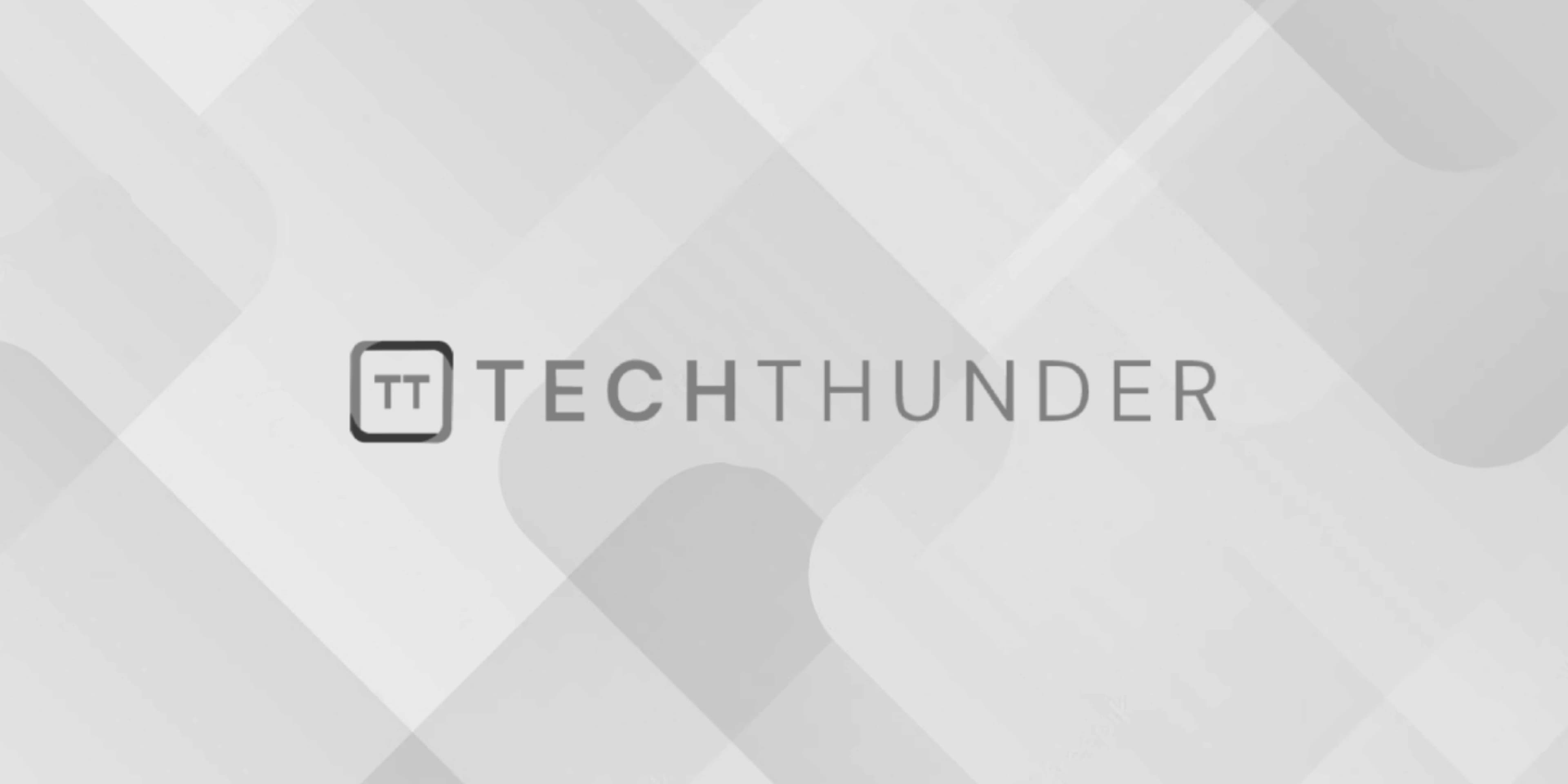
C++ Destructor
The C++ a destructor is a special member function of a class that is used to clean up resources and perform any necessary cleanup when an object of that class is destroyed or goes out of scope. The destructor has the same name as the class, preceded by a tilde (~
). Destructors are called automatically when objects are destroyed, and they are mainly used to release resources such as memory allocated with new
, close files, or perform any other cleanup operations.
Here’s the basic syntax of a destructor in C++:
class MyClass {
public:
// Constructor
MyClass() {
// Constructor code
}
// Destructor
~MyClass() {
// Destructor code (cleanup)
}
};
Key points about C++ destructors:
- Destructor Syntax: The destructor’s name is the same as the class name, but preceded by a tilde (
~
). It does not take any parameters and has no return type (not evenvoid
). - Automatic Invocation: Destructors are called automatically when an object of the class is destroyed, either when it goes out of scope (e.g., at the end of a function) or when
delete
is used to deallocate memory for dynamically allocated objects. - Resource Cleanup: Destructors are often used to release resources like memory, close open files, or perform other cleanup operations. For example, if a class allocates memory using
new
, it should deallocate that memory in its destructor to prevent memory leaks.
class DynamicMemoryClass {
private:
int* dynamicArray;
public:
DynamicMemoryClass() {
dynamicArray = new int[10];
}
~DynamicMemoryClass() {
delete[] dynamicArray; // Cleanup: deallocate dynamic memory
}
};
- Multiple Destructors: In C++, you cannot define multiple destructors with different parameters or overloads. Each class has only one destructor, and it is automatically called when an object of that class is destroyed.
- Order of Destructor Calls: If an object of a derived class is destroyed, the destructor of the base class is called first, followed by the destructor of the derived class. This ensures that resources are properly released in the correct order in class hierarchies.
- Destructor Inheritance: Derived classes inherit the destructor of the base class, but they can also define their own destructor if needed. When the derived class destructor is called, it automatically calls the base class destructor.
class Base {
public:
~Base() {
// Base class destructor code
}
};
class Derived : public Base {
public:
~Derived() {
// Derived class destructor code
}
};
- No Explicit Invocation: You should not explicitly call a destructor like a regular member function. Destructors are automatically called when an object’s lifetime ends. Calling a destructor explicitly can lead to undefined behavior.
Destructors are essential for proper resource management in C++ classes, especially when dealing with dynamically allocated memory and other external resources. They help ensure that resources are released and cleaned up correctly when objects are no longer needed.