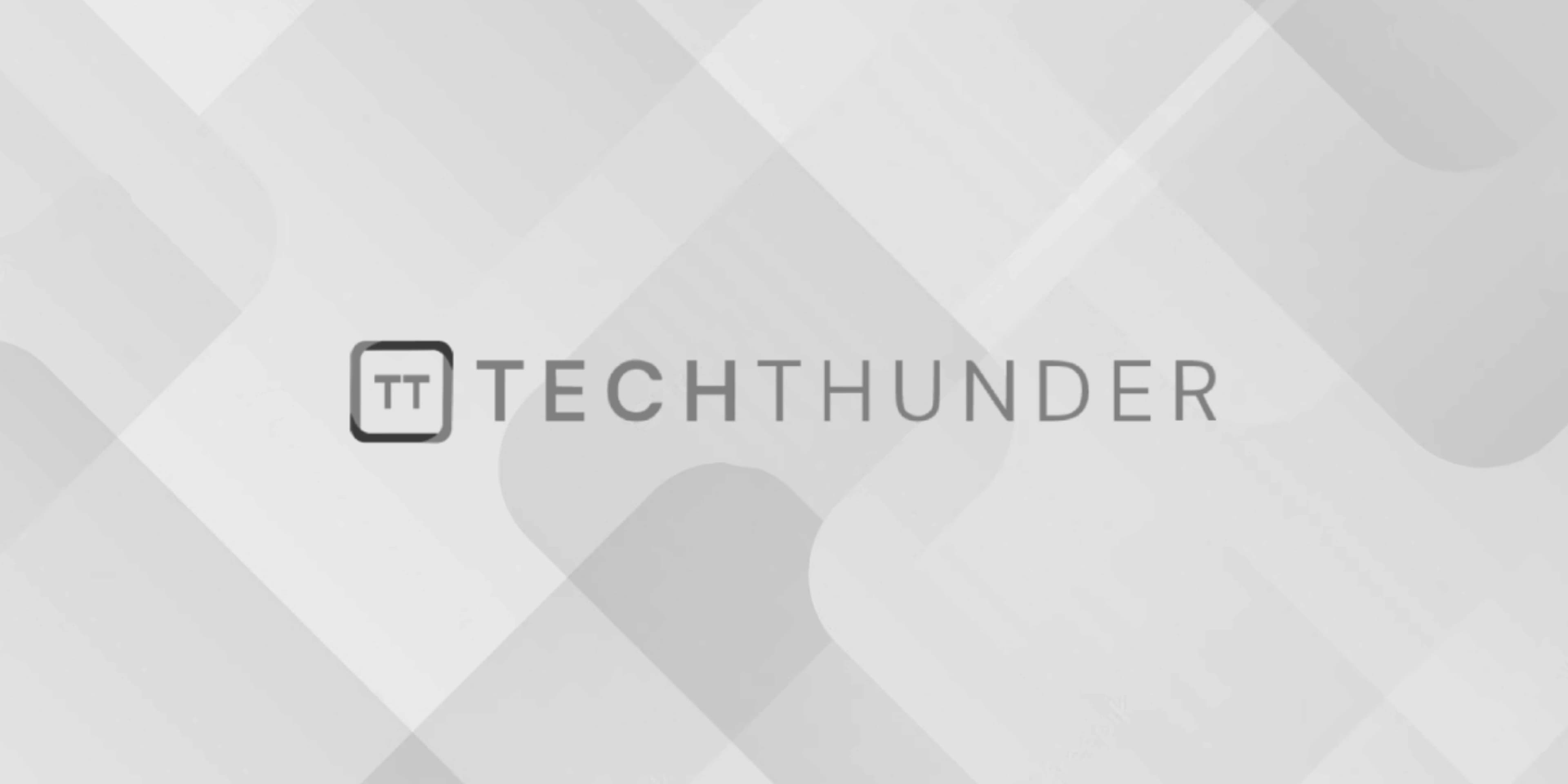
C++ Bitwise XOR Operator
The C++, the bitwise XOR operator is represented by the caret symbol ^
. It is used to perform bitwise XOR (exclusive OR) operations on individual bits of two integers. The XOR operation returns 1 if the corresponding bits of the two operands are different, and it returns 0 if they are the same.
Here’s the basic syntax of the bitwise XOR operator:
result = operand1 ^ operand2;
Here’s how it works with two binary numbers:
1010 (operand1)
^ 1100 (operand2)
---------
0110 (result)
In this example, the bitwise XOR operation is performed on each pair of corresponding bits. If the bits are different, the result is 1; otherwise, it’s 0.
Here’s a simple C++ code example illustrating the use of the bitwise XOR operator:
#include <iostream>
int main() {
int num1 = 10; // binary: 1010
int num2 = 12; // binary: 1100
int result = num1 ^ num2;
std::cout << "num1 ^ num2 = " << result << std::endl; // Output: 6 (binary: 0110)
return 0;
}
In this code, num1 ^ num2
performs a bitwise XOR operation on num1
and num2
, and the result is stored in the result
variable. The output demonstrates that the XOR operation yields the result 6, which corresponds to the binary 0110
.