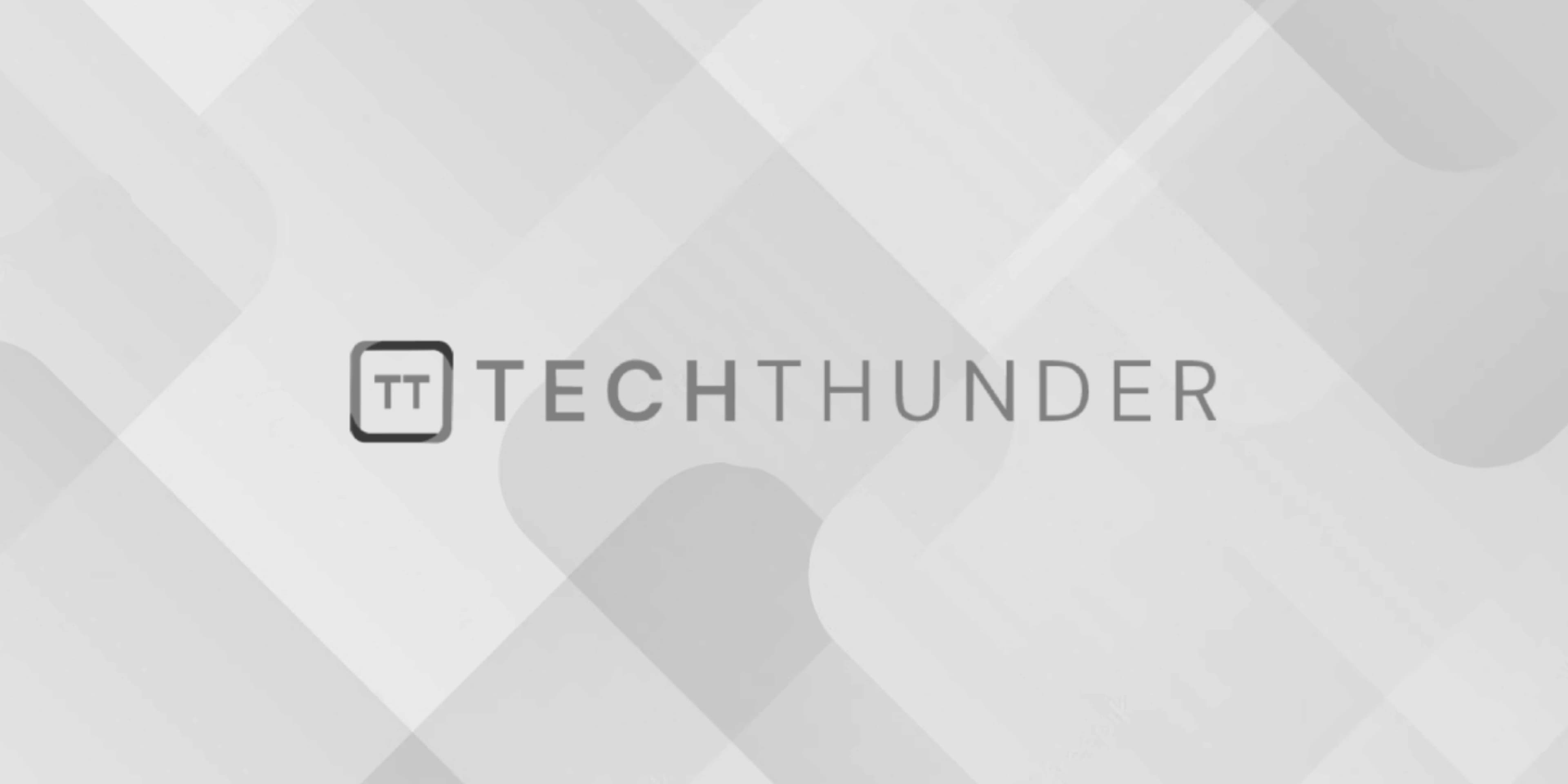
136 views
Maximum Number of Edges to be Added to a Tree so that it stays a Bipartite Graph in C++
To convert a tree into a bipartite graph, you can add at most n-1
edges, where n
is the number of nodes in the tree. This can be achieved by adding edges between nodes of the same level.
Here is a C++ program to illustrate this:
C++
#include <iostream>
#include <vector>
using namespace std;
const int MAXN = 100005;
vector<int> adj[MAXN];
vector<bool> visited(MAXN, false);
vector<int> level(MAXN, 0);
vector<int> parent(MAXN, -1);
void dfs(int node, int lvl) {
visited[node] = true;
level[node] = lvl;
for (int child : adj[node]) {
if (!visited[child]) {
parent[child] = node;
dfs(child, lvl + 1);
}
}
}
int main() {
int n;
cin >> n;
// Assuming the tree is given as n-1 edges
for (int i = 0; i < n - 1; i++) {
int u, v;
cin >> u >> v;
adj[u].push_back(v);
adj[v].push_back(u);
}
dfs(1, 0);
// Print the edges to be added to make it bipartite
cout << "Edges to be added to make it Bipartite:" << endl;
for (int i = 2; i <= n; i++) {
if (level[i] % 2 == level[parent[i]] % 2) {
cout << i << " " << parent[i] << endl;
}
}
return 0;
}
Explanation:
- We perform a depth-first search (DFS) on the tree, assigning levels to each node.
- The nodes at even levels and odd levels will be in different partitions (bipartite property).
- For each node, if its level is the same as its parent’s level, we print an edge between them.
Keep in mind that this program assumes the input tree is given as n-1
edges in the format of pairs (u, v)
representing an edge between nodes u
and v
. Modify the input reading part according to your specific input format if needed.