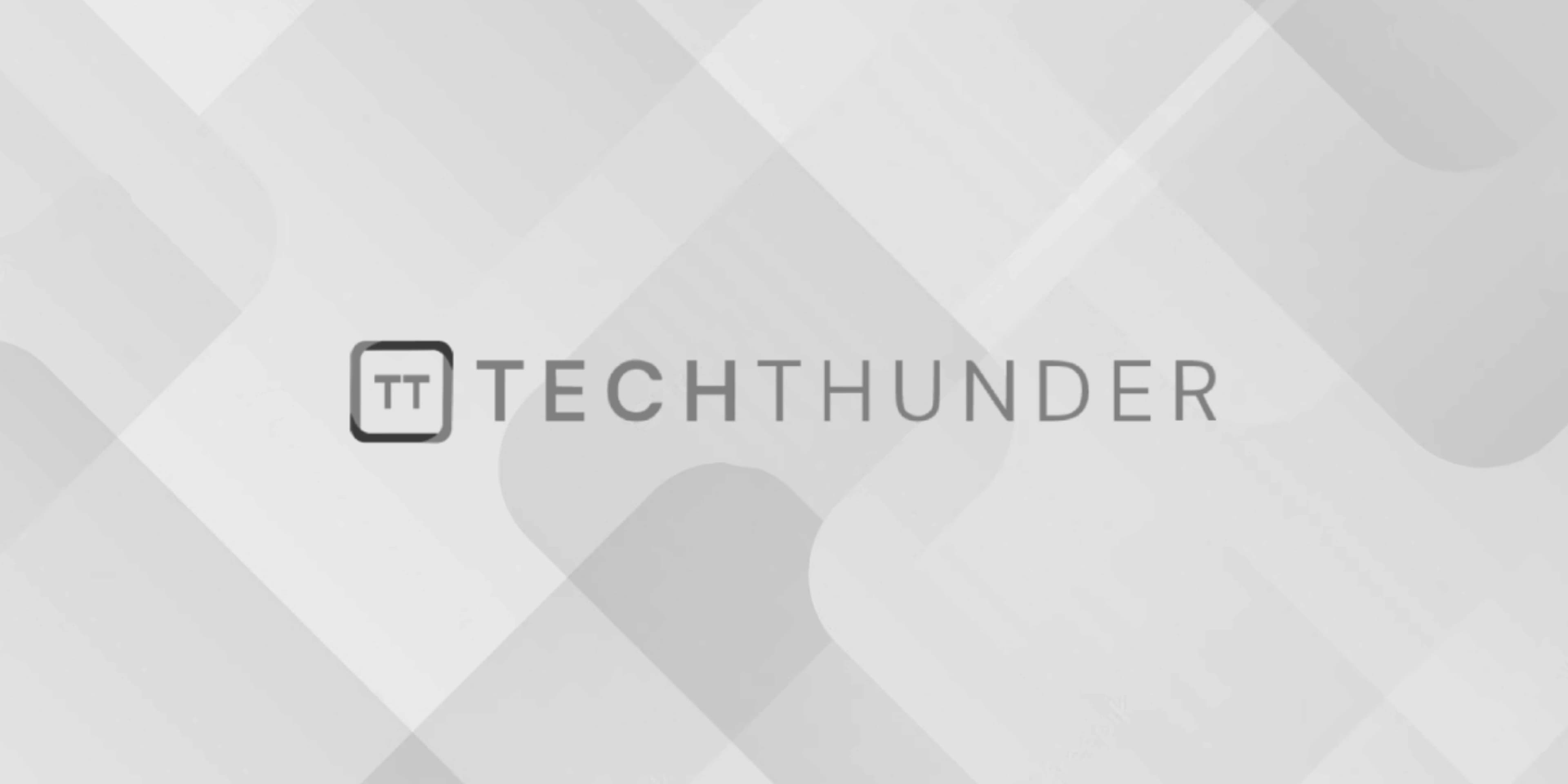
Hybrid inheritance in C++
Hybrid inheritance in C++ refers to a combination of multiple types of inheritance within a single class hierarchy. It involves the use of multiple inheritance and can include hierarchical, multiple, or multilevel inheritance within the same hierarchy. Hybrid inheritance allows you to create complex class relationships by combining various forms of inheritance to meet your program’s requirements.
Here’s an example of hybrid inheritance:
#include <iostream>
// Base class 1
class Animal {
public:
void eat() {
std::cout << "Animal is eating." << std::endl;
}
};
// Base class 2
class Vehicle {
public:
void drive() {
std::cout << "Vehicle is driving." << std::endl;
}
};
// Derived class 1 with hierarchical inheritance
class Dog : public Animal {
public:
void bark() {
std::cout << "Dog is barking." << std::endl;
}
};
// Derived class 2 with multiple inheritance
class Car : public Vehicle, public Animal {
public:
void honk() {
std::cout << "Car is honking." << std::endl;
}
};
int main() {
Dog dog;
Car car;
dog.eat(); // Inherited from Animal
dog.bark(); // Unique to Dog
car.drive(); // Inherited from Vehicle
car.eat(); // Inherited from Animal
car.honk(); // Unique to Car
return 0;
}
In this example, we have two base classes (Animal
and Vehicle
) and two derived classes (Dog
and Car
). Dog
uses hierarchical inheritance from the Animal
base class, inheriting the eat
method. Car
uses multiple inheritance, inheriting from both Vehicle
and Animal
, which means it inherits both drive
and eat
methods. Additionally, Car
has its own unique method, honk
.
Hybrid inheritance allows you to build complex class relationships and reuse code effectively, but it can also lead to potential ambiguity issues or the diamond problem when multiple inheritance is involved. To avoid such issues, you can use virtual inheritance to resolve ambiguities in cases of multiple inheritance within a hybrid inheritance hierarchy.