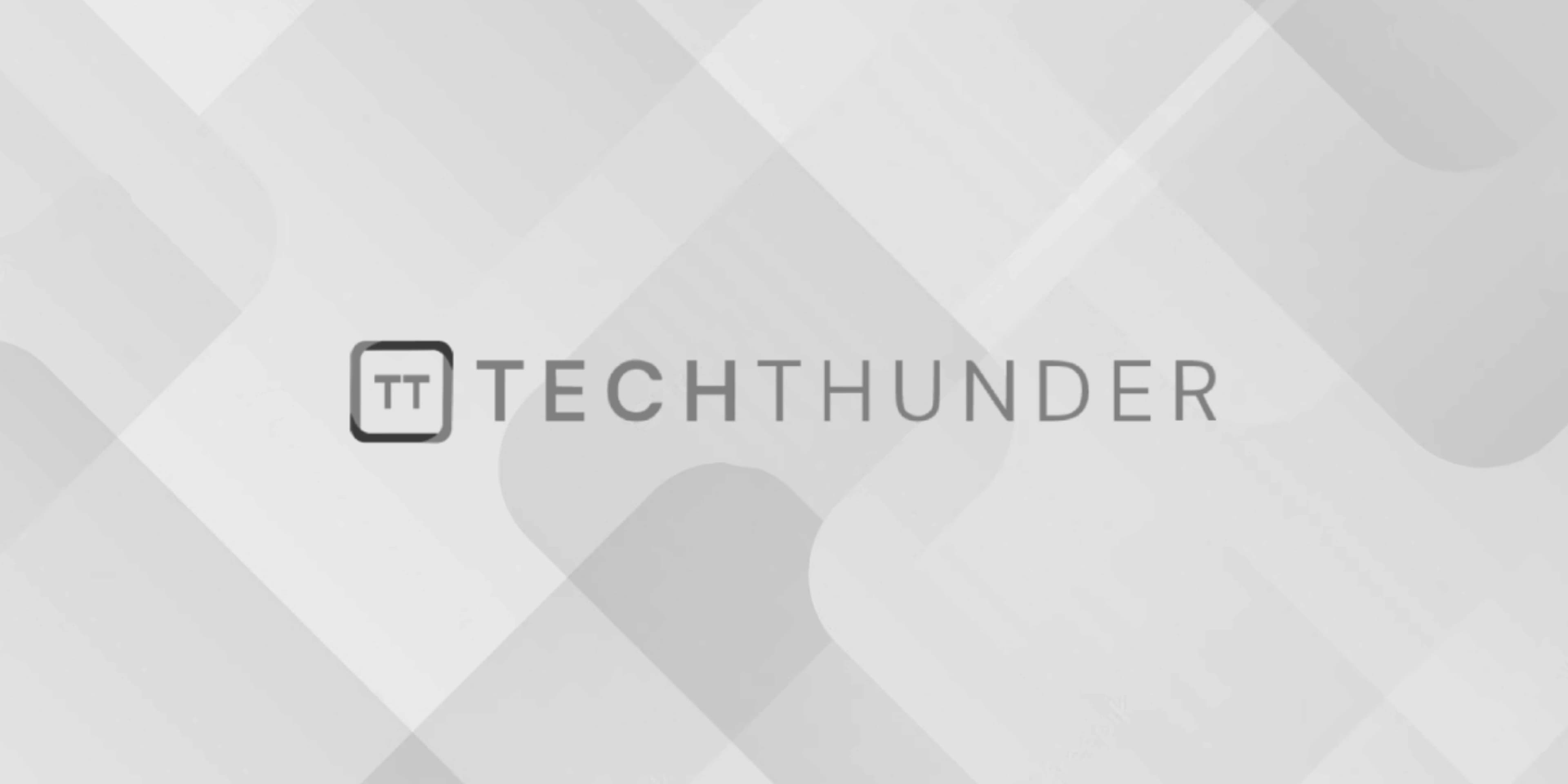
Delete Operator in C++
The C++ delete
operator is used to deallocate memory that was previously allocated using the new
operator. This operator is essential for dynamic memory management, especially when working with objects and arrays of objects. Properly deallocating memory with delete
helps prevent memory leaks in your C++ programs.
Here’s how you use the delete
operator:
Deleting a Single Object:
// Allocate memory for a single integer
int* ptr = new int;
// Use the allocated memory
// Deallocate the memory when it's no longer needed
delete ptr;
In the example above, new int
dynamically allocates memory for a single integer and returns a pointer to that memory. The delete
operator is then used to release that memory once it’s no longer needed.
Deleting an Array:
// Allocate memory for an array of integers
int* arr = new int[5];
// Use the allocated array
// Deallocate the array when it's no longer needed
delete[] arr;
When you use new[]
to allocate memory for an array, you must use delete[]
to deallocate it. Failing to use the correct form of delete
can lead to memory leaks or undefined behavior.
Deleting Objects:
class MyClass {
public:
MyClass() {
std::cout << "MyClass constructor" << std::endl;
}
~MyClass() {
std::cout << "MyClass destructor" << std::endl;
}
};
int main() {
// Allocate a single object dynamically
MyClass* obj = new MyClass;
// Use the object
// Deallocate the object
delete obj;
return 0;
}
In this example, we allocate an object of class MyClass
dynamically using new
and deallocate it using delete
. The destructor of MyClass
is automatically called when delete
is used, allowing you to perform cleanup operations if necessary.
It’s important to note the following points about the delete
operator:
- Always pair
new
withdelete
andnew[]
withdelete[]
. Using the wrong form ofdelete
for memory allocated withnew[]
or vice versa can lead to undefined behavior. - Deleting memory that has already been deleted or was not allocated with
new
can also result in undefined behavior. - To prevent potential memory leaks, it’s a good practice to use smart pointers (
std::unique_ptr
andstd::shared_ptr
) or standard containers (e.g.,std::vector
) when possible, as they manage memory automatically and reduce the need for manual memory management withnew
anddelete
.