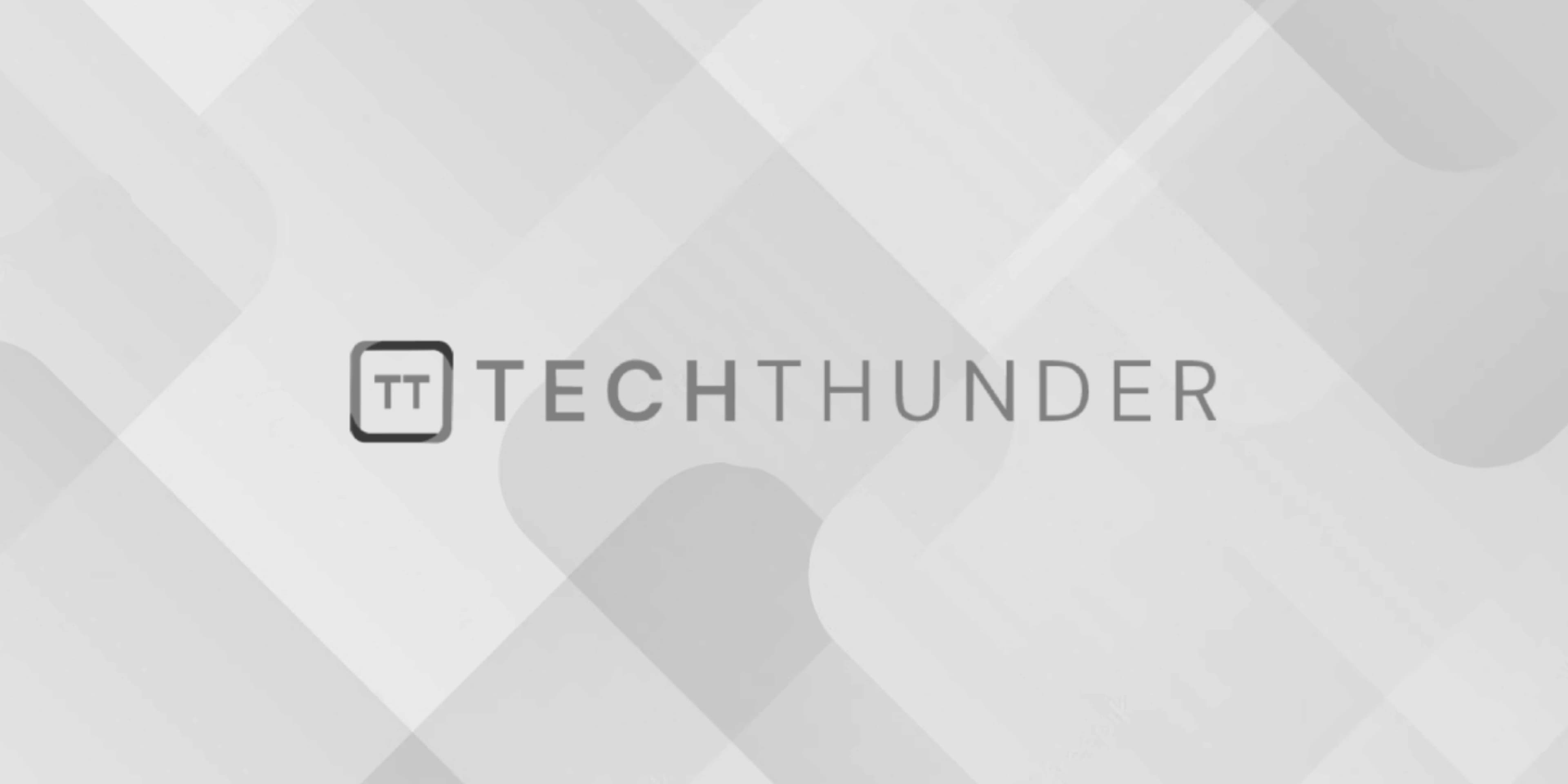
C++ Goto Statement
The C++ goto
statement is used to transfer control to a labeled statement within the same function or block of code. The goto
statement is considered a structured programming construct but is often discouraged because it can lead to unstructured and hard-to-read code. Instead, it’s usually better to use control structures like loops and conditional statements (e.g., for
, while
, if
, else
) to achieve the desired flow of execution.
The basic syntax of the goto
statement is as follows:
label_name:
// Code here
// Somewhere else in your code
goto label_name; // Jump to the labeled statement
Here’s an example of how the goto
statement can be used:
#include <iostream>
int main() {
int i = 1;
start: // Label
if (i <= 5) {
std::cout << i << " ";
i++;
goto start; // Jump to the 'start' label
}
std::cout << std::endl;
return 0;
}
Output:
1 2 3 4 5
In this example, a label called start
is defined, and the program uses goto
to jump back to that label within a loop. It effectively creates a loop without using a standard loop construct like for
or while
. However, using goto
in this manner is generally discouraged because it can make code harder to understand and maintain.
It’s important to note that the use of goto
should be limited, and it should be avoided when more structured and readable alternatives are available. Modern C++ code typically relies on loops and conditional statements for control flow, as they provide clearer and more maintainable code.