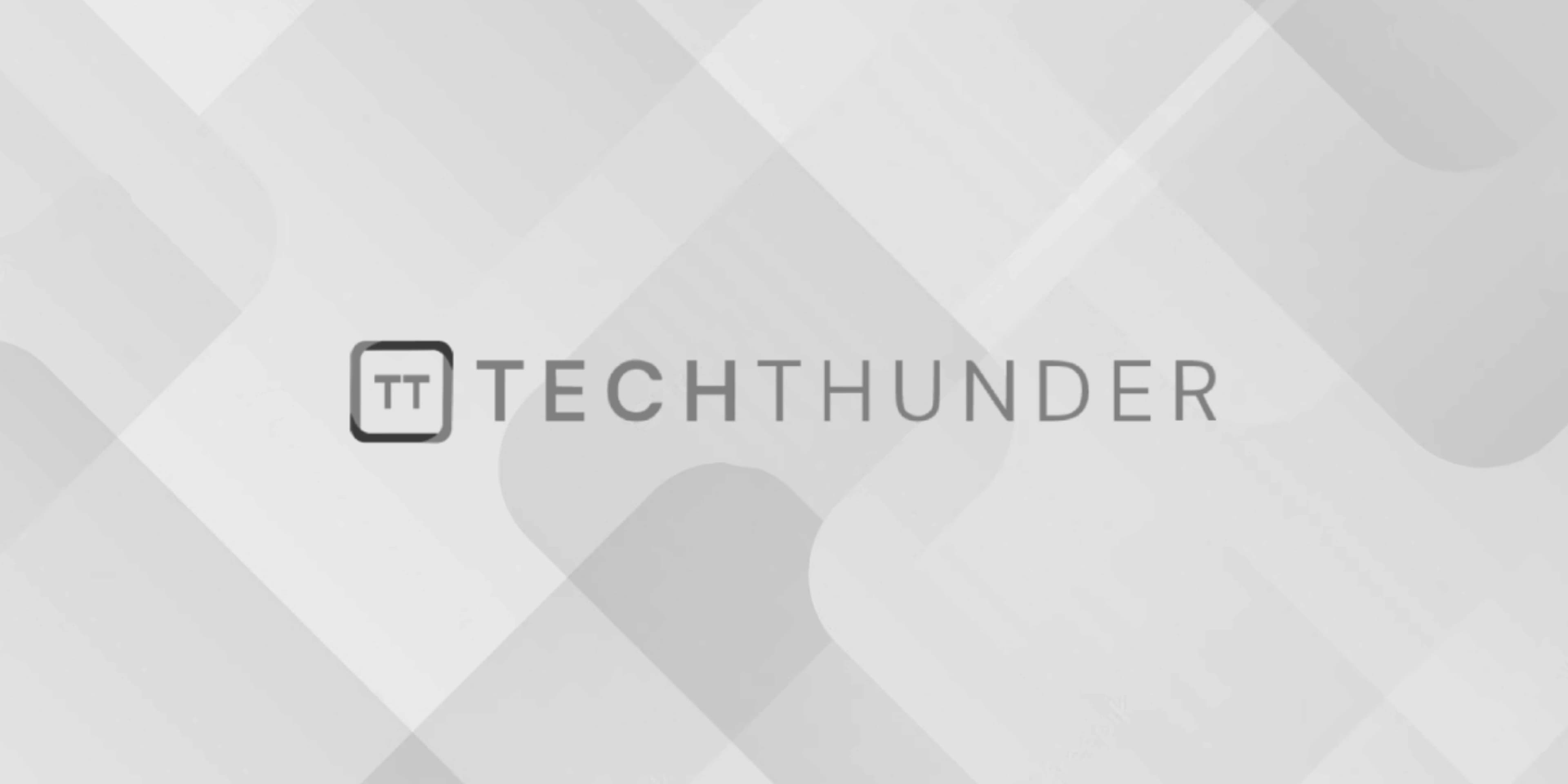
How to Create and use CComPtr and CComQIPtr Instances in C++
CComPtr
and CComQIPtr
are smart pointer classes provided by the Microsoft Active Template Library (ATL) for managing COM (Component Object Model) interfaces in C++. They are designed to simplify the memory management and lifetime management of COM objects.
Here’s how to create and use CComPtr
and CComQIPtr
instances in C++:
1. Include the ATL headers:
#include <atlbase.h>
2. Create a CComPtr
instance:
CComPtr<IUnknown> spUnknown; // Example interface type (replace with your interface)
3. Initialize the CComPtr
with a COM object:
HRESULT hr = CoCreateInstance(CLSID_YourCOMObject, NULL, CLSCTX_ALL, IID_IUnknown, (void**)&spUnknown);
if (SUCCEEDED(hr)) {
// The COM object was created successfully
}
4. Use the CComPtr
like a regular pointer:
if (spUnknown) {
// The pointer is valid, you can use it
}
5. Automatically release the COM object when the CComPtr
goes out of scope:
You don’t need to explicitly release the COM object; the CComPtr
will take care of it when it’s destructed.
Here’s how to use CComQIPtr
for Query Interface (QI) operations:
1. Create a CComQIPtr
instance:
CComQIPtr<IMyInterface> spMyInterface;
2. Initialize the CComQIPtr
by querying the interface from another COM object:
HRESULT hr = spUnknown->QueryInterface(IID_IMyInterface, (void**)&spMyInterface);
if (SUCCEEDED(hr)) {
// The QI operation succeeded, and you can now use spMyInterface
}
3. Use the CComQIPtr
like a regular pointer:
if (spMyInterface) {
// The pointer is valid, you can use it
}
4. Automatically release the COM object when the CComQIPtr
goes out of scope:
As with CComPtr
, you don’t need to explicitly release the COM object; the CComQIPtr
will handle it.
CComPtr
and CComQIPtr
help ensure proper resource management when working with COM interfaces in C++, making it easier to write robust and leak-free code.