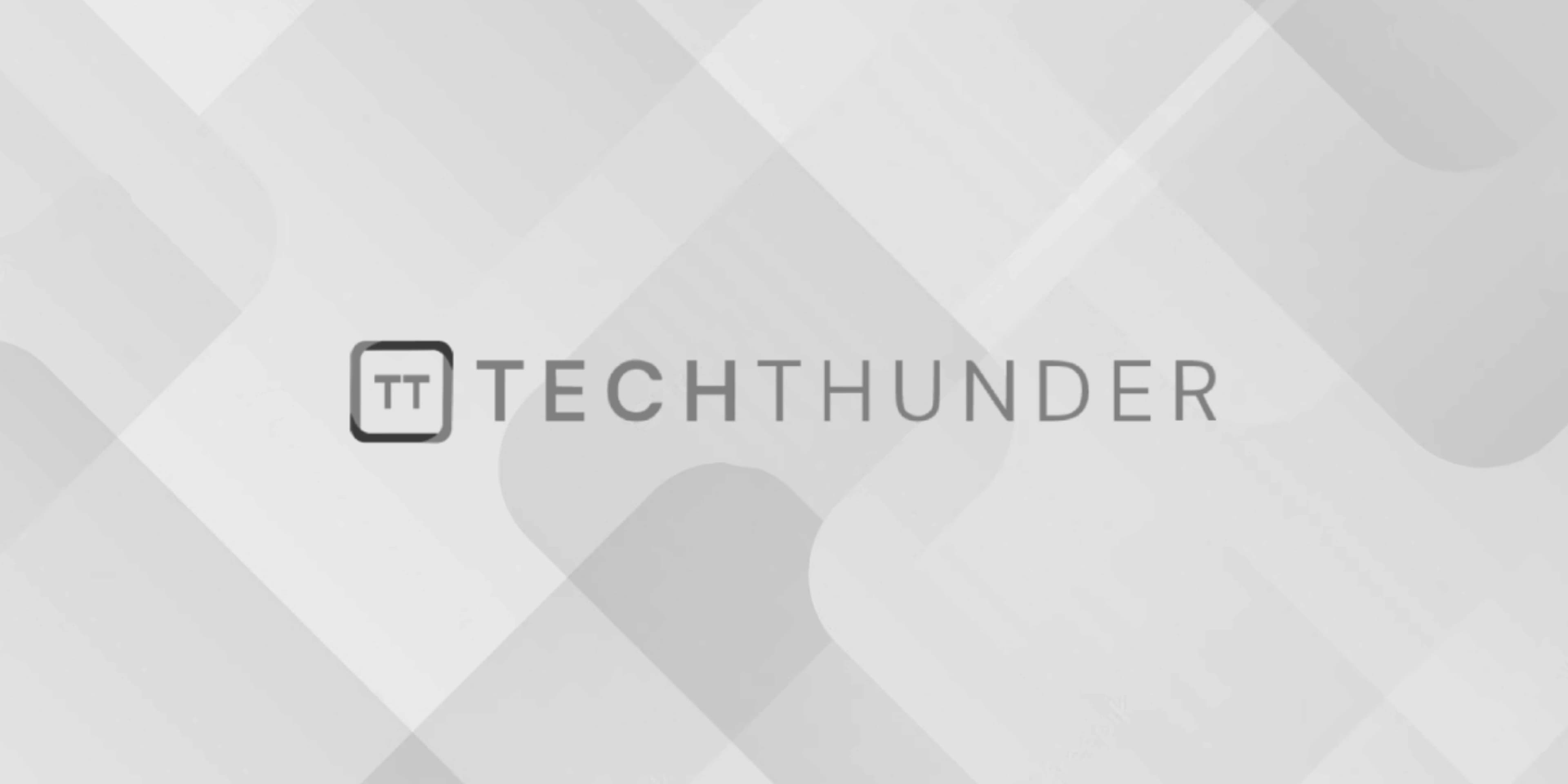
197 views
Calculator Program in C++
Creating a simple calculator program in C++ is a common programming exercise. Here’s a basic example of a calculator program that can perform addition, subtraction, multiplication, and division:
C++
#include <iostream>
int main() {
char operatorSymbol;
double num1, num2;
// Input
std::cout << "Enter an operator (+, -, *, /): ";
std::cin >> operatorSymbol;
std::cout << "Enter two numbers: ";
std::cin >> num1 >> num2;
// Perform the calculation based on the operator
switch (operatorSymbol) {
case '+':
std::cout << num1 << " + " << num2 << " = " << (num1 + num2) << std::endl;
break;
case '-':
std::cout << num1 << " - " << num2 << " = " << (num1 - num2) << std::endl;
break;
case '*':
std::cout << num1 << " * " << num2 << " = " << (num1 * num2) << std::endl;
break;
case '/':
if (num2 != 0) {
std::cout << num1 << " / " << num2 << " = " << (num1 / num2) << std::endl;
} else {
std::cout << "Error! Division by zero is not allowed." << std::endl;
}
break;
default:
std::cout << "Error! Invalid operator." << std::endl;
break;
}
return 0;
}
In this program:
- The program prompts the user to enter an operator (
+
,-
,*
,/
) and two numbers. - It uses a
switch
statement to perform the appropriate calculation based on the operator. - If the user attempts to divide by zero, the program displays an error message to prevent a division by zero error.
- If the user enters an invalid operator, the program displays an error message.
Compile and run this program, and you can perform basic arithmetic calculations using the provided operator and numbers.