumerations: The scope resolution operator can also be used to access enumeration values.
C++
enum Colors {
RED,
GREEN,
BLUE
};
int main() {
int colorValue = Colors::GREEN; // Accessing enum value using ::
return 0;
}
The scope resolution operator is a powerful tool in C++ that helps you specify which scope or namespace you want to access when dealing with identifiers that may have the same name in different scopes. It enhances code readability and avoids naming conflicts.