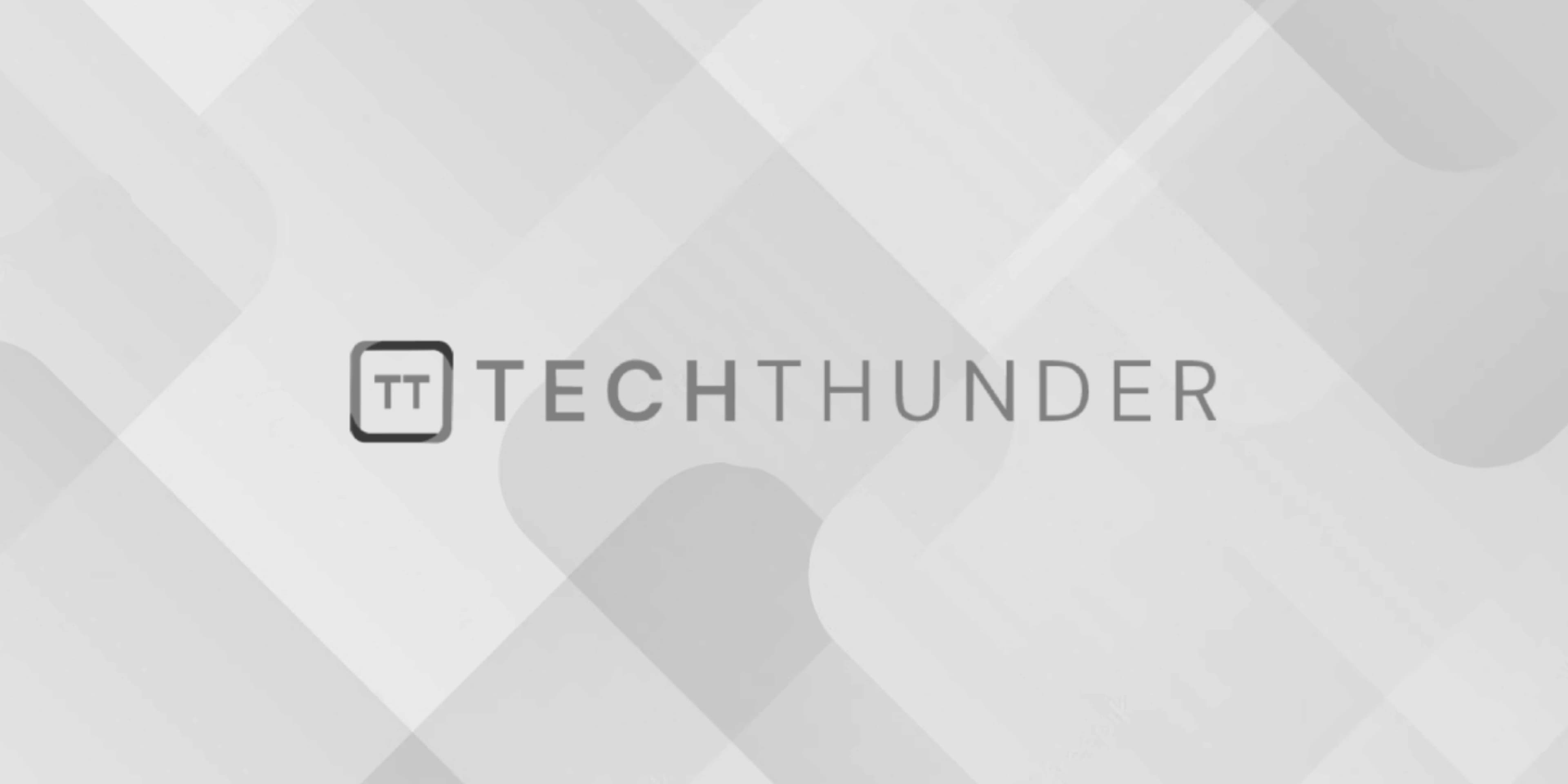
Inline function in C++
The C++, an inline function is a function that the compiler may choose to expand inline at the point of its call rather than performing a regular function call. The inline
keyword is used to declare a function as inline. Here are some key points about inline functions in C++:
- Purpose:
- The primary purpose of inline functions is to improve program performance by reducing the overhead of function call, especially for small, frequently used functions.
- Inline functions are a compiler optimization; they suggest to the compiler that it should replace the function call with the actual function code at the call site.
- Declaration:
- To declare a function as inline, you use the
inline
keyword in the function declaration or definition. - Inline functions should be defined in header files to ensure that the function’s code is available to all translation units that include the header.
inline int add(int a, int b) {
return a + b;
}
- Inlining Decision:
- The decision of whether a function is actually inlined or not is made by the compiler. The compiler may choose not to inline a function if it believes that inlining would not result in performance improvements or if the function is too complex.
- Factors such as function size, the number of calls, and the optimization level set for compilation influence the inlining decision.
- Function Complexity:
- Inline functions are most effective for small, simple functions. Functions with complex logic, large bodies, or functions that call other functions may not be suitable candidates for inlining.
- Header Files:
- It is common practice to declare and define inline functions in header files to make them accessible to other parts of the program.
- When an inline function is defined in a header and included in multiple source files, there may be multiple copies of the function code generated. This is usually not a problem due to the One Definition Rule (ODR), which allows multiple identical definitions of inline functions as long as they are the same.
- Drawbacks:
- Overusing inline functions can lead to code bloat, as multiple copies of the function’s code may be generated.
- The decision to inline a function is ultimately up to the compiler, so using the
inline
keyword does not guarantee inlining.
It’s important to note that modern C++ compilers are quite capable of making optimization decisions automatically, including function inlining, based on their analysis of the code. Therefore, you should use the inline
keyword judiciously and focus on writing clear, maintainable code, allowing the compiler to handle most optimization tasks. Explicitly marking functions as inline is generally recommended when there is a clear performance benefit, and the function meets the criteria for inlining.