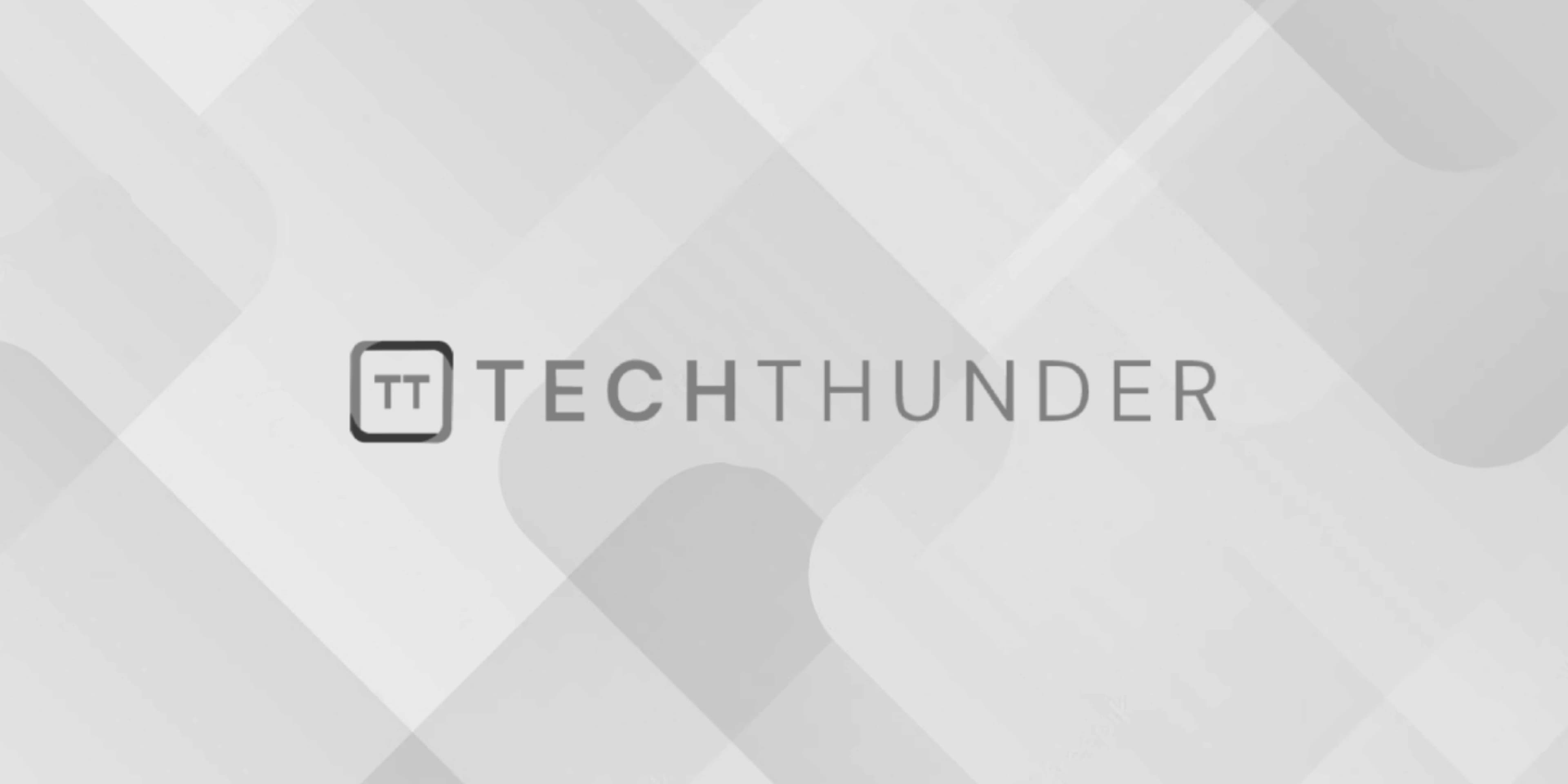
When do we pass arguments by reference or pointer in C++
The C++ can pass function arguments by reference or by pointer when you want to avoid making a copy of the argument. Passing by reference or pointer is often used to improve performance, modify the original object, or work with large data structures more efficiently. Here are some common scenarios for passing arguments by reference or pointer:
1. Modifying the original object: If you need to modify the original object within a function, passing by reference or pointer is preferred because changes made to the object will affect the original.
// Pass by reference
void modifyValueByReference(int& x) {
x += 10;
}
int main() {
int value = 5;
modifyValueByReference(value);
// value is now 15
return 0;
}
2. Avoiding unnecessary copying: When passing large objects or data structures (e.g., vectors, strings) to functions, passing by reference or pointer can be significantly more efficient than passing by value because it avoids copying the entire object.
// Pass by reference
void processVectorByReference(std::vector<int>& vec) {
// Modify vec directly
vec.push_back(42);
}
int main() {
std::vector<int> data = {1, 2, 3};
processVectorByReference(data);
// data now contains {1, 2, 3, 42}
return 0;
}
3. Returning multiple values: If you need to return multiple values from a function, you can use references or pointers to modify values within the function and pass them back.
// Pass by reference
void calculateSumAndProduct(int a, int b, int& sum, int& product) {
sum = a + b;
product = a * b;
}
int main() {
int x = 3, y = 4, sum, product;
calculateSumAndProduct(x, y, sum, product);
// sum is 7, product is 12
return 0;
}
4. Dynamic memory allocation: When working with dynamically allocated objects (created with new
), passing by pointer is common to avoid copying the entire object. Be cautious when passing raw pointers to manage memory properly and avoid memory leaks.
// Pass by pointer
void deleteAndSetToNull(int* ptr) {
delete ptr;
ptr = nullptr; // Avoid a dangling pointer
}
int main() {
int* value = new int(42);
deleteAndSetToNull(value);
// value is now nullptr
return 0;
}
5. Optional or nullable arguments: When a function parameter is optional or can be absent, passing by pointer (with a null pointer as a default value) or using references with optional wrapper types (e.g., std::optional
) can be a suitable approach.
// Pass by pointer (with nullptr as a default)
void processOptionalValue(int* ptr = nullptr) {
if (ptr) {
// Value is present
} else {
// Value is absent
}
}
int main() {
int value = 42;
processOptionalValue(&value);
processOptionalValue(); // Value is absent
return 0;
}
In summary, you pass arguments by reference or pointer in C++ when you want to modify the original object, avoid copying large objects, return multiple values, work with dynamically allocated memory, or handle optional or nullable arguments efficiently. However, be mindful of proper memory management and potential null or dangling pointer issues when using pointers.