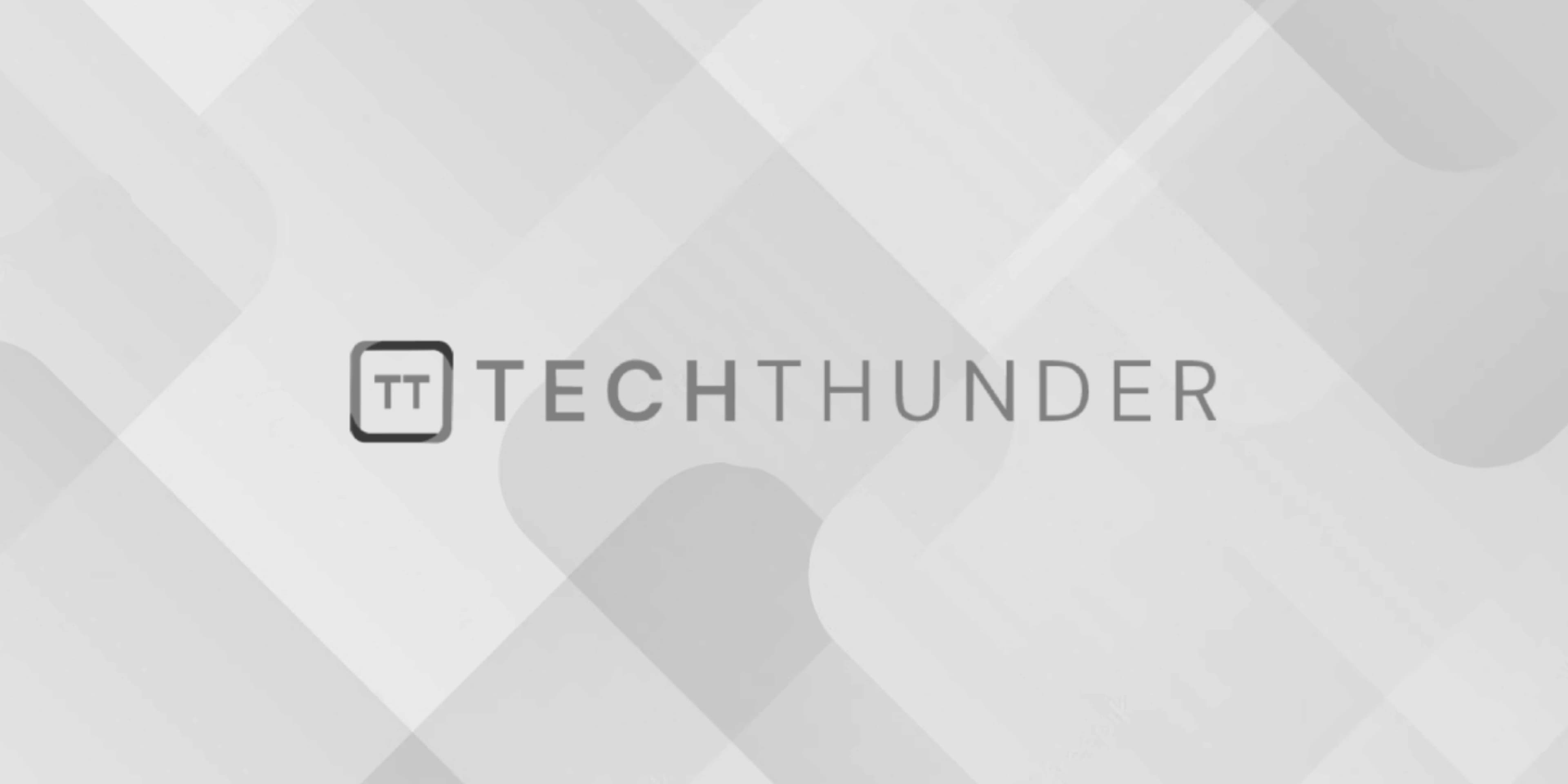
Virtual Destructor in C++
The C++, when you have a class hierarchy with inheritance and you use polymorphism (i.e., you have base class pointers or references pointing to derived class objects), it’s a good practice to use a virtual destructor in the base class. A virtual destructor helps ensure that the destructor of the most derived class in the hierarchy is called when an object is deleted through a base class pointer or reference. This is essential for proper resource cleanup and preventing memory leaks.
Here’s how you declare and use a virtual destructor in C++:
class Base {
public:
Base() {
// Constructor code
}
virtual ~Base() {
// Virtual destructor code
}
};
class Derived : public Base {
public:
Derived() {
// Derived class constructor code
}
~Derived() {
// Derived class destructor code
}
};
int main() {
Base* basePtr = new Derived;
delete basePtr; // Calls the virtual destructor
return 0;
}
In the code above:
- The
Base
class has a virtual destructor declared with thevirtual
keyword. - The
Derived
class is derived fromBase
and also has a destructor. - In the
main
function, we create aDerived
object using aBase
pointer (Base* basePtr = new Derived;
). Since the destructor inBase
is declared as virtual, when we calldelete basePtr
, it correctly calls the destructor of the most derived class, which isDerived
.
If you don’t use a virtual destructor in the base class, deleting an object through a base class pointer when the object is of a derived class type may lead to resource leaks because only the base class destructor would be called.
In summary, using a virtual destructor is essential in C++ when working with class hierarchies and polymorphism to ensure proper cleanup and prevent resource leaks when deleting objects through base class pointers or references.