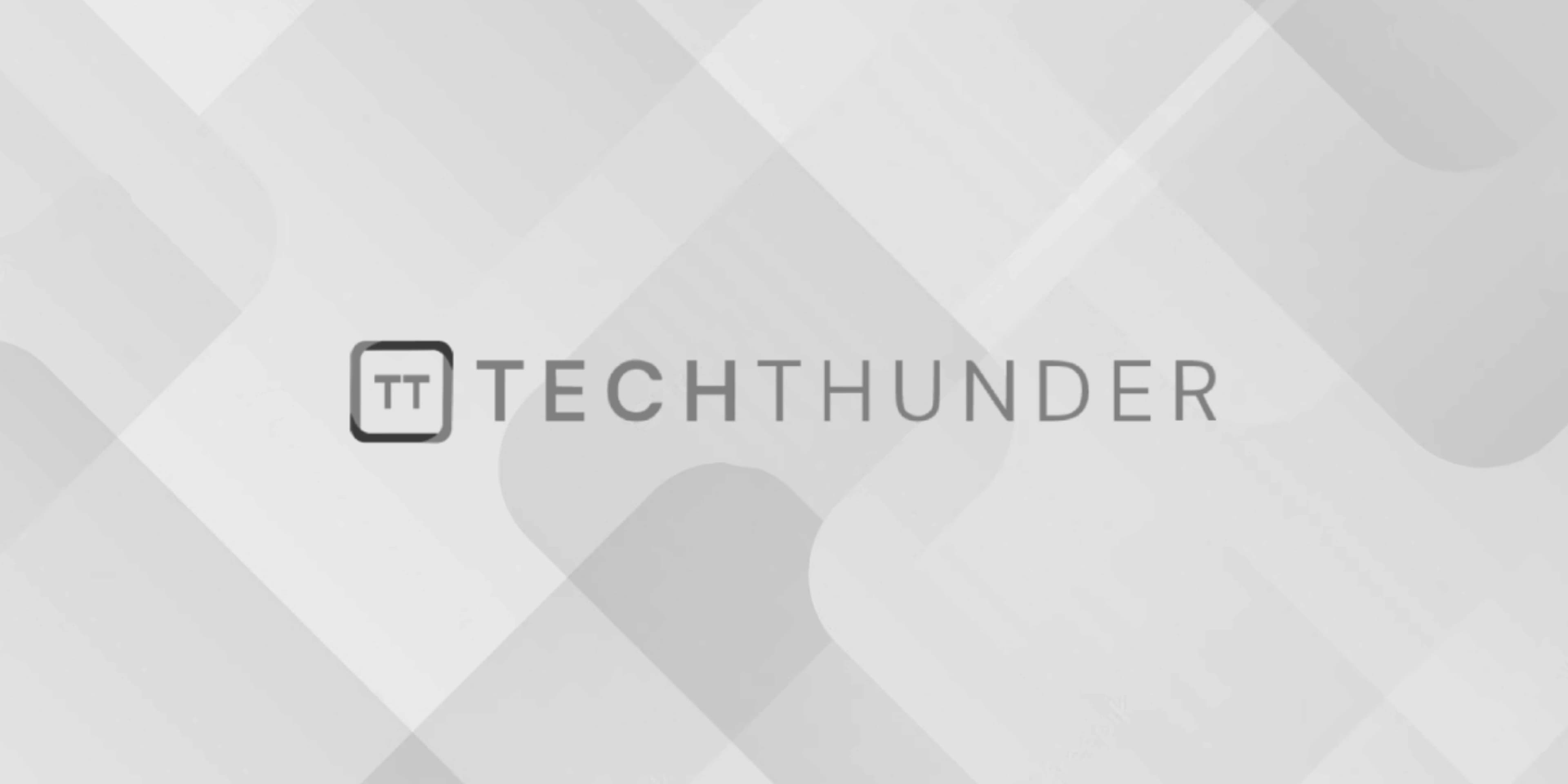
Factorial in C++
To calculate the factorial of a non-negative integer in C++, you can use a loop or recursion. Here’s how to compute the factorial of a number using both methods:
Using a Loop (Iterative):
#include <iostream>
int main() {
int n;
unsigned long long factorial = 1; // Use an unsigned long long to handle large factorials
// Input a non-negative integer
std::cout << "Enter a non-negative integer: ";
std::cin >> n;
// Check if n is negative
if (n < 0) {
std::cout << "Factorial is not defined for negative numbers." << std::endl;
} else {
// Calculate factorial using a loop
for (int i = 1; i <= n; ++i) {
factorial *= i;
}
std::cout << "Factorial of " << n << " is " << factorial << std::endl;
}
return 0;
}
In this program, an unsigned long long
variable is used to handle large factorials. A for
loop is used to calculate the factorial of the input number.
Using Recursion:
#include <iostream>
unsigned long long factorial(int n) {
if (n == 0 || n == 1) {
return 1;
} else {
return n * factorial(n - 1);
}
}
int main() {
int n;
// Input a non-negative integer
std::cout << "Enter a non-negative integer: ";
std::cin >> n;
// Check if n is negative
if (n < 0) {
std::cout << "Factorial is not defined for negative numbers." << std::endl;
} else {
unsigned long long result = factorial(n);
std::cout << "Factorial of " << n << " is " << result << std::endl;
}
return 0;
}
In this program, a recursive function factorial
is defined to calculate the factorial of a number. The base case is when n
is 0 or 1, in which case the factorial is 1. Otherwise, the function calls itself with n-1
and multiplies the result by n
.
Both programs check for negative input and provide appropriate error messages. Compile and run either program to calculate the factorial of a non-negative integer.