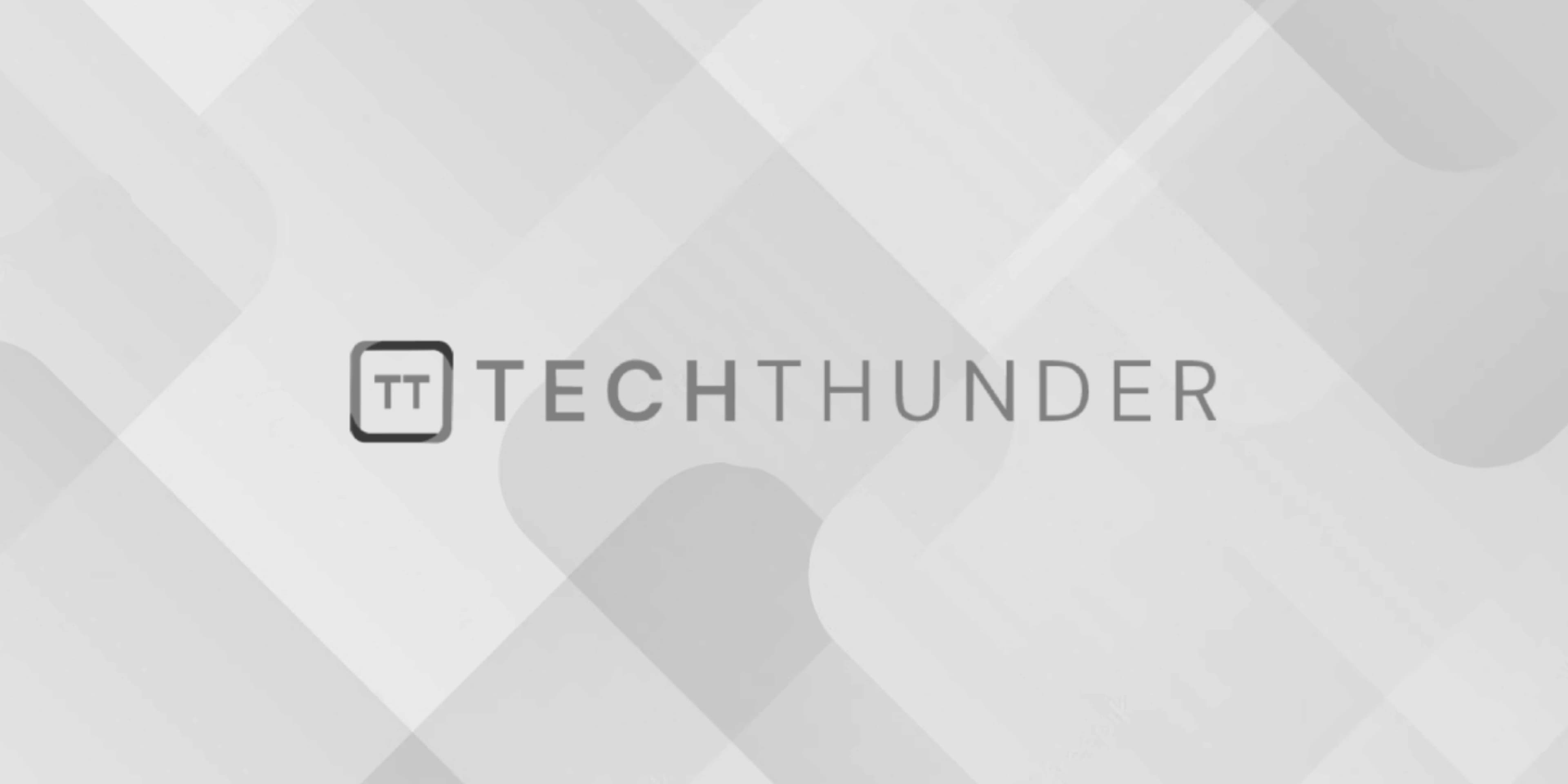
126 views
How to Split strings in C++
The C++ can split a string into substrings using various techniques. Here are two common methods: using std::istringstream
and using std::getline()
.
Method 1: Using std::istringstream
:
C++
#include <iostream>
#include <sstream>
#include <vector>
#include <string>
int main() {
std::string inputString = "This is a sample string to split";
std::istringstream iss(inputString);
std::vector<std::string> tokens;
std::string token;
while (std::getline(iss, token, ' ')) {
tokens.push_back(token);
}
// Display the split substrings
for (const std::string& str : tokens) {
std::cout << str << std::endl;
}
return 0;
}
In this example:
- We create a
std::istringstream
object namediss
and initialize it with the input string. - We use a
while
loop andstd::getline()
to split the string by space (‘ ‘) and store the substrings in a vector (tokens
in this case). - Finally, we loop through the vector to display the split substrings.
Method 2: Using std::getline()
directly:
C++
#include <iostream>
#include <string>
#include <vector>
int main() {
std::string inputString = "This is a sample string to split";
std::vector<std::string> tokens;
std::istringstream iss(inputString);
std::string token;
while (std::getline(iss, token, ' ')) {
tokens.push_back(token);
}
// Display the split substrings
for (const std::string& str : tokens) {
std::cout << str << std::endl;
}
return 0;
}
Both methods accomplish the same task of splitting a string into substrings based on a delimiter (‘ ‘ in this case). You can replace the space character with any other delimiter as needed.
Remember to include the necessary headers (#include <iostream>
, #include <sstream>
, #include <string>
, and #include <vector>
) at the beginning of your code.