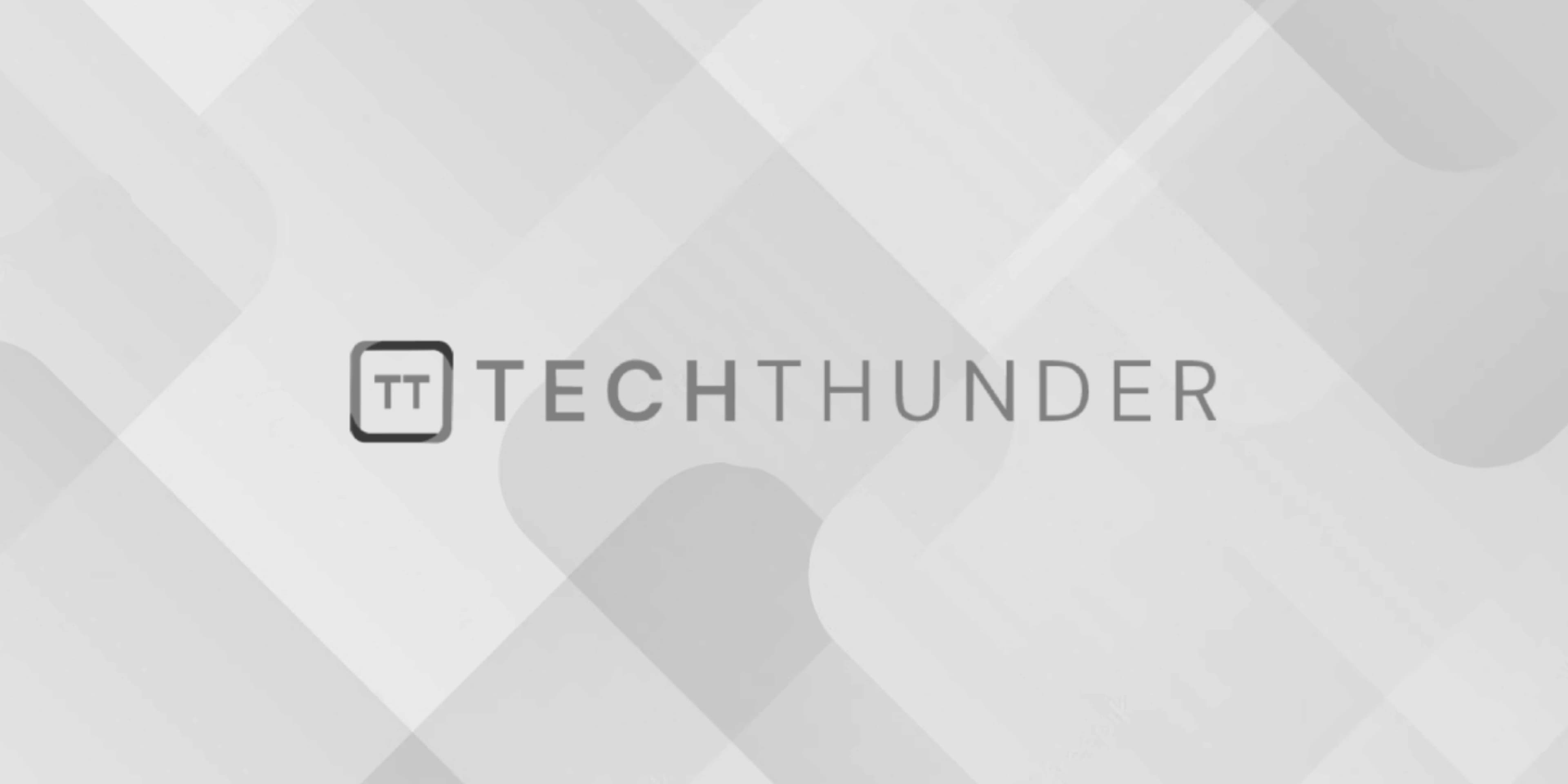
Inheritance in C++ vs JAVA
Inheritance is a fundamental object-oriented programming (OOP) concept that allows you to create a new class (derived or subclass) from an existing class (base or superclass). Both C++ and Java support inheritance, but there are some differences in how it is implemented and certain features related to inheritance. Here’s a comparison of inheritance in C++ and Java:
1. Syntax for Inheritance:
- C++: In C++, inheritance is declared using the
class
orstruct
keyword, followed by a colon:
and the access specifier (e.g.,public
,private
,protected
) and the base class name. Multiple inheritance is allowed in C++, meaning a class can inherit from multiple base classes.
class Base {
// Base class members
};
class Derived : public Base {
// Derived class members
};
- Java: In Java, inheritance is declared using the
extends
keyword, and Java supports single inheritance only (a class can extend only one class). However, a class can implement multiple interfaces.
class Base {
// Base class members
}
class Derived extends Base {
// Derived class members
}
2. Access Modifiers:
- C++: In C++, access control specifiers (e.g.,
public
,private
,protected
) control the visibility of base class members in the derived class. The default access specifier isprivate
for class members if not specified otherwise. - Java: In Java, base class members with access modifier
public
orprotected
are inherited by the derived class, whileprivate
members are not inherited. Java does not have explicit access specifiers likeprivate
andprotected
for class itself (the class itself is always package-private).
3. Constructor Invocation:
- C++: In C++, the derived class constructor can explicitly call the base class constructor using the base class’s name in the member initializer list.
Derived() : Base() {
// Derived class constructor
}
- Java: In Java, the base class constructor is implicitly called when a derived class object is created. Java enforces this rule, and you cannot explicitly call a base class constructor from a derived class constructor.
4. Method Overriding:
- C++: In C++, you can override base class methods in the derived class by using the
virtual
keyword in the base class andoverride
keyword in the derived class (C++11 and later).
class Base {
virtual void foo() {
/* Base class implementation */
}
};
class Derived : public Base {
void foo()
override {
/* Derived class implementation */
}
};
- Java: In Java, methods in a derived class are automatically considered to override the same-named methods in the base class. You can use the
@Override
annotation to indicate explicit intent.
class Base {
void foo() {
/* Base class implementation */
}
}
class Derived extends Base {
@Override void foo() {
/* Derived class implementation */
}
}
5. Multiple Inheritance:
- C++: C++ supports multiple inheritance, allowing a class to inherit from multiple base classes. This can lead to issues like the diamond problem, which C++ addresses through virtual inheritance.
- Java: Java supports single inheritance of classes but allows multiple inheritance of interfaces through the
implements
keyword. This avoids the diamond problem, as interfaces do not contain implementation details.
In summary, both C++ and Java support inheritance, but they have differences in syntax, access control, constructor invocation, and handling of method overriding and multiple inheritance. These differences are influenced by the design philosophies of each language and their goals for simplicity, safety, and maintainability.